Unpacking the Power of Functions in JavaScript: From Simple Functions to Higher-Order Functions
Welcome to this comprehensive guide on JavaScript functions, where we’ll demystify the core concepts of JavaScript functions and learn how to use them. Whether you’re an absolute beginner, a seasoned professional, or someone looking to hire JavaScript developers, this post is designed to help you master the basics, improve your coding skills, and better understand what skills to look for in potential hires.
Introduction to JavaScript Functions
In JavaScript, functions are first-class objects. This means they share properties with other objects like strings, numbers, or arrays; they can be passed as arguments to other functions, returned as values from other functions, assigned to variables or stored in data structures. This distinctive feature makes JavaScript functions highly valuable and essential for those planning to hire JavaScript developers.
A JavaScript function is a defined block of code designed to perform a specific task. Its primary goal is to enable you to structure your code in a way that is reusable, maintainable, and easy to debug. Understanding these fundamental aspects of JavaScript functions is not only important for developers, but also for those who wish to hire JavaScript developers, as this knowledge aids in assessing potential candidates’ coding prowess and their ability to write efficient, maintainable code.
.
Declaring a Function
Functions in JavaScript are declared with the `function` keyword, followed by a name and a set of parentheses `()`. The code to be executed by the function is placed inside curly brackets `{}`. Here is a simple example:
```javascript function greeting() { console.log("Hello, World!"); } ```
This function, named `greeting`, prints “Hello, World!” when called.
Calling a Function
To execute the function, we need to call or invoke it. This is done by writing the name of the function followed by parentheses `()`. Let’s call the `greeting` function:
```javascript greeting(); // Outputs: "Hello, World!" ```
Function Parameters and Arguments
A function can also accept parameters. Parameters are a mechanism to pass values to functions. The function can then use these parameters to perform its task. Let’s look at an example:
```javascript function greeting(name) { console.log("Hello, " + name + "!"); } greeting("Alice"); // Outputs: "Hello, Alice!" ```
Here, the function `greeting` accepts one parameter `name`. When we call the function, we pass the argument “Alice” to the `name` parameter.
Return Statement
A function can also return a value. The `return` statement stops the execution of a function and returns a value from that function. Here is an example:
```javascript function square(number) { return number * number; } var result = square(5); // result is now 25 ```
In this example, the `square` function accepts a parameter `number` and returns the square of this number.
Function Expressions and Anonymous Functions
JavaScript supports not just function declarations but also function expressions. A function expression is a function defined inside an expression. An anonymous function is a function without a name. It’s often used in function expressions.
```javascript var square = function(number) { return number * number }; var result = square(4); // result is now 16 ```
Here, an anonymous function is assigned to the variable `square`. We can then call `square` as if it’s a function, because it is!
Arrow Functions
Introduced in ES6, arrow functions offer a more concise syntax to write function expressions. They are also lexically bound, which means `this` value inside an arrow function is determined by its surrounding context. Here’s how you can define the `square` function as an arrow function:
```javascript const square = number => number * number; let result = square(6); // result is now 36 ```
Arrow functions are particularly useful when passing a function as an argument to another function. For instance:
```javascript let numbers = [1, 2, 3, 4, 5]; let squares = numbers.map(number => number * number); // squares is now [1, 4, 9, 16, 25] ```
In the above example, we pass an arrow function as an argument to the `map` method.
Higher-Order Functions and Callbacks
As we mentioned earlier, functions are first-class objects in JavaScript. This means we can pass functions as arguments to other functions and return functions from functions. Functions that operate on other functions are known as Higher-Order Functions.
A callback function is a function that is passed as an argument to another function and is executed after some operation has been completed. Below is an example of a callback function:
```javascript function greeting(name, callback) { let message = 'Hello ' + name + '!'; callback(message); } greeting('John', function(message) { console.log(message); // Outputs: "Hello, John!" }); ```
Closure
In JavaScript, a closure is a function which has access to the outer (enclosing) function’s variables—scope chain. This means that a function nested inside another function has access to the outer function’s variables. Here is an example:
```javascript function outerFunction(outerVariable) { return function innerFunction(innerVariable) { console.log('outerVariable:', outerVariable); console.log('innerVariable:', innerVariable); } } const newFunction = outerFunction('outside'); newFunction('inside'); // Logs: outerVariable: outside, innerVariable: inside ```
In this example, `innerFunction` has access to variables in `outerFunction`’s scope, which is a demonstration of a closure.
Conclusion
Functions in JavaScript are powerful tools that enable the creation of more modular and maintainable code. They range from simple function declarations to more complex higher-order functions and closures. Understanding these tools is fundamental to becoming a successful JavaScript developer, and crucial for those who wish to hire JavaScript developers, as this knowledge allows them to discern truly proficient candidates.
Whether you’re embarking on your JavaScript journey, brushing up on the basics, or aiming to hire JavaScript developers, mastering functions will elevate your programming skills or hiring acumen to the next level. So, roll up your sleeves, create functions, experiment with the provided examples, and embark on a rewarding coding journey!
Table of Contents
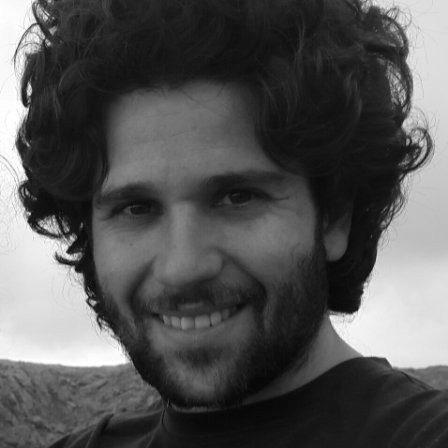
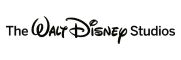