JavaScript Function Optimization: Strategies for Faster Execution
In the world of web development, JavaScript is the powerhouse that drives interactivity and dynamic behavior on websites. As developers, we strive to create efficient and fast-running code to provide users with a seamless experience. One crucial aspect of achieving this goal is optimizing JavaScript functions for faster execution. In this blog, we’ll delve into various strategies, techniques, and best practices that can significantly enhance the performance of your JavaScript functions.
Table of Contents
1. Optimization Strategies
1.1. Choose the Right Data Structures
Selecting appropriate data structures can have a significant impact on the performance of your JavaScript functions. Arrays are excellent for linear data access, while objects excel at key-value pair storage. Maps and Sets are advantageous for specific use cases like fast lookups and uniqueness. By using the right data structure for your task, you can avoid unnecessary iterations and achieve faster execution.
javascript // Using an object for fast data retrieval const userScores = { 'Alice': 95, 'Bob': 85, 'Eve': 92 }; // Iterating through an array const numbers = [10, 20, 30, 40, 50]; let sum = 0; for (const num of numbers) { sum += num; }
1.2. Minimize DOM Manipulations
DOM manipulations are notorious for their impact on performance. When modifying the DOM, the browser has to recalculate styles, layouts, and repaints, which can cause significant delays. Minimize DOM updates by batching changes or using techniques like creating elements in memory before adding them to the DOM. Additionally, consider using CSS classes for multiple updates instead of changing styles individually.
javascript // Inefficient: Multiple DOM manipulations const element = document.getElementById('myElement'); element.style.width = '200px'; element.style.height = '100px'; element.textContent = 'Updated content'; // Efficient: Batch DOM manipulations const element = document.getElementById('myElement'); element.classList.add('updated-style'); element.textContent = 'Updated content';
1.3. Utilize Caching
Caching involves storing the results of expensive function calls and reusing them when needed. This strategy is particularly useful for functions that perform repetitive computations or fetch data from external sources. Caching can be implemented using techniques such as memoization, where function results are stored based on their input arguments.
javascript // Without caching function fibonacci(n) { if (n <= 1) return n; return fibonacci(n - 1) + fibonacci(n - 2); } // With caching (memoization) function fibonacciMemoized() { const cache = {}; return function(n) { if (n in cache) return cache[n]; if (n <= 1) return n; cache[n] = fibonacci(n - 1) + fibonacci(n - 2); return cache[n]; }; } const memoizedFibonacci = fibonacciMemoized();
1.4. Debouncing and Throttling
Debouncing and throttling are techniques used to optimize functions that are triggered frequently, such as in response to user input or window resizing. Debouncing limits the execution of a function until a certain time has passed since the last invocation, while throttling limits the rate at which a function is called. These techniques can prevent performance issues caused by rapid, repeated function calls.
javascript // Debouncing function to limit frequent API calls function debounce(func, delay) { let timer; return function() { clearTimeout(timer); timer = setTimeout(() => { func.apply(this, arguments); }, delay); }; } const debouncedSearch = debounce(searchFunction, 300); // Throttling function to limit event handler calls function throttle(func, delay) { let isThrottled = false; return function() { if (isThrottled) return; isThrottled = true; func.apply(this, arguments); setTimeout(() => { isThrottled = false; }, delay); }; } const throttledScroll = throttle(scrollHandler, 200);
1.5. Avoid Synchronous Operations
Synchronous operations can block the main thread and lead to unresponsive user interfaces. Asynchronous operations, on the other hand, allow non-blocking execution and better user experiences. Utilize features like Promises and the async/await syntax to handle asynchronous tasks efficiently and ensure that your JavaScript functions do not introduce unnecessary delays.
javascript // Synchronous operation function syncFunction() { const data = fetchData(); // This might take a while process(data); } // Asynchronous operation with async/await async function asyncFunction() { const data = await fetchData(); // Non-blocking process(data); }
Optimizing JavaScript functions goes beyond these strategies. In part two of this blog, we’ll explore additional techniques, including code compilation and minification, memory
management, web workers, hardware acceleration, and continuous monitoring. Stay tuned for more insights on how to make your JavaScript functions lightning-fast!
Conclusion
Efficient JavaScript functions are the backbone of a performant web application. By implementing the optimization strategies discussed in this blog, you can significantly improve the execution speed of your code. Remember that performance optimization is an ongoing process, and continuous monitoring and refinement are essential to ensure your code remains fast and responsive. With the right tools, techniques, and practices, you can provide users with a seamless and enjoyable experience on your website.
Table of Contents
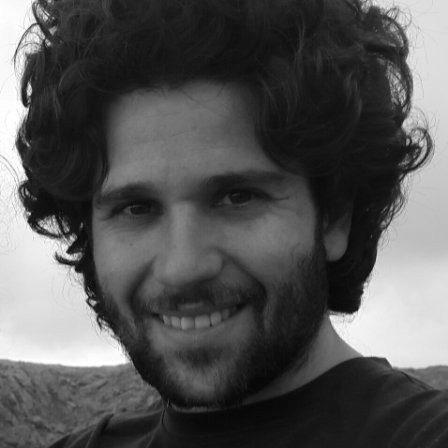
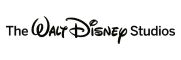