Mastering JavaScript Immediately Executing Arrow Functions (IIFE + Arrow)
In the world of JavaScript, mastering various techniques can significantly enhance your coding prowess. One such powerful technique is the use of Immediately Executing Arrow Functions, commonly known as IIFE + Arrow. These compact and efficient functions combine the advantages of Arrow Functions and Immediately Invoked Function Expressions (IIFE) to create concise, self-invoking code blocks. In this article, we’ll delve into the intricacies of IIFE and Arrow Functions and explore how combining them can streamline your code and lead to cleaner, more readable JavaScript.
Table of Contents
1. Understanding Arrow Functions
1.1. Introduction to Arrow Functions
Arrow Functions, introduced in ECMAScript 6 (ES6), provide a concise syntax for writing function expressions. They offer a shorter and more readable way to define functions compared to traditional function expressions. The syntax of Arrow Functions omits the need for the function keyword and curly braces when there’s only a single expression to be returned.
Here’s a quick example of a traditional function expression and its equivalent Arrow Function:
javascript // Traditional function expression const multiply = function(a, b) { return a * b; }; // Arrow Function equivalent const multiply = (a, b) => a * b;
1.2. Advantages of Arrow Functions
Arrow Functions come with several advantages that contribute to cleaner and more efficient code:
- Conciseness: Arrow Functions eliminate the need for explicit return statements when there’s a single expression in the function body. This leads to shorter code.
- Lexical this: In traditional functions, the value of this depends on how the function is called. Arrow Functions, however, maintain the value of this from the surrounding code, making it easier to manage scope and context.
- Implicit return: When using Arrow Functions, the return value of the function is implicitly determined by the expression within the function body, further reducing the need for explicit return statements.
2. Exploring Immediately Invoked Function Expressions (IIFE)
2.1. What is an IIFE?
An Immediately Invoked Function Expression (IIFE) is a JavaScript function that is executed immediately after it’s defined. It’s often used to create a new scope for variables, encapsulating them and preventing them from polluting the global scope. The primary syntax of an IIFE involves wrapping the function in parentheses and then immediately invoking it by adding () at the end.
Here’s a basic example of an IIFE:
javascript (function() { // IIFE code here })();
2.2. Benefits of Using IIFE
IIFE offers several benefits, including:
- Encapsulation: By creating a new scope, IIFE helps prevent variable name clashes and unintended global variable declarations. This is crucial for maintaining clean and organized code.
- Pollution Prevention: IIFE ensures that any variables declared within the function are not accessible from the outside scope, reducing the chances of unintended variable modifications.
- Dependency Management: IIFE can be used to manage dependencies by passing them as arguments to the function. This enables better control over which variables are accessible within the function.
3. Merging Powers: IIFE + Arrow
3.1. Syntax of IIFE + Arrow
The fusion of Immediately Invoked Function Expressions and Arrow Functions results in a potent combination that enhances code readability and maintainability. The syntax involves wrapping an Arrow Function in parentheses and then immediately invoking it.
Here’s how an IIFE + Arrow looks:
javascript (() => { // IIFE + Arrow code here })();
The advantage of using this combination is that you can leverage the concise syntax of Arrow Functions while benefiting from the encapsulation and scope isolation provided by IIFE.
4. Use Cases and Examples
4.1. Data Encapsulation
Consider a scenario where you want to create a counter that’s encapsulated within its own scope. Using IIFE + Arrow, you can achieve this easily:
javascript const counter = (() => { let count = 0; return { increment: () => { count++; }, getCount: () => { return count; } }; })(); counter.increment(); console.log(counter.getCount()); // Output: 1
4.2. Event Handling
IIFE + Arrow is also useful when dealing with event handlers. Let’s say you want to attach an event listener to a button:
javascript const button = document.getElementById('myButton'); (() => { button.addEventListener('click', () => { console.log('Button clicked!'); }); })();
4.3. Module Initialization
When creating modular JavaScript applications, you can utilize IIFE + Arrow to initialize modules with private and public methods:
javascript const myModule = (() => { const privateData = 'I am private'; const getPrivateData = () => { return privateData; }; const publicMethod = () => { console.log('Public method'); }; return { publicMethod, getPrivateData }; })(); myModule.publicMethod(); console.log(myModule.getPrivateData()); // Output: "I am private"
5. Mastering IIFE + Arrow: Best Practices
5.1. Maintaining Readability
While IIFE + Arrow provides a concise way to structure your code, it’s important to prioritize readability. Avoid writing overly complex logic within the IIFE + Arrow to prevent sacrificing clarity for brevity.
5.2. Handling Dependencies
When working with dependencies, consider passing them as arguments to the IIFE + Arrow instead of relying on the surrounding scope. This ensures that the IIFE + Arrow is self-contained and can be reused without unexpected side effects.
5.3. Future of JavaScript: ECMAScript Modules
As JavaScript continues to evolve, the use of ECMAScript Modules (ESM) is becoming more prominent. ESM offers a standardized way to work with modules, enabling you to easily share code between files and projects. While IIFE + Arrow is a useful technique, embracing ES Modules can provide even better modularization and maintainability.
Conclusion
Mastering Immediately Executing Arrow Functions (IIFE + Arrow) can significantly enhance your JavaScript coding skills. By combining the advantages of Arrow Functions and IIFE, you can create clean, encapsulated, and efficient code. From data encapsulation to event handling and module initialization, the applications of IIFE + Arrow are diverse and powerful. Remember to prioritize readability and proper dependency management while using this technique. As JavaScript continues to evolve, exploring new features like ECMAScript Modules will also contribute to your growth as a developer. Happy coding!
Table of Contents
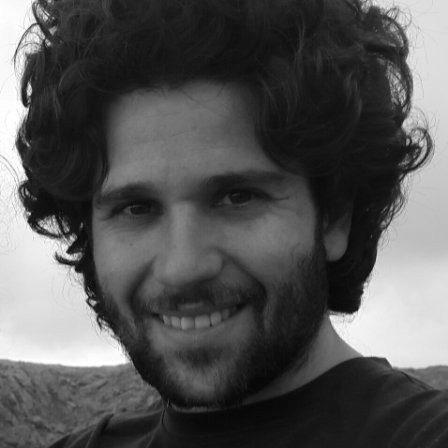
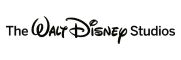