Exploring JavaScript Function Wrappers: A Comprehensive Guide
JavaScript, the versatile scripting language, is a cornerstone of modern web development. It provides developers with the tools to create dynamic and interactive web applications. One of the key features that makes JavaScript so powerful is its ability to manipulate functions. Function wrappers, also known as function decorators or higher-order functions, are an advanced concept that can greatly enhance your coding capabilities. In this comprehensive guide, we will delve into the world of JavaScript function wrappers, exploring their definition, use cases, benefits, and providing you with practical code samples to grasp the concept effectively.
Table of Contents
1. Understanding Function Wrappers
At its core, a function wrapper is a higher-order function that takes another function as an argument and returns a new function with enhanced behavior. In other words, it allows you to modify the behavior of a function without modifying the function itself. This concept of wrapping functions is widely used in JavaScript for various purposes, such as adding logging, caching, access control, or altering the input/output of functions.
1.1. The Anatomy of a Function Wrapper
A function wrapper typically follows this structure:
javascript function wrapper(originalFunction, ...args) { // Code to execute before the original function call // ... const result = originalFunction(...args); // Code to execute after the original function call // ... return result; }
In this structure, originalFunction represents the function that you want to wrap, and args are the arguments passed to that function. The wrapper function can contain code that runs before and after the original function call, effectively allowing you to augment its behavior.
2. Common Use Cases for Function Wrappers
2.1. Logging
Logging is a crucial aspect of debugging and monitoring applications. By wrapping functions with logging logic, you can easily track function calls, their inputs, and outputs.
javascript function logWrapper(originalFunction, ...args) { console.log(`Calling ${originalFunction.name} with arguments:`, args); const result = originalFunction(...args); console.log(`Function ${originalFunction.name} returned:`, result); return result; } // Usage const wrappedAdd = logWrapper(add); wrappedAdd(2, 3); // Output will be logged
2.2. Caching
Function wrappers are also handy for caching expensive function calls, improving performance by avoiding redundant computations.
javascript function cacheWrapper(originalFunction, ...args) { const cacheKey = JSON.stringify(args); if (cacheKey in cache) { return cache[cacheKey]; } const result = originalFunction(...args); cache[cacheKey] = result; return result; } // Usage const cachedMultiply = cacheWrapper(multiply); cachedMultiply(4, 5); // The result will be cached for future calls
2.3. Access Control
Function wrappers can enforce access control mechanisms by restricting the execution of certain functions based on user roles or permissions.
javascript function accessControlWrapper(originalFunction, user) { if (user.isAdmin) { return originalFunction; } else { return () => { throw new Error("Access denied"); }; } } // Usage const adminDeleteUser = accessControlWrapper(deleteUser, { isAdmin: true }); const regularDeleteUser = accessControlWrapper(deleteUser, { isAdmin: false }); adminDeleteUser(); // Executes deleteUser regularDeleteUser(); // Throws an access denied error
3. Benefits of Using Function Wrappers
3.1. Reusability
Function wrappers promote code reusability by separating cross-cutting concerns from core logic. You can apply the same wrapper to multiple functions without duplicating code.
3.2. Modularity
Wrappers enhance modularity by allowing you to add and remove features without modifying the original function. This prevents tightly coupled code and makes maintenance easier.
3.3. Debugging and Profiling
With function wrappers, you can add debugging or profiling logic globally, making it easier to monitor the behavior of your application’s functions.
3.4. Code Readability
By isolating specific functionality within wrappers, your main functions remain concise and focused on their primary tasks, leading to cleaner and more readable code.
4. Implementing Function Wrappers in Real Projects
To fully grasp the power of function wrappers, let’s explore how they can be applied in a real-world scenario.
Consider a scenario where you’re building an e-commerce platform. You have a variety of functions that handle cart operations, such as adding items, removing items, and calculating totals. You want to introduce logging and access control to these functions. Instead of cluttering your cart functions with this additional code, you can use wrappers.
javascript function logWrapper(originalFunction, ...args) { console.log(`Calling ${originalFunction.name} with arguments:`, args); const result = originalFunction(...args); console.log(`Function ${originalFunction.name} returned:`, result); return result; } function accessControlWrapper(originalFunction, user) { if (user.isLoggedIn) { return originalFunction; } else { return () => { throw new Error("Access denied"); }; } } const cart = { items: [], addItem: accessControlWrapper(logWrapper(function(item) { cart.items.push(item); return cart.items; }), { isLoggedIn: true }), // Other cart methods... };
In this example, the addItem function is wrapped with both logging and access control logic. This approach maintains clean, focused cart functions while adding desired features.
Conclusion
JavaScript function wrappers are a powerful tool in a developer’s arsenal. They provide the ability to modify, enhance, and manage the behavior of functions without altering their core logic. By leveraging function wrappers, you can achieve cleaner code, improved modularity, and easier debugging. Whether you’re working on a simple project or a complex application, understanding and effectively using function wrappers can significantly enhance your coding capabilities. So, start exploring function wrappers and unlock new possibilities in your JavaScript programming journey. Happy coding!
Table of Contents
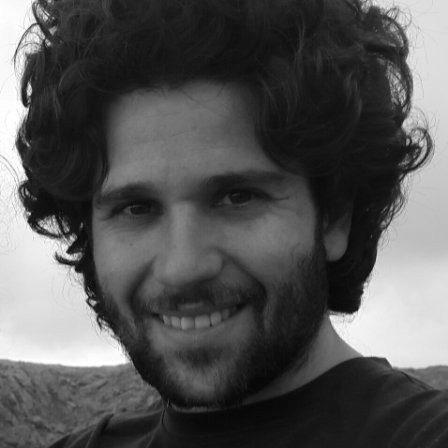
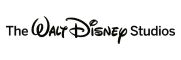