Simplifying Asynchronous Programming with JavaScript Async Functions
Asynchronous programming lies at the heart of modern web development, enabling applications to handle tasks like data fetching, file I/O, and network requests without freezing up the user interface. JavaScript, as a language, has evolved to address these challenges with various asynchronous patterns and APIs. One of the most significant advancements in this realm is the introduction of async functions, a feature that simplifies and enhances the readability of asynchronous code. In this article, we will delve into the world of JavaScript async functions, exploring how they work, their benefits, and how to use them effectively.
Table of Contents
1. Understanding Asynchronous JavaScript
Before diving into async functions, let’s quickly recap why asynchronous programming is crucial in JavaScript. JavaScript is single-threaded, meaning it executes one piece of code at a time. This can lead to performance bottlenecks when handling time-consuming operations, like fetching data from a server or reading files. To prevent the entire program from freezing while waiting for these operations to complete, JavaScript provides asynchronous mechanisms.
1.1. The Callback Hell Conundrum
Traditionally, JavaScript used callbacks to manage asynchronous operations. However, this approach often led to what’s colloquially known as “Callback Hell.” Nesting multiple callbacks within callbacks could quickly turn code into an unreadable and hard-to-maintain mess. Here’s an example of what it might look like:
javascript getData(function(data) { getMoreData(data, function(moreData) { getEvenMoreData(moreData, function(evenMoreData) { // And it goes on... }); }); });
1.2. Introducing Promises
To alleviate the callback problem, Promises were introduced. Promises provide a cleaner way to handle asynchronous operations, allowing you to chain then() methods. While Promises were a significant improvement, they still required handling some boilerplate code and managing the flow using .then() and .catch().
javascript getData() .then(data => getMoreData(data)) .then(moreData => getEvenMoreData(moreData)) .then(evenMoreData => { // Handle evenMoreData }) .catch(error => { // Handle errors });
2. Enter Async Functions
Async functions take asynchronous programming in JavaScript to the next level by making it look and feel like synchronous code. They are built on top of Promises and provide a cleaner and more intuitive syntax for managing asynchronous operations. Let’s explore how async functions work and the benefits they offer.
2.1. The Anatomy of an Async Function
An async function is declared using the async keyword before the function keyword in a function declaration or expression. Inside an async function, you can use the await keyword to pause the execution of the function until a Promise is resolved.
Here’s a basic structure of an async function:
javascript async function fetchData() { // Asynchronous operations using await const data = await getData(); const moreData = await getMoreData(data); return moreData; }
In this example, the execution of fetchData() will pause at each await expression until the corresponding Promise resolves. This allows you to write asynchronous code in a more linear and readable manner.
2.2. Error Handling with Async Functions
Error handling in async functions is straightforward and closely resembles traditional try-catch blocks. You can wrap your await statements in a try block and catch any errors that occur during the asynchronous operations.
javascript async function fetchDataWithErrors() { try { const data = await getData(); // ... } catch (error) { // Handle errors } }
3. Benefits of Async Functions
Async functions offer several benefits that simplify asynchronous programming in JavaScript:
3.1. Improved Readability
By removing the need for nested callbacks or long chains of .then() calls, async functions make your code easier to read and maintain.
3.2. Synchronous-Like Syntax
Async functions make asynchronous code look and behave more like synchronous code, reducing cognitive load and making it easier for developers to reason about the program flow.
3.3. Error Propagation
Error handling becomes more intuitive and centralized with try-catch blocks, making it easier to manage and troubleshoot errors.
3.4. Modularization
Async functions encourage breaking down complex asynchronous operations into smaller, more manageable functions, promoting code reusability.
4. Using Async Functions in Real-World Scenarios
Let’s walk through a real-world scenario where async functions shine: fetching data from an API and rendering it on a web page.
javascript async function fetchAndRenderData() { try { const data = await fetchDataFromAPI(); renderData(data); } catch (error) { showError(error); } } fetchAndRenderData();
In this example, the fetchAndRenderData() function encapsulates the process of fetching data from an API and rendering it on the web page. Any errors that occur during these steps are caught and handled within the function.
Conclusion
JavaScript async functions provide an elegant solution to the challenges of asynchronous programming. By combining the power of Promises with a synchronous-like syntax, async functions make your code more readable, maintainable, and error-resistant. As modern web development continues to evolve, mastering asynchronous programming with async functions is a skill that every JavaScript developer should possess. So go ahead, simplify your asynchronous JavaScript code and embrace the world of async functions.
Asynchronous programming is a crucial aspect of modern web development, enabling applications to perform tasks like data fetching, file I/O, and network requests without blocking the user interface. However, dealing with asynchronous operations using traditional callback-based or promise-based approaches can often lead to complex and hard-to-maintain code. This is where JavaScript async functions come to the rescue. In this article, we’ll explore how async functions can simplify asynchronous programming in JavaScript and provide cleaner, more readable code.
Understanding Asynchronous JavaScript
Before we dive into async functions, let’s briefly review why asynchronous programming is essential in JavaScript. JavaScript is single-threaded, meaning it can only execute one task at a time. This can create performance issues when dealing with time-consuming operations like network requests or file reading. To avoid blocking the execution and freezing the user interface, JavaScript offers asynchronous mechanisms.
The Callback Hell
In the past, callbacks were the primary means of managing asynchronous operations in JavaScript. However, using callbacks for complex sequences of asynchronous tasks often led to a phenomenon known as “Callback Hell.” This involved deeply nested and indented callback functions, making the code difficult to read, debug, and maintain.
javascript getData(function(data) { getMoreData(data, function(moreData) { getEvenMoreData(moreData, function(evenMoreData) { // ... }); }); });
Introducing Promises
To address the issues associated with callbacks, JavaScript introduced Promises. Promises offer a more structured way to handle asynchronous operations, allowing you to chain them together using then() and catch() methods. While Promises improved the situation, they still required managing multiple layers of callbacks.
javascript getData() .then(data => getMoreData(data)) .then(moreData => getEvenMoreData(moreData)) .then(evenMoreData => { // ... }) .catch(error => { // Handle errors });
Enter Async Functions
Async functions build upon the concept of Promises and provide a more elegant solution to writing asynchronous code. They make asynchronous code appear and behave more like synchronous code, improving its readability and maintainability. Let’s dive into how async functions work and the benefits they offer.
Anatomy of an Async Function
An async function is defined using the async keyword before the function keyword in a function declaration or expression. Inside an async function, you can use the await keyword to pause the execution until a Promise is resolved.
javascript async function fetchData() { const data = await getData(); const moreData = await getMoreData(data); return moreData; }
In this example, the execution of the fetchData() function will pause at each await expression until the corresponding Promise resolves. This linear flow makes the code easier to follow compared to nested callbacks or chained Promises.
Error Handling with Async Functions
Error handling in async functions is similar to traditional try-catch blocks. You can wrap await statements in a try block and catch errors that occur during the asynchronous operations.
javascript async function fetchDataWithErrors() { try { const data = await getData(); // ... } catch (error) { // Handle errors } }
Benefits of Async Functions
Async functions offer several advantages that simplify asynchronous programming:
1. Improved Readability
By eliminating nested callbacks and long promise chains, async functions make the code more readable and easier to understand.
2. Synchronous-Like Syntax
Async functions provide a more intuitive and synchronous-like syntax, making it easier to reason about the program’s flow.
3. Error Propagation
Error handling becomes straightforward with try-catch blocks, centralizing error management and making debugging simpler.
4. Modularization
Async functions encourage breaking down complex asynchronous operations into smaller, reusable functions, enhancing code modularity.
Using Async Functions in Real-World Scenarios
Let’s walk through a real-world example to showcase how async functions can be used effectively: fetching data from an API and displaying it on a web page.
javascript async function fetchAndDisplayData() { try { const data = await fetchDataFromAPI(); displayData(data); } catch (error) { showError(error); } } fetchAndDisplayData();
In this scenario, the fetchAndDisplayData() function encapsulates the process of fetching data from an API and rendering it on a web page. Any errors that occur during these steps are caught and handled within the function.
Conclusion
Async functions have revolutionized the way we write asynchronous JavaScript code. By combining the power of Promises with a more synchronous-like syntax, async functions offer cleaner, more readable, and more maintainable code. As the world of web development continues to evolve, mastering asynchronous programming using async functions is a crucial skill for every JavaScript developer. So go ahead, simplify your asynchronous JavaScript code using async functions and embrace a more elegant way of handling asynchronous operations. Your codebase and your fellow developers will thank you for it.
Table of Contents
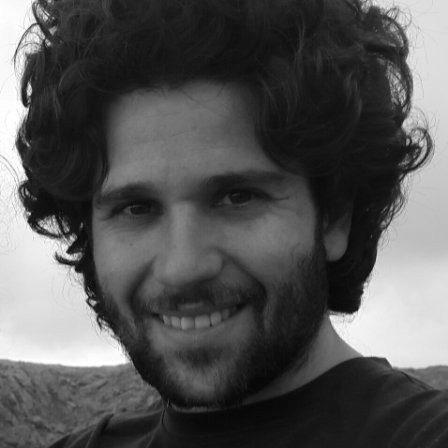
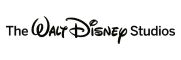