Creating Command-Line Tools with Node.js
In the world of software development, command-line tools play a crucial role in enhancing productivity, automating tasks, and performing various operations efficiently. Whether you’re a beginner or an experienced developer, creating your own command-line tools using Node.js can be an empowering skill. This comprehensive guide will walk you through the process of building command-line tools using Node.js, from the basics to advanced concepts, along with practical examples and best practices.
Table of Contents
1. Introduction to Command-Line Tools
Command-line tools are programs designed to be executed through a command-line interface (CLI). They provide a convenient way to interact with a computer’s operating system and perform tasks without the need for a graphical user interface (GUI). Command-line tools are widely used for various purposes, such as system administration, automation, data processing, and more.
2. Setting Up Your Development Environment
Before diving into building command-line tools, ensure you have Node.js and npm (Node Package Manager) installed on your system. You can download and install them from the official Node.js website (https://nodejs.org/). Once installed, you can verify the installations by running the following commands in your terminal:
bash node -v npm -v
3. Building Your First Command-Line Tool
3.1. Handling Command-Line Arguments
Command-line tools often require users to provide input arguments to customize their behavior. Node.js provides a built-in module called process that gives you access to the command-line arguments. Here’s a basic example of how to retrieve and use command-line arguments:
javascript // my-tool.js const args = process.argv.slice(2); // Remove the first two arguments (node executable and script file) if (args.length === 0) { console.log('Usage: node my-tool.js <name>'); } else { const name = args[0]; console.log(`Hello, ${name}!`); }
Run the tool using the following command:
bash node my-tool.js John
3.2. Creating User-Friendly Interfaces
While basic command-line tools can get the job done, creating a user-friendly experience is essential. The commander package is a popular choice for building command-line interfaces in Node.js. Install it using:
bash npm install commander
Here’s an example of using commander to enhance your command-line tool:
javascript // my-tool.js const { program } = require('commander'); program.version('1.0.0').description('A friendly command-line tool.'); program .option('-n, --name <name>', 'Your name') .parse(process.argv); const options = program.opts(); if (options.name) { console.log(`Hello, ${options.name}!`); } else { console.log('Usage: node my-tool.js -n <name>'); }
4. Adding Functionality: From Simple to Complex
4.1. File Manipulation and I/O Operations
Command-line tools often need to work with files and perform I/O operations. Node.js provides several built-in modules, such as fs (File System), that make working with files straightforward. Here’s an example of reading and displaying the contents of a file:
javascript // read-file.js const fs = require('fs'); fs.readFile('example.txt', 'utf8', (err, data) => { if (err) { console.error('Error reading file:', err); return; } console.log('File contents:', data); });
4.2. Networking and API Calls
Command-line tools can also interact with web services and APIs. The axios package is a popular choice for making HTTP requests in Node.js. Install it using:
bash npm install axios
Here’s an example of using axios to fetch data from an API:
javascript // fetch-data.js const axios = require('axios'); axios.get('https://jsonplaceholder.typicode.com/posts/1') .then(response => { console.log('Data:', response.data); }) .catch(error => { console.error('Error fetching data:', error); });
5. Structuring Your Command-Line Tool Project
5.1. Managing Dependencies
As your command-line tool grows in complexity, you might need to manage dependencies efficiently. Use a package.json file to list your project’s dependencies and their versions. You can create one by running:
bash npm init
Then, install and save dependencies using:
bash npm install dependency-name
5.2. Organizing Code Effectively
To maintain a clean and organized codebase, consider structuring your project into multiple files or modules. For instance, you can separate different functionalities into their own modules and then require them in your main script.
plaintext my-tool/ ??? my-tool.js (main script) ??? utils/ ? ??? file.js ? ??? network.js ??? package.json
6. Testing and Debugging
6.1. Writing Unit Tests
Testing is crucial to ensure your command-line tool behaves as expected. The jest framework is commonly used for testing in Node.js. Install it using:
bash npm install --save-dev jest
Create a test file, such as my-tool.test.js, to write tests for your tool’s functions and components:
javascript // my-tool.test.js const myTool = require('./my-tool'); test('Greeting message includes the name', () => { const greeting = myTool.generateGreeting('Alice'); expect(greeting).toContain('Alice'); });
Run tests using:
bash npx jest
6.2. Debugging Techniques
Debugging command-line tools can be challenging due to the absence of a graphical interface. However, you can use Node.js’s built-in debugging capabilities and tools like node-inspect to debug your code. Insert debugger statements in your code and run the tool with the following command:
bash node --inspect-brk my-tool.js
Then, open Chrome and navigate to chrome://inspect to start debugging.
7. Packaging and Distributing Your Tool
7.1. NPM Packages and Versioning
If you plan to share your command-line tool with others, packaging it as an npm package is a convenient approach. To publish your tool on npm, create an account on the npm website (https://www.npmjs.com/) and use the following commands:
bash npm login npm publish
Remember to follow semantic versioning (semver) for version numbers (e.g., 1.0.0).
7.2. Global Installation vs. Local Usage
Users can install your tool globally or use it locally within a project. Global installation allows users to access the tool from anywhere in their terminal:
bash npm install -g my-tool
Local usage involves installing the tool as a development dependency within a project:
bash npm install --save-dev my-tool
8. Best Practices for Command-Line Tool Development
8.1. User Experience and Documentation
Prioritize user experience by providing clear and concise usage instructions, error messages, and help messages. Include a –help option that displays information about how to use the tool.
8.2. Error Handling and Logging
Implement robust error handling to provide informative error messages when something goes wrong. Additionally, use logging to keep track of tool usage and potential issues.
Conclusion
Creating command-line tools with Node.js can significantly enhance your productivity and offer automation capabilities for various tasks. By following the steps outlined in this guide, you can build versatile and efficient tools that cater to your specific needs. Remember to focus on user experience, adhere to best practices, and continuously improve your tools as you gain more experience in command-line tool development. With Node.js, the possibilities are endless, and you have the power to create tools that streamline your workflow and make your development journey more enjoyable.
Whether you’re a system administrator, a data scientist, or a software engineer, mastering the art of command-line tool development will undoubtedly prove to be an invaluable skill in your arsenal.
Table of Contents
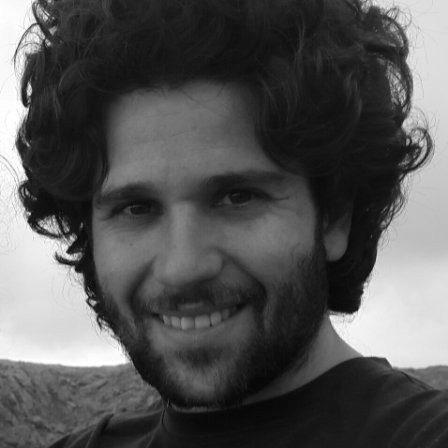
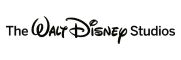