From Uploads to Downloads: Navigating Laravel’s Versatile File Storage System
When developing web applications, handling file uploads and downloads is a common task. Laravel, a powerful PHP framework used by expert Laravel developers, makes this straightforward with its built-in file storage system. The file storage system in Laravel not only allows you to manage uploads and downloads but also abstracts file storage, enabling the use of various file systems such as local, Amazon S3, and even FTP. This is one of the reasons why businesses choose to hire Laravel developers. In this post, we will delve into how to manage uploads and file systems using Laravel’s File Storage, giving you a glimpse into the skills and capabilities you access when you hire Laravel developers.
What is Laravel File Storage?
Laravel’s file storage is an abstraction layer powered by the Flysystem PHP package. This layer provides simple to use drivers for working with local file systems, Amazon S3, and FTP servers. Its simplicity allows developers to switch between these systems effortlessly and without changing the underlying code base. This flexibility comes in handy when you decide to move from storing files locally to a more scalable solution like Amazon S3.
Configuring File Systems
To start using Laravel’s file storage, you need to configure your file systems. Laravel’s filesystem configuration file is located at `config/filesystems.php`. This file includes several configuration options.
```php 'default' => env('FILESYSTEM_DRIVER', 'local'), 'disks' => [ 'local' => [ 'driver' => 'local', 'root' => storage_path('app'), ], 'public' => [ 'driver' => 'local', 'root' => storage_path('app/public'), 'url' => env('APP_URL').'/storage', 'visibility' => 'public', ], 's3' => [ 'driver' => 's3', 'key' => env('AWS_ACCESS_KEY_ID'), 'secret' => env('AWS_SECRET_ACCESS_KEY'), 'region' => env('AWS_DEFAULT_REGION'), 'bucket' => env('AWS_BUCKET'), ], ], ```
Each disk represents a particular storage driver and its associated configurations. The `default` option defines which disk Laravel will use when no specific disk is selected.
File Uploads
Uploading files with Laravel is a breeze. Let’s consider an example where a user uploads a profile picture.
In your form, you will need to include a file input field.
```html <form action="/profile" method="POST" enctype="multipart/form-data"> @csrf <input type="file" name="avatar"> <button type="submit">Submit</button> </form> ```
In the corresponding controller, you would then handle the file upload as follows:
```php public function update(Request $request) { $request->validate([ 'avatar' => 'required|image', ]); $path = $request->file('avatar')->store('avatars'); return $path; } ```
The `store` method will generate a unique ID for the file and store it in the disk specified. If no disk is mentioned, Laravel will use the default disk. The method returns the file path, which can be stored in the database for later retrieval.
File Downloads
Downloading files is also straightforward with Laravel. Let’s assume you want to download the avatar uploaded in the previous example. You can create a route that retrieves and downloads the file:
```php Route::get('/avatars/{path}', function ($path) { return Storage::download('avatars/'.$path); }); ```
The `download` method will read the file from the disk and download it to your computer. If the file is not found, a 404 HTTP response is sent back.
File Visibility
Laravel’s file storage also handles file visibility. By default, all files are private, which means they can’t be accessed directly via a URL. However, you can change a file’s visibility to make it publicly accessible. This feature can be useful when dealing with files like profile pictures, which need to be publicly accessible. Here’s how you can make a file public:
```php $path = $request->file('avatar')->storePublicly('avatars'); ```
For files already uploaded, you can change their visibility using the `setVisibility` method:
```php Storage::setVisibility($path, 'public'); ```
Switching File Systems
One of the best features of Laravel’s file storage is the ability to switch between file systems with ease. This can be done using the `disk` method, which allows you to interact with any configured disk:
```php $path = $request->file('avatar')->store('avatars', 's3'); ```
In this example, the file is stored in the Amazon S3 disk instead of the local disk. Similarly, you can retrieve or delete files from any disk:
```php Storage::disk('s3')->get($path); Storage::disk('s3')->delete($path); ```
Conclusion
Laravel’s file storage system is an invaluable tool for developers, including those businesses might hire as Laravel developers. It abstracts the complexity of dealing with file uploads, downloads, and different file storage systems, making it easier for developers to focus on building the core functionalities of their web applications. With the simplicity and flexibility it provides, managing files has never been easier. Regardless of whether you’re storing files locally, on Amazon S3, or any other supported file system, Laravel’s got you covered.
This robust toolset is one of the many reasons why businesses opt to hire Laravel developers for their projects.
In this article, we’ve only touched the surface of what Laravel’s file storage can do. For more advanced features like streaming downloads, file URLs, and custom file systems, businesses often hire Laravel developers for their expert knowledge. Do check out Laravel’s official documentation for a deeper dive. Happy coding!
Table of Contents
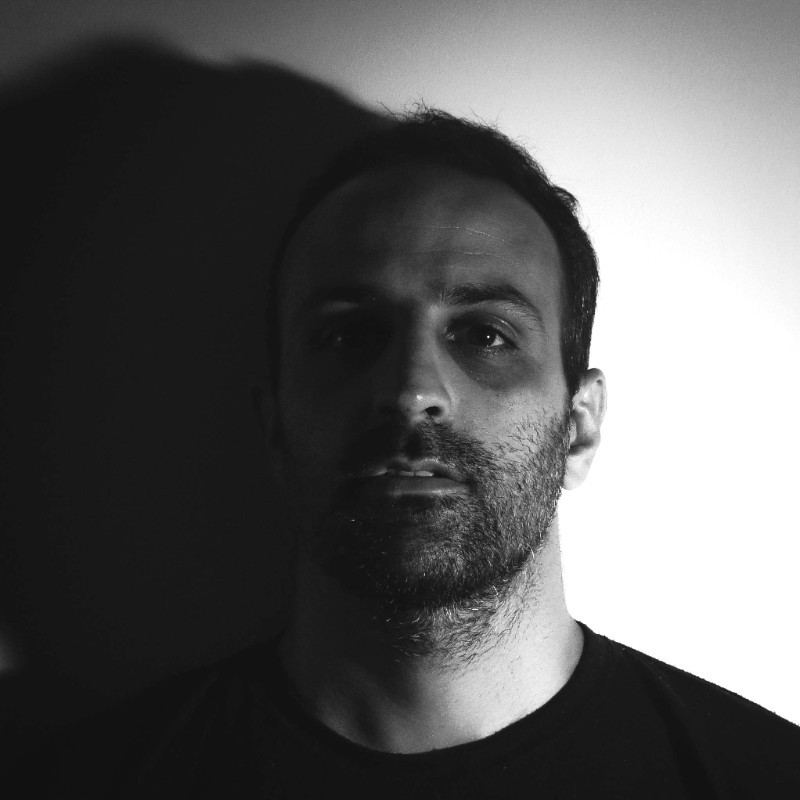
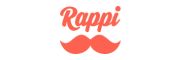