Simplifying JavaScript Development with Function Libraries
In the fast-paced world of web development, JavaScript remains an essential language for creating dynamic and interactive websites. While JavaScript offers a wide array of built-in functions and methods, harnessing its full potential often requires writing extensive code. This is where function libraries come to the rescue. Function libraries are collections of pre-written JavaScript functions that can be easily integrated into your projects, simplifying development, enhancing productivity, and promoting code reusability.
Table of Contents
1. The Power of Function Libraries
At its core, JavaScript function libraries provide developers with a set of tools to perform common tasks without having to reinvent the wheel each time. These libraries encapsulate complex operations into simple function calls, reducing the amount of code you need to write. This not only speeds up the development process but also leads to more maintainable code in the long run.
2. Advantages of Using Function Libraries
- Code Efficiency: Function libraries offer a wide range of pre-built functions that are optimized for performance and accuracy. Instead of spending time coding repetitive tasks, you can leverage these functions to achieve your goals swiftly.
javascript // Without Function Library let sum = 0; for (let i = 0; i < numbers.length; i++) { sum += numbers[i]; } // With Function Library let total = library.sum(numbers);
- Consistency: Function libraries often follow best practices and coding standards, ensuring a consistent coding style across your project. This consistency is particularly valuable when working in a team or collaborating with other developers.
- Cross-Browser Compatibility: Dealing with browser inconsistencies is a common challenge in JavaScript development. Many function libraries abstract away these differences, allowing you to write code that works seamlessly across various browsers.
- Enhanced Productivity: By using function libraries, you can save significant time during the development process. These libraries provide well-documented APIs and examples, reducing the learning curve and enabling you to focus on solving specific problems.
- Bug Reduction: Function libraries are often developed, tested, and maintained by a community of experienced developers. This collective effort helps identify and fix bugs quickly, ensuring that your codebase remains stable.
3. Popular JavaScript Function Libraries
Several function libraries have gained widespread popularity in the JavaScript community due to their versatility and robustness. Let’s explore some of these libraries and how they can simplify your development workflow:
3.1. Lodash
Lodash is a widely used utility library that provides a plethora of functions to manipulate arrays, objects, strings, and more. It simplifies complex operations and enhances code readability.
Example: Removing duplicates from an array
javascript // Without Lodash const uniqueArray = []; for (let i = 0; i < inputArray.length; i++) { if (!uniqueArray.includes(inputArray[i])) { uniqueArray.push(inputArray[i]); } } // With Lodash const uniqueArray = _.uniq(inputArray);
3.2. jQuery
jQuery revolutionized the way developers interact with the DOM and handle asynchronous operations. Although modern JavaScript has improved in these areas, jQuery’s concise syntax and browser compatibility still make it a valuable library.
Example: Making an AJAX request
javascript // Without jQuery fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Handle data }) .catch(error => { // Handle error }); // With jQuery $.ajax({ url: 'https://api.example.com/data', success: function(data) { // Handle data }, error: function(error) { // Handle error } });
3.3. Moment.js
For handling dates and times, Moment.js offers a comprehensive set of functions that simplify parsing, formatting, and manipulating dates.
Example: Formatting a date
javascript // Without Moment.js const date = new Date(); const formattedDate = `${date.getFullYear()}-${date.getMonth() + 1}-${date.getDate()}`; // With Moment.js const formattedDate = moment().format('YYYY-MM-DD');
3.4. Axios
Axios is a promise-based HTTP client that simplifies making HTTP requests and handling responses. It supports various features like request cancellation, interceptors, and automatic conversion of JSON data.
Example: Making a GET request
javascript // Without Axios fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Handle data }) .catch(error => { // Handle error }); // With Axios axios.get('https://api.example.com/data') .then(response => { // Handle data }) .catch(error => { // Handle error });
4. Integrating Function Libraries into Your Project
Integrating a function library into your project is usually straightforward. Most libraries can be added via package managers like npm or yarn, making installation a breeze. Once installed, you can import the desired functions and start using them in your code.
4.1. Installation
bash # Using npm npm install lodash # Using yarn yarn add lodash
4.2. Importing and Using Functions
javascript // Importing Lodash functions import { uniq, sortBy, filter } from 'lodash'; const numbers = [3, 1, 2, 3, 1, 4, 5, 2, 6]; const uniqueNumbers = uniq(numbers); const sortedNumbers = sortBy(numbers); const filteredNumbers = filter(numbers, num => num > 2);
Conclusion
Function libraries play a pivotal role in simplifying JavaScript development. They enable developers to write less code, enhance productivity, and maintain a consistent coding style. By leveraging the power of popular libraries like Lodash, jQuery, Moment.js, and Axios, you can streamline your development workflow and focus on building exceptional web applications. Whether you’re a beginner or an experienced developer, integrating function libraries into your projects can undoubtedly make your coding journey smoother and more efficient. So why not explore these libraries and take your JavaScript skills to the next level?
Incorporating function libraries is a smart strategy for any JavaScript developer looking to expedite their projects while maintaining code quality. These libraries offer a treasure trove of functions that simplify complex tasks, provide solutions to common challenges, and contribute to a more seamless and enjoyable development experience. So, why not embrace the power of function libraries and unlock your full JavaScript potential? Your future self will thank you.
Table of Contents
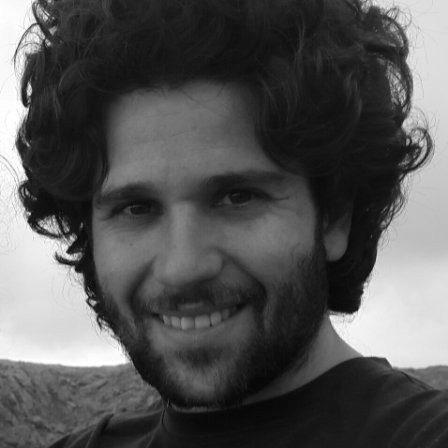
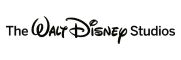