Unit Testing in Node.js: Tools and Best Practices
In the realm of modern software development, ensuring code quality and reliability is paramount. Unit testing is a cornerstone practice that helps developers achieve these goals by allowing them to test individual units of code in isolation. In this blog, we will delve into the world of unit testing in Node.js, exploring the tools and best practices that empower developers to build robust and error-free applications.
Table of Contents
1. Why Unit Testing Matters:
Unit testing involves testing individual units or components of code in isolation to ensure their correctness. These units can be functions, classes, or even smaller modules. The primary benefits of unit testing include:
- Early Detection of Bugs: Unit tests can catch bugs early in the development process, reducing the cost and effort of fixing them later.
- Code Documentation: Well-written unit tests act as documentation, providing examples of how functions or modules should be used.
- Code Refactoring: Unit tests provide confidence to refactor code without introducing new bugs, as long as the tests continue to pass.
- Improved Collaboration: When multiple developers work on a project, unit tests provide a common understanding of expected behavior.
2. Choosing a Testing Framework:
Node.js offers a variety of testing frameworks to choose from. Some of the most popular ones are:
2.1. Mocha:
Mocha is a versatile testing framework that supports both synchronous and asynchronous testing. It provides a clean and expressive syntax for writing tests and supports various assertion libraries such as Chai and Should.js.
2.2. Jest:
Jest is a popular testing framework developed by Facebook. It emphasizes simplicity while providing powerful features such as automatic mocking and parallel test execution.
2.3. Jasmine:
Jasmine is a behavior-driven testing framework that focuses on readability. It provides an expressive syntax for describing test cases and assertions.
3. Setting Up Your Testing Environment:
Before diving into writing tests, let’s ensure you have a proper testing environment set up.
3.1. Installing Dependencies:
To get started with unit testing in Node.js, you’ll need to install the necessary testing framework and assertion library. Here’s an example using Mocha and Chai:
bash npm install mocha chai --save-dev
3.2. Creating a Test Script:
In your project’s package.json file, you can add a test script that runs your unit tests. For Mocha, the script might look like this:
json "scripts": { "test": "mocha" }
Writing Your First Unit Test:
Now, let’s write a simple unit test using Mocha and Chai. Suppose you have a function called add that adds two numbers:
javascript // math.js function add(a, b) { return a + b; } module.exports = { add };
Create a corresponding test file:
javascript // test/math.test.js const chai = require('chai'); const math = require('../math'); const expect = chai.expect; describe('Math', () => { describe('add', () => { it('should return the sum of two numbers', () => { const result = math.add(2, 3); expect(result).to.equal(5); }); }); });
4. Best Practices for Effective Unit Testing:
4.1. Isolate Tests:
Ensure that each test is independent and doesn’t rely on the state or behavior of previous tests. Use tools like test doubles (mocks, stubs, spies) to isolate units of code.
4.2. Clear and Descriptive Test Names:
Write descriptive test names that clearly convey what aspect of the code is being tested. This enhances readability and understanding.
4.3. Arrange-Act-Assert (AAA) Pattern:
Structure your tests using the AAA pattern: Arrange the initial conditions, Act on the code, and Assert the expected outcome.
4.4. Use Mocks and Stubs Sparingly:
While mocks and stubs can be useful, overusing them can lead to tightly coupled tests that don’t accurately reflect how components interact.
4.5. Test Edge Cases:
Ensure your tests cover a wide range of inputs, including edge cases and invalid inputs.
4.6. Continuous Integration (CI):
Integrate your tests into your CI/CD pipeline to ensure that tests are run automatically whenever code is pushed or merged.
4.7. Test Coverage:
Aim for high test coverage to ensure that most of your code is being exercised by your tests.
Conclusion
Unit testing is an essential practice for ensuring the quality, reliability, and maintainability of Node.js applications. By adopting the right tools, such as Mocha, Chai, and other testing frameworks, and following best practices, developers can confidently write and maintain code that stands up to the rigors of real-world use. Whether you’re a seasoned developer or just starting out, embracing unit testing will empower you to create software that is robust, bug-free, and ready to take on the challenges of the ever-evolving software landscape.
Table of Contents
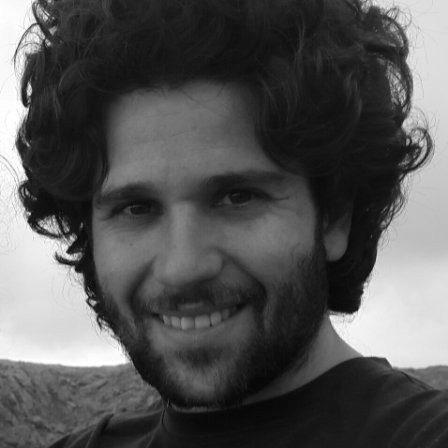
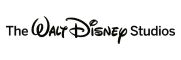