A Comprehensive JavaScript Developer Interview Questions Guide
JavaScript, the dynamic programming language, has transformed the realm of web development. To craft exceptional web applications, hiring skilled JavaScript developers is essential. This guide serves as your compass through the hiring journey, empowering you to evaluate candidates’ technical prowess, problem-solving abilities, and JavaScript expertise. Let’s embark on this exploration, uncovering essential interview questions and strategies to identify the perfect JavaScript developer for your team.
Table of Contents
1. How to Hire JavaScript Developers
Embarking on the journey to hire JavaScript developers? Follow these steps for a successful voyage:
- Job Requirements: Define specific job requirements, outlining the skills and experience you’re seeking.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ JavaScript proficiency, relevant experience, and additional skills.
- Technical Assessment: Develop a comprehensive technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of JavaScript Developers to Look For
When assessing JavaScript developers, keep an eye out for these pivotal skills:
- JavaScript Mastery: A strong understanding of JavaScript syntax, principles, and concepts.
- Front-End Proficiency: Familiarity with HTML, CSS, and JavaScript integration for creating dynamic user interfaces.
- DOM Manipulation: Skill in manipulating the Document Object Model to create interactive web experiences.
- Asynchronous Programming: Expertise in handling asynchronous operations using callbacks, promises, and async/await.
- Web API Familiarity: Knowledge of working with browser APIs, including Fetch API, Web Storage, and Geolocation.
- ES6+ Knowledge: Proficiency in modern JavaScript features like arrow functions, template literals, and destructuring.
- Problem-Solving Skills: Ability to dissect complex problems and devise efficient solutions.
3. Overview of the JavaScript Developer Hiring Process
Here’s a bird’s-eye view of the JavaScript developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create captivating job descriptions that accurately convey the role, attracting the right candidates.
3.3 Crafting JavaScript Developer Interview Questions
Develop a comprehensive set of interview questions covering JavaScript intricacies, problem-solving aptitude, and relevant technologies.
4. Sample JavaScript Developer Interview Questions and Answers
Explore these sample questions with in-depth answers to assess candidates’ JavaScript skills:
Q1. Explain the concept of closures in JavaScript and provide an example.
A: Closures are functions that remember the variables from the outer scope even after the outer function has finished executing. An example:
function outer() { const outerVar = 'I am outer'; return function inner() { console.log(outerVar); }; } const innerFunc = outer(); innerFunc(); // Outputs: I am outer
Q2. What is the Event Loop in JavaScript, and how does it work?
A: The Event Loop is a mechanism that allows JavaScript to handle asynchronous operations by executing callback functions in a non-blocking way. It manages the execution of code in the call stack and the task queue.
Q3. Write a function in JavaScript that returns a promise to fetch data from an API.
function fetchData(url) { return new Promise((resolve, reject) => { fetch(url) .then(response => response.json()) .then(data => resolve(data)) .catch(error => reject(error)); }); }
Q4. Explain the concept of prototype inheritance in JavaScript and provide an example.
A: Prototype inheritance in JavaScript allows objects to inherit properties and methods from other objects. An example:
function Animal(name) { this.name = name; } Animal.prototype.sayHello = function() { console.log(`Hello, I'm ${this.name}`); }; function Cat(name) { Animal.call(this, name); } Cat.prototype = Object.create(Animal.prototype); Cat.prototype.constructor = Cat; const cat = new Cat('Whiskers'); cat.sayHello(); // Outputs: Hello, I'm Whiskers
Q5. How does JavaScript handle data types, and what are the primitive data types?
A: JavaScript uses dynamic typing, where variables can hold values of any data type. Primitive data types in JavaScript include number
, string
, boolean
, null
, undefined
, and symbol
.
Q6. Write a JavaScript function that uses the map function to double each element in an array.
function doubleArray(arr) { return arr.map(item => item * 2); }
Q7. Explain the purpose of JavaScript’s this
keyword and how it behaves in different contexts.
A: The this
keyword refers to the current execution context. Its behavior varies depending on where it’s used, such as in a global context, function, object method, or event handler.
Q8. What is the difference between null
and undefined
in JavaScript?
A: null
represents the intentional absence of any value, while undefined
indicates that a variable has been declared but has not been assigned a value.
Q9. Write a JavaScript function that returns the sum of all even numbers in an array.
function sumEvenNumbers(arr) { return arr.reduce((sum, num) => (num % 2 === 0 ? sum + num : sum), 0); }
Q10. How do you handle errors and exceptions in JavaScript?
A: Errors and exceptions in JavaScript can be handled using try...catch
blocks to gracefully handle exceptions and prevent program crashes.
5. Hiring JavaScript Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s requirements, preferred skills, and expected experience.
Step 2: Find Your Ideal Match: Within 24 hours, CloudDevs presents you with carefully selected JavaScript developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial: Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional JavaScript developers, ensuring your team possesses the skills required to craft extraordinary web applications.
6. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to evaluate JavaScript developers comprehensively. Whether you’re scripting dynamic behaviors or crafting robust applications, securing the right JavaScript developers for your team is the cornerstone of your project’s triumph.
Table of Contents
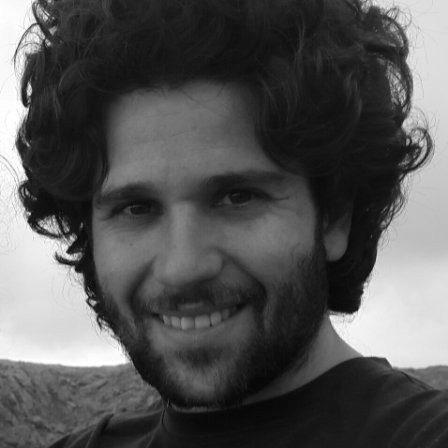
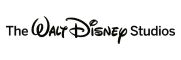