Exploring GraphQL in Node.js: Concepts and Implementation
In the realm of modern web development, GraphQL has emerged as a powerful alternative to the traditional REST API. It provides developers with a flexible and efficient way to request and manipulate data from servers. If you’re working with Node.js, integrating GraphQL into your applications can offer numerous benefits. In this blog post, we will delve into the concepts behind GraphQL and guide you through the process of implementing it in a Node.js environment.
Table of Contents
1. Understanding GraphQL
1.1. What is GraphQL?
GraphQL is an open-source query language developed by Facebook that allows clients to request exactly the data they need from a server. Unlike REST APIs, where data retrieval is determined by the server, GraphQL empowers the client to define the structure of the response. This reduces over-fetching and under-fetching of data, resulting in more efficient and optimized data transfers.
1.2. Advantages over REST
- Efficient Data Fetching: GraphQL minimizes the over-fetching problem encountered in REST APIs, ensuring that clients receive only the required data.
- Single Request: Clients can retrieve all the necessary data using a single GraphQL query, reducing the number of requests and responses.
- Flexibility: Clients can specify the structure and shape of the response, reducing the need for multiple endpoints.
- Strong Typing: GraphQL schemas are defined using a type system, providing clear expectations for the data.
- Introspection: GraphQL schemas can be introspected, allowing tools to generate documentation and perform validation.
2. Core Concepts of GraphQL
2.1. The Schema
The schema is at the heart of every GraphQL server. It defines the types of data that can be queried and the relationships between them. GraphQL schemas are defined using the Schema Definition Language (SDL), which provides a clear and concise syntax for describing types and their fields.
Here’s a simple example of a GraphQL schema:
graphql type Post { id: ID! title: String! body: String! } type Query { post(id: ID!): Post }
In this example, we’ve defined a Post type with fields id, title, and body, and a Query type with a single field post that takes an id argument and returns a Post.
2.2. Queries
Queries are used to request data from a GraphQL server. They mirror the structure of the schema and allow clients to specify which fields they want to retrieve.
graphql query { post(id: "123") { title body } }
In this query, we’re requesting the title and body fields of a post with the ID 123.
2.3. Mutations
Mutations are used to modify data on the server. They are similar in structure to queries but are used for operations that cause side effects, such as creating, updating, or deleting data.
graphql mutation { createPost(title: "New Post", body: "This is a new post.") { id title } }
This mutation creates a new post with the given title and body and returns the id and title of the newly created post.
2.4. Resolvers
Resolvers are functions responsible for fetching the data requested in a query or mutation. Each field in the schema has a corresponding resolver function that retrieves the data. Resolvers are executed in a specific order, starting from the root fields defined in the query.
Here’s a simple resolver for the post query:
javascript const resolvers = { Query: { post: (parent, args) => { // Logic to fetch and return the post with the provided ID }, }, };
3. Setting Up a GraphQL Server in Node.js
3.1. Initializing a Node.js Project
To get started with GraphQL in Node.js, ensure you have Node.js and npm (Node Package Manager) installed on your system. You can initialize a new Node.js project using the following commands:
bash mkdir graphql-nodejs-tutorial cd graphql-nodejs-tutorial npm init -y
3.2. Installing Dependencies
The most common library used to create a GraphQL server in Node.js is apollo-server. You can install it along with its required dependencies using the following command:
bash npm install apollo-server graphql
3.3. Creating the GraphQL Schema
Create a new file named schema.js in your project directory. This file will hold your GraphQL schema definition.
javascript const { gql } = require('apollo-server'); const typeDefs = gql` type Post { id: ID! title: String! body: String! } type Query { post(id: ID!): Post } `; module.exports = typeDefs;
3.4. Implementing Resolvers
Next, create a file named resolvers.js in the same directory as schema.js. This is where you’ll implement resolver functions for the fields in your schema.
javascript const resolvers = { Query: { post: (parent, args) => { // Logic to fetch and return the post with the provided ID }, }, }; module.exports = resolvers;
4. Writing Queries and Mutations
With the schema and resolvers in place, you can now start writing queries and mutations to interact with your GraphQL server.
4.1. Basic Queries
Open your terminal and navigate to your project directory. Start a Node.js REPL session by running the following command:
bash node
Inside the REPL, you can import the required libraries and set up the Apollo Server:
javascript const { ApolloServer } = require('apollo-server'); const typeDefs = require('./schema'); const resolvers = require('./resolvers'); const server = new ApolloServer({ typeDefs, resolvers }); server.listen().then(({ url }) => { console.log(`Server ready at ${url}`); });
Now you can query your server using GraphQL syntax:
graphql query { post(id: "123") { title body } }
4.2. Query with Arguments
Queries can take arguments to customize the data retrieval. Modify the post query in the schema to accept an argument:
javascript type Query { post(id: ID!): Post }
Now you can query a specific post using its ID:
graphql query { post(id: "123") { title body } }
4.3. Mutations for Data Modification
To implement mutations, start by extending your schema to include a mutation type and a createPost mutation:
javascript type Mutation { createPost(title: String!, body: String!): Post }
Now you can use the createPost mutation to add new posts:
graphql mutation { createPost(title: "New Post", body: "This is a new post.") { id title } }
5. Advanced GraphQL Features
5.1. Fragments
Fragments allow you to reuse parts of queries to keep them DRY (Don’t Repeat Yourself). Define a fragment and use it in multiple queries:
graphql fragment PostFields on Post { id title body } query { post(id: "123") { ...PostFields } }
5.2. Pagination
For queries that return a list of items, implement pagination using arguments like limit and offset:
graphql type Query { posts(limit: Int!, offset: Int!): [Post!]! } graphql Copy code query { posts(limit: 10, offset: 0) { ...PostFields } }
5.3. Authentication and Authorization
Integrate authentication and authorization by adding middleware to your resolver functions. You can also leverage third-party libraries for more advanced scenarios.
6. Testing and Debugging
6.1. Using GraphQL Playground
GraphQL Playground is a powerful tool for testing and debugging GraphQL queries and mutations. By default, Apollo Server provides a web-based interface for the playground at http://localhost:4000.
6.2. Error Handling and Debugging Tips
GraphQL provides detailed error responses with useful information to aid in debugging. Handle errors gracefully in your resolvers and ensure your server logs provide meaningful context.
7. Integrating GraphQL with Databases
7.1. Connecting to a Database
To integrate GraphQL with a database, you can use libraries like prisma, mongoose, or raw SQL queries. Connect your resolvers to your chosen database for fetching and modifying data.
7.2. Fetching and Modifying Data
Modify your resolvers to fetch and modify data from your database. Implement logic to handle data retrieval, creation, updating, and deletion.
8. Best Practices for GraphQL Development
8.1. Keep the Schema Simple
Design your schema with simplicity and reusability in mind. Avoid unnecessary complexity and nested types.
8.2. Caching Strategies
Leverage caching mechanisms to optimize query performance. Tools like Apollo Client offer built-in caching solutions.
8.3. Performance Considerations
Optimize your resolver functions and minimize the number of database queries. Use DataLoader to efficiently batch and cache database requests.
Conclusion
In conclusion, GraphQL offers an exciting and efficient approach to data fetching and manipulation in Node.js applications. Its flexible nature, strong typing, and ability to precisely request only the necessary data make it a compelling choice for modern web development. By grasping the core concepts and following the step-by-step implementation guide in this blog post, you’ll be well-equipped to integrate GraphQL into your Node.js projects and unlock its full potential. Happy coding!
Table of Contents
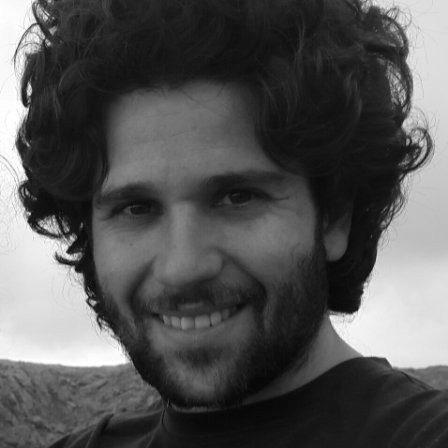
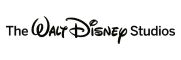