Simplifying API Consumption with JavaScript Fetch Functions
In today’s fast-paced world of web development, consuming data from APIs is a common task. Whether you’re building a dynamic web application, fetching data for a mobile app, or integrating third-party services, a streamlined approach to API consumption is crucial. JavaScript’s fetch() function has become a game-changer in this realm, providing a modern and simplified way to make network requests and handle responses. In this blog post, we’ll dive deep into the world of fetch() and learn how to simplify API consumption using this powerful function.
Table of Contents
1. Understanding the Traditional Approach
Before we delve into the wonders of fetch(), let’s briefly touch on the traditional approach to making API requests in JavaScript. In the past, developers often relied on the XMLHttpRequest object, which allowed them to send HTTP requests and handle responses. However, this approach had its complexities and quirks, making the code less readable and harder to maintain. With the advent of modern web standards, the fetch() function emerged as a more elegant solution.
2. The Power of the Fetch Function
The fetch() function is built into modern browsers and provides a straightforward way to initiate network requests. It returns a Promise that resolves to the Response to that request, whether it’s successful or not. The beauty of fetch() lies in its simplicity and consistency, making it an ideal choice for consuming APIs.
3. Making a Basic GET Request
Let’s start with a simple example of how to use the fetch() function to make a GET request to an API:
javascript fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { console.log(data); }) .catch(error => { console.error('Error:', error); });
In this example, we call the fetch() function with the URL of the API endpoint we want to retrieve data from. The returned Promise is then handled using .then() to extract the JSON response using the json() method. Finally, we log the data to the console. If there’s an error during the process, it’s caught using .catch().
4. Handling Different HTTP Methods
The simplicity of fetch() doesn’t stop at just making GET requests. It can be used for various HTTP methods like POST, PUT, DELETE, and more. Let’s see how to make a POST request with data:
javascript const postData = { title: 'New Post', content: 'This is some content for the new post.' }; fetch('https://api.example.com/posts', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(postData) }) .then(response => response.json()) .then(data => { console.log('New post created:', data); }) .catch(error => { console.error('Error:', error); });
In this example, we specify the HTTP method as ‘POST’ in the fetch() options. We also set the ‘Content-Type’ header to indicate that we’re sending JSON data. The data is converted to a JSON string using JSON.stringify() and sent in the request body.
5. Simplifying Error Handling
Error handling is an important aspect of any network request. With fetch(), handling errors becomes straightforward:
javascript fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => { console.log(data); }) .catch(error => { console.error('Fetch error:', error); });
In this example, we use the ok property of the response to check if the request was successful. If not, we throw an error. This error is then caught in the .catch() block, making error handling clean and intuitive.
6. Dealing with Asynchronous Operations
Asynchronous operations are at the heart of network requests, and fetch() seamlessly integrates with JavaScript’s Promise-based asynchronous programming. This makes it easy to manage multiple requests and responses without blocking the main thread.
7. Streamlining Code with Async/Await
While the .then() syntax is effective, JavaScript’s async/await syntax offers an even cleaner way to handle asynchronous operations:
javascript async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Async error:', error); } } fetchData();
By using async and await, the code flows in a more sequential manner, making it easier to read and understand. The try...catch block provides a centralized location for error handling. Customizing Headers and Authentication API requests often require custom headers or authentication tokens. With fetch(), adding headers is a breeze:
javascript const headers = new Headers({ 'Authorization': 'Bearer YOUR_ACCESS_TOKEN', 'Custom-Header': 'Custom-Value' }); fetch('https://api.example.com/data', { headers: headers }) .then(response => response.json()) .then(data => { console.log(data); }) .catch(error => { console.error('Error:', error); });
Here, we create a Headers object and add the necessary headers to it. Then, we pass the headers object in the fetch() options.
Conclusion
In the world of modern web development, simplifying processes without compromising functionality is a constant goal. JavaScript’s fetch() function exemplifies this philosophy by offering a clean and concise way to consume APIs. Its Promise-based nature, combined with async/await syntax, makes handling asynchronous operations a breeze. Whether you’re making simple GET requests or more complex operations involving different HTTP methods and custom headers, fetch() streamlines the process.
As you embark on your journey to build dynamic web applications, remember the power of fetch(). With its user-friendly approach, it’s an indispensable tool for simplifying API consumption and enhancing your development workflow. So, go ahead and embrace the elegance of fetch() to unlock a new level of efficiency in your projects. Happy coding!
Table of Contents
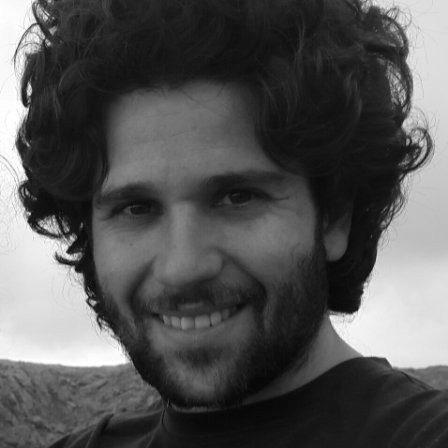
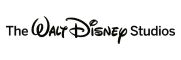