Simplifying JavaScript Form Validation with Helper Functions
As web developers, one of the most crucial aspects of creating user-friendly and efficient web applications is handling form validation. User input needs to be validated to ensure data accuracy and enhance the overall user experience. JavaScript, as a powerful scripting language, offers various ways to accomplish this task. In this blog post, we’ll explore how to simplify JavaScript form validation using helper functions. By the end, you’ll have a clear understanding of how these functions can streamline your code and lead to more maintainable forms.
Table of Contents
1. Why Helper Functions Matter
Before diving into the specifics of using helper functions for form validation, let’s understand the importance of these functions in the development process. Helper functions are reusable pieces of code designed to perform specific tasks. They encapsulate complex logic, making it easier to manage, test, and maintain your codebase. When it comes to form validation, helper functions can significantly reduce code duplication and improve the readability of your code.
2. The Common Challenges of Form Validation
Form validation might seem straightforward at first, but it can quickly become complex as you consider various scenarios and input types. Some common challenges include:
2.1. Handling Multiple Fields
Web forms often consist of multiple input fields that need validation. Writing individual validation logic for each field can lead to redundant code.
2.2. Ensuring Data Consistency
Validating user data involves ensuring consistency, such as confirming that passwords match or that email addresses are properly formatted.
2.3. Providing User Feedback
Displaying appropriate error messages when validation fails is essential for a good user experience. Manually managing error messages can be time-consuming and error-prone.
2.4. Adapting to Changing Requirements
As application requirements evolve, so does the validation logic. Maintaining and updating validation rules throughout your codebase can become a challenge.
3. Streamlining with Helper Functions
To overcome these challenges, let’s explore how helper functions can streamline your form validation process.
3.1. Creating Reusable Validation Functions
Instead of writing separate validation logic for each field, create reusable validation functions that can be applied to multiple fields. For example, you can create a function to validate email addresses:
javascript function isValidEmail(email) { const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; return emailPattern.test(email); }
Now you can use this function to validate email inputs across your application without duplicating code.
3.2. Handling Multiple Fields Effectively
Managing validation for multiple fields becomes simpler when you have helper functions in place. Consider a registration form with username, password, and confirm password fields. You can create a function to check if two fields have the same value:
javascript function areFieldsEqual(field1, field2) { return field1.value === field2.value; }
This function can be reused for comparing passwords, email confirmations, and more.
3.3. Providing Clear Error Messages
Helper functions also enable you to standardize error messages and make them more user-friendly. Create a function to display error messages consistently:
javascript function displayError(field, message) { const errorElement = field.nextElementSibling; errorElement.textContent = message; field.classList.add('error'); }
By calling this function whenever validation fails, you ensure that error messages are displayed consistently and styled appropriately.
3.4. Adapting to Changes Seamlessly
As requirements change, updating validation logic becomes easier with helper functions. Modifying a single function will reflect the changes across all instances of its use.
4. Putting It All Together: A Practical Example
Let’s put the concepts into action with a practical example. Imagine a login form with an email and password field. We want to ensure that both fields are filled out and that the email is in a valid format. Additionally, we want to display error messages if validation fails.
Here’s how we can use helper functions to achieve this:
javascript // Validation function for checking if a field is empty function isFieldEmpty(field) { return field.value.trim() === ''; } // Validation function for checking a valid email format function isValidEmail(email) { const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; return emailPattern.test(email); } // Display error message and highlight field function displayError(field, message) { const errorElement = field.nextElementSibling; errorElement.textContent = message; field.classList.add('error'); } // Clear error message and styling function clearError(field) { const errorElement = field.nextElementSibling; errorElement.textContent = ''; field.classList.remove('error'); } // Form submission handler function handleSubmit(event) { event.preventDefault(); const emailField = document.getElementById('email'); const passwordField = document.getElementById('password'); // Clear previous errors clearError(emailField); clearError(passwordField); // Validate email if (isFieldEmpty(emailField)) { displayError(emailField, 'Email cannot be empty'); } else if (!isValidEmail(emailField.value)) { displayError(emailField, 'Invalid email format'); } // Validate password if (isFieldEmpty(passwordField)) { displayError(passwordField, 'Password cannot be empty'); } } const loginForm = document.getElementById('login-form'); loginForm.addEventListener('submit', handleSubmit);
In this example, we’ve created specific helper functions for each aspect of form validation: checking if a field is empty, validating email format, and managing error messages. This modular approach enhances code readability and maintainability.
Conclusion
Streamlining JavaScript form validation with helper functions is a powerful technique that improves code organization, reduces duplication, and enhances the overall user experience. By encapsulating validation logic into reusable functions, you create a more manageable and adaptable codebase. Whether you’re working on simple login forms or complex multi-step wizards, using helper functions for form validation can simplify your development process and lead to more maintainable applications. So, the next time you’re faced with form validation challenges, remember the power of helper functions and apply them to create more elegant and efficient code.
Table of Contents
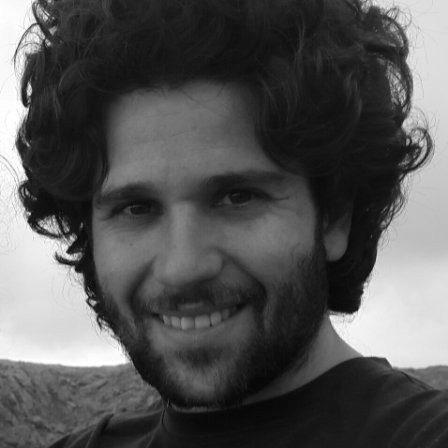
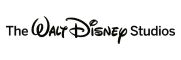