Mastering JavaScript Function Chaining for Streamlined Development
When it comes to writing efficient and elegant code in JavaScript, function chaining is a powerful technique that can significantly improve your development workflow. Function chaining allows you to perform multiple operations on the same object or result in a single, concise line of code, resulting in cleaner and more maintainable code. In this blog, we’ll explore what function chaining is, why it’s beneficial, and how to master it with real-world examples and best practices.
Table of Contents
1. What is Function Chaining?
Function chaining is a coding technique that involves calling multiple functions on the same object or result in a single line of code. It enables you to execute a series of operations sequentially, without the need for intermediate variables or separate lines of code. Function chaining is commonly used with methods that return the object they operate on, allowing you to keep modifying the same object in a fluent manner.
Consider this example:
javascript const numbers = [1, 2, 3, 4, 5]; // Without function chaining let sum = numbers.reduce((acc, num) => acc + num, 0); let squaredSum = Math.pow(sum, 2); console.log(squaredSum); // Output: 225 // With function chaining const result = numbers.reduce((acc, num) => acc + num, 0).pow(2); console.log(result); // Output: 225
In the above example, function chaining allowed us to calculate the sum of the numbers array and then immediately square that sum, all in one line of code. This concise and readable code is one of the primary benefits of function chaining.
2. Why Should You Use Function Chaining?
Function chaining offers several advantages that make it a valuable tool in your JavaScript development toolkit:
2.1. Improved Code Readability
One of the main benefits of function chaining is that it leads to more readable code. By chaining functions together, you can clearly express a sequence of operations, making the code easier to understand and maintain. This is particularly useful when working with complex data manipulations or multiple transformations on the same data.
2.2. Reduced Intermediary Variables
Function chaining allows you to eliminate the need for intermediate variables, reducing clutter and potential naming conflicts in your code. Without function chaining, you might need to create several variables to hold intermediate results, leading to bloated code.
2.3. Concise Code Structure
By combining multiple operations into a single line of code, function chaining promotes a more concise code structure. It can help you achieve more with fewer lines of code, making your codebase cleaner and easier to manage.
2.4. Method Chaining with Libraries
Many JavaScript libraries and frameworks take advantage of function chaining to provide fluent APIs that read like natural language. When you master function chaining, you’ll be better equipped to work with these libraries and write more expressive code.
3. Basic Function Chaining
Let’s dive into some examples of basic function chaining to understand how it works and its applications.
3.1. Chaining Built-in Array Methods
JavaScript’s array methods, such as map(), filter(), and reduce(), are well-suited for function chaining. Let’s see how we can chain these methods together:
javascript const numbers = [1, 2, 3, 4, 5]; // Chaining array methods const result = numbers .filter((num) => num % 2 === 0) // Filter even numbers .map((num) => num * 2) // Double each number .reduce((acc, num) => acc + num, 0); // Sum the doubled numbers console.log(result); // Output: 24
In the above example, we chained filter(), map(), and reduce() to filter even numbers, double them, and then calculate their sum.
3.2. Chaining Custom Functions
You can also chain custom functions together, as long as they return the object they are operating on. Here’s an example:
javascript // Custom function that returns the object it operates on function add(x) { return { value: x, plus: function (y) { this.value += y; return this; }, getValue: function () { return this.value; }, }; } const result = add(5).plus(3).plus(2).getValue(); console.log(result); // Output: 10
In this example, the add() function returns an object with a plus() method that adds a value to the current object’s value property. The plus() method returns the object itself, allowing us to chain multiple plus() calls together.
4. Intermediate Function Chaining
As you become more proficient with function chaining, you can explore some intermediate techniques that further enhance your code.
4.1. Returning the Same Object
When chaining methods, it’s common to return a new object or result after each operation. However, in some cases, you might want to return the same object to perform multiple operations on it. Be cautious when using this approach, as it can lead to unexpected behavior if not handled properly.
Consider the following example:
javascript const calculator = { value: 0, add: function (x) { this.value += x; return this; }, multiply: function (x) { this.value *= x; return this; }, getValue: function () { return this.value; }, }; const result = calculator.add(5).multiply(3).getValue(); console.log(result); // Output: 15
In this example, the calculator object maintains its state throughout the chaining process, allowing us to call add() and multiply() consecutively on the same object.
4.2. Handling Errors in Function Chaining
When chaining multiple methods, it’s essential to handle potential errors gracefully. If one of the chained methods encounters an error, it should propagate the error to the next method or provide a fallback behavior.
Let’s consider a scenario where we’re chaining methods to fetch data from an API:
javascript function fetchData() { return fetch('https://api.example.com/data') .then((response) => { if (!response.ok) { throw new Error('Failed to fetch data'); } return response.json(); }); } function processData(data) { // Process the fetched data } fetchData() .then(processData) .then(() => console.log('Data processing successful')) .catch((error) => console.error('Error:', error.message));
In this example, if the fetchData() function encounters an error while fetching the data, it throws an error. This error is propagated to the next method in the chain using the catch() method, where it is appropriately handled.
5. Advanced Function Chaining Techniques
Function chaining becomes even more powerful when applied to advanced scenarios, such as asynchronous JavaScript and creating fluent APIs.
5.1. Chaining in Asynchronous JavaScript
In modern JavaScript, asynchronous operations are common, and chaining them can be challenging due to their non-blocking nature. However, you can achieve function chaining with asynchronous operations using promises or the async/await syntax.
Consider this example using promises:
javascript function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => resolve('Data fetched'), 1000); }); } function processData(data) { return new Promise((resolve, reject) => { setTimeout(() => resolve(`${data} and processed`), 500); }); } fetchData() .then(processData) .then((result) => console.log(result)) .catch((error) => console.error('Error:', error.message));
In this example, the fetchData() function returns a promise that resolves after a timeout, simulating an asynchronous operation. We can then chain the processData() function to handle the data once it’s fetched.
5.2. Fluent APIs with Function Chaining
Fluent APIs are interfaces designed to be readable and resemble natural language. Function chaining plays a crucial role in creating fluent APIs, making your codebase more expressive and user-friendly.
Let’s create a simple fluent API for a fictional drawing library:
javascript class Drawing { constructor() { this.shapes = []; } circle(radius) { this.shapes.push(`Circle with radius ${radius}`); return this; } square(side) { this.shapes.push(`Square with side ${side}`); return this; } rectangle(width, height) { this.shapes.push(`Rectangle with width ${width} and height ${height}`); return this; } render() { console.log('Drawing the following shapes:'); this.shapes.forEach((shape) => console.log(shape)); } } const drawing = new Drawing(); drawing.circle(5).square(8).rectangle(10, 6).render();
In this example, the Drawing class contains methods for drawing shapes, and each method returns the same object, allowing us to chain multiple shape-drawing calls together.
6. Best Practices for Function Chaining
While function chaining can be a powerful tool, it’s essential to use it judiciously and follow best practices to maintain code readability and avoid potential pitfalls.
6.1. Keep it Readable
Always prioritize code readability over brevity. Avoid chaining too many methods in a single line, as it can lead to hard-to-read code. Break long chains into separate lines with proper indentation and formatting.
6.2. Error Handling and Graceful Failures
Ensure that your function chains handle errors gracefully. If a method in the chain can potentially throw an error, use the catch() method to handle and log the errors appropriately.
6.3. Mind the Performance
While function chaining can improve code structure, be mindful of performance implications. Chaining too many methods might result in reduced performance, especially when working with large datasets or complex computations. Consider optimizing your code if you notice any significant performance bottlenecks.
Conclusion
Function chaining is a powerful technique in JavaScript that can streamline your development workflow and make your code more elegant and maintainable. By mastering function chaining, you can significantly improve your code’s readability and conciseness while keeping your development process efficient and effective.
In this blog, we covered the basics of function chaining, its benefits, and explored various examples, including chaining built-in array methods, chaining custom functions, and handling asynchronous operations. Additionally, we looked into creating fluent APIs with function chaining and outlined best practices to ensure clean and robust code.
By incorporating function chaining into your JavaScript development, you can write more expressive, efficient, and streamlined code that will make your projects more enjoyable to work on and maintain. Happy coding!
Table of Contents
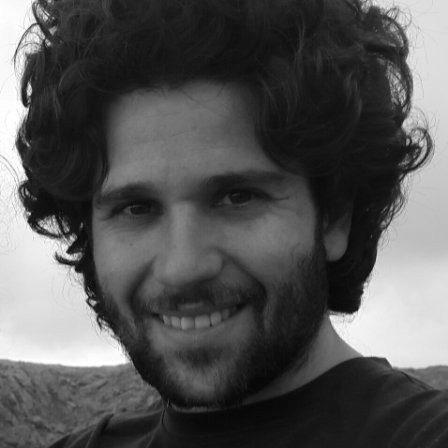
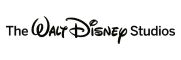