Laravel Localization: Building Multilingual Web Applications
In today’s globalized world, building multilingual web applications is a common requirement. Users from different regions and cultures expect content that is presented in their native language. Laravel, a popular PHP framework, provides robust support for implementing localization in web applications. Localization allows developers to easily translate their application’s content into multiple languages, making it accessible to a wider audience.
This comprehensive guide will walk you through the process of implementing localization in Laravel. We will explore the necessary steps, best practices, and code samples to ensure that your web application is fully multilingual and user-friendly.
Understanding Localization in Laravel
Localization refers to the process of adapting an application’s content, such as text strings, dates, numbers, and formatting, to suit the cultural and language preferences of different regions. Laravel simplifies the localization process by providing a powerful translation system.
The Localization Configuration
Before diving into the implementation, let’s start by configuring Laravel for localization. Laravel’s configuration file config/app.php contains a locale key, which determines the default locale for your application. This default locale will be used if no other locale is explicitly specified.
To change the default locale, modify the locale key in config/app.php to the desired language code, such as ‘en’ for English or ‘fr’ for French.
Setting Up Language Files
Laravel uses language files to store translations for different locales. By default, these files are located in the resources/lang directory. Each language file corresponds to a specific locale and contains an array of key-value pairs representing the translated strings.
To add a new language file, create a new directory inside resources/lang with the desired locale code, such as fr for French. Then, create a new file within that directory, such as messages.php, and define the translated strings in an array format.
Here’s an example of a language file (resources/lang/fr/messages.php) containing French translations:
php <?php return [ 'welcome' => 'Bienvenue sur notre site web!', 'greeting' => 'Bonjour, :name!', ];
In this example, the key ‘welcome’ maps to the French translation ‘Bienvenue sur notre site web!’, and the key ‘greeting’ includes a placeholder :name that will be replaced dynamically.
Translating Text Strings
Once you have set up the language files, you can start translating text strings in your views or application logic. Laravel provides a helper function called __() to translate strings.
Here’s an example of translating a string in a view:
php <!DOCTYPE html> <html> <head> <title>{{ __('Welcome') }}</title> </head> <body> <h1>{{ __('Welcome to our website!') }}</h1> </body> </html>
In this example, the __(‘Welcome’) helper function is used to translate the title of the page, while __(‘Welcome to our website!’) translates the heading.
Using Placeholders and Variables in Translations
Often, translated strings may contain placeholders or dynamic variables that need to be replaced at runtime. Laravel’s localization system supports this with a syntax similar to the one used in the language files.
Here’s an example of using a placeholder in a translation string:
php $greeting = __('Hello, :name!', ['name' => 'John']);
In this example, the translated string for ‘Hello, :name!’ will replace :name with the value ‘John’.
Pluralization
Handling pluralization is a common requirement in multilingual applications. Laravel provides a simple and intuitive way to handle plural forms in translations.
Here’s an example of pluralization in a language file:
php return [ 'apples' => '{0} No apples|{1} :count apple|[2,*] :count apples', ];
In this example, the key ‘apples’ contains different variations based on the count of apples. The {0}, {1}, and [2,*] are the pluralization rules. The format is {count} translation for each rule.
To use the pluralized string in your application, you can utilize the trans_choice() helper function:
php echo trans_choice('messages.apples', 5, ['count' => 5]);
This will output ‘5 apples’ in this case
Switching Between Locales
In addition to the default locale, Laravel allows you to switch between different locales dynamically. This is useful when users want to view the application in a language other than the default.
To set the locale dynamically, you can use the app()->setLocale() method:
php app()->setLocale('fr');
In this example, we set the locale to French (‘fr’). From this point forward, Laravel will use the French translations defined in the language files.
Conclusion
Implementing localization in Laravel is essential for building multilingual web applications that cater to a diverse audience. By following the steps outlined in this guide, you can easily configure Laravel for localization, set up language files, translate text strings, handle pluralization, and switch between different locales.
Harnessing Laravel’s powerful localization features ensures that your application is accessible and user-friendly, allowing you to reach a wider audience and provide an exceptional user experience.
Remember, localization goes beyond translation. Understanding cultural nuances and adapting your application’s content to different regions will help you create a truly immersive and personalized experience for your users.
So, why limit your application to a single language when you can easily make it multilingual with Laravel’s localization capabilities? Start building your multilingual web application today!
Table of Contents
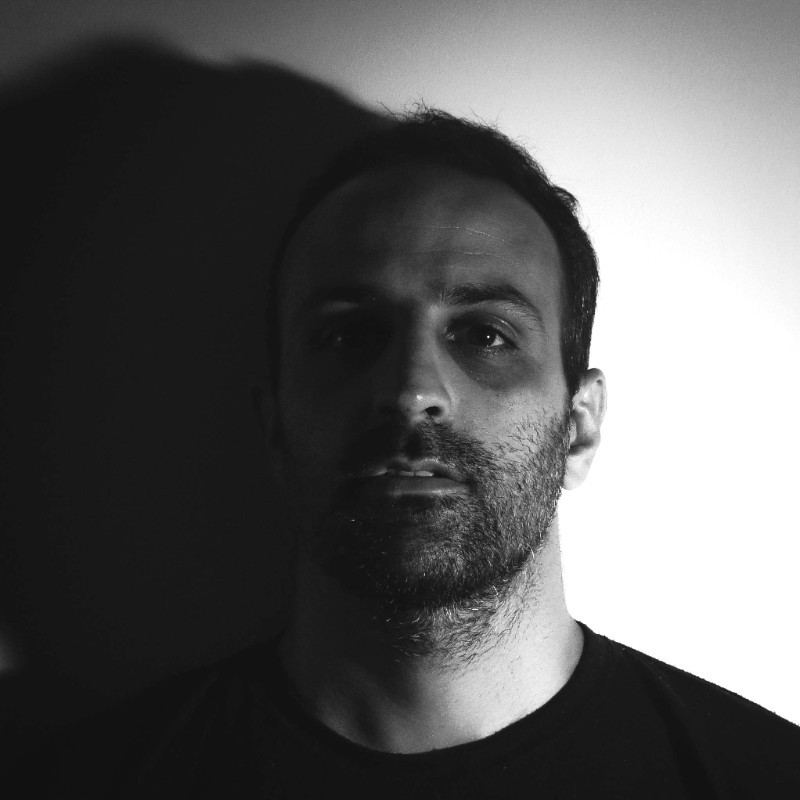
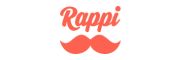