Data Streaming with Node.js: Techniques and Use Cases
In today’s data-driven landscape, the ability to process and analyze data in real time is crucial for making informed decisions and delivering responsive applications. This is where data streaming comes into play. Data streaming involves the continuous flow of data records, enabling organizations to process and act upon data as it arrives. In this blog post, we will delve into the realm of data streaming using Node.js, one of the most popular and versatile JavaScript runtime environments. We will explore various techniques, use cases, and provide code samples to demonstrate how Node.js can be harnessed for efficient data streaming.
Table of Contents
1. Introduction to Data Streaming
Data streaming involves the continuous transmission of data from one point to another, typically in the form of small, manageable chunks called data records. Unlike batch processing, where data is processed in large batches at once, data streaming processes data in real time as it becomes available. This approach offers numerous advantages, including quicker insights, faster response times, and the ability to react to changing conditions promptly.
2. Benefits of Data Streaming with Node.js
2.1. Real-time Processing
Node.js, known for its non-blocking, event-driven architecture, is well-suited for real-time data processing. Its event loop mechanism allows developers to handle multiple connections simultaneously without blocking the execution of other tasks. This makes Node.js an excellent choice for building applications that require instant processing of incoming data streams, such as live dashboards, real-time analytics, and more.
2.2. Scalability
Node.js’ lightweight nature and support for asynchronous operations make it highly scalable. As data streams grow in volume, Node.js can efficiently handle multiple streams concurrently. Moreover, Node.js’ built-in support for clustering enables developers to take full advantage of multi-core systems, further enhancing scalability for data-intensive applications.
2.3. Fault Tolerance
Building fault-tolerant data streaming applications is crucial to ensure data integrity and system reliability. Node.js, with its built-in mechanisms for handling errors and exceptions, aids in creating resilient data streaming pipelines. Additionally, various Node.js libraries and modules can be integrated to implement strategies like data replication, message acknowledgment, and automatic recovery in case of failures.
3. Techniques for Data Streaming with Node.js
3.1. Using Core Streams Module
Node.js provides a Core Streams module that offers a foundation for working with streams. Streams in Node.js can be categorized into four types: Readable, Writable, Duplex, and Transform. These streams enable developers to read, write, and manipulate data efficiently, making them essential for data streaming applications.
Here’s an example of creating a simple Readable stream in Node.js:
javascript const { Readable } = require('stream'); class MyReadableStream extends Readable { constructor(dataArray) { super({ objectMode: true }); this.dataArray = dataArray; this.currentIndex = 0; } _read() { if (this.currentIndex < this.dataArray.length) { this.push(this.dataArray[this.currentIndex]); this.currentIndex++; } else { this.push(null); } } } const data = ['Record 1', 'Record 2', 'Record 3']; const readableStream = new MyReadableStream(data); readableStream.on('data', (chunk) => { console.log('Received:', chunk); }); readableStream.on('end', () => { console.log('Stream ended.'); });
3.2. Implementing Kafka Streams
Kafka Streams is a powerful library for building applications and microservices that process and analyze data streams in real time. Apache Kafka, a distributed streaming platform, forms the backbone of Kafka Streams. Node.js can be used to interact with Kafka and implement real-time data processing pipelines.
To get started with Kafka Streams in Node.js, you’ll need to install the ‘kafkajs’ package:
bash npm install kafkajs
Here’s a basic example of using Kafka Streams with Node.js:
javascript const { Kafka } = require('kafkajs'); const kafka = new Kafka({ clientId: 'my-streaming-app', brokers: ['broker1:9092', 'broker2:9092'], }); const streamProcessing = async () => { const consumer = kafka.consumer({ groupId: 'my-group' }); await consumer.connect(); await consumer.subscribe({ topic: 'data-topic' }); await consumer.run({ eachMessage: async ({ topic, partition, message }) => { console.log({ key: message.key.toString(), value: message.value.toString(), }); }, }); }; streamProcessing().catch(console.error);
3. Use Cases of Data Streaming with Node.js
3.1. Real-time Analytics
Real-time analytics involves processing and analyzing data as it arrives to gain immediate insights. Node.js’ event-driven architecture and support for asynchronous programming make it a natural fit for real-time analytics applications. Whether it’s monitoring user activity on a website, tracking sensor data from IoT devices, or analyzing social media trends, Node.js can handle the continuous flow of data and provide real-time analytics dashboards for actionable insights.
3.2. Fraud Detection
Fraud detection systems rely on identifying anomalous patterns and behaviors in real time to prevent fraudulent activities. Node.js’ ability to process incoming data streams quickly can be leveraged to build fraud detection pipelines. By continuously analyzing transaction data, user behaviors, and other relevant information, Node.js-powered streaming applications can detect and respond to suspicious activities in real time, helping organizations mitigate financial losses and security breaches.
3.3. Social Media Monitoring
Social media monitoring involves tracking and analyzing mentions, hashtags, and sentiments across various social media platforms. With Node.js, developers can build streaming applications that aggregate and process social media data in real time. These applications can help businesses and marketing teams gauge public sentiment, track the performance of marketing campaigns, and respond promptly to emerging trends and discussions.
4. Getting Started: Code Samples
4.1. Setting Up a Basic Data Stream
To set up a basic data stream using Node.js, you can use the built-in Readable stream. Here’s a simple example that generates and emits random numbers as a data stream:
javascript const { Readable } = require('stream'); class NumberStream extends Readable { constructor(options) { super(options); this.max = options.max || 100; this.current = 1; } _read() { if (this.current > this.max) { this.push(null); return; } this.push(`${this.current}\n`); this.current++; } } const numberStream = new NumberStream({ max: 10 }); numberStream.pipe(process.stdout);
4.2. Creating a Simple Kafka Stream Processor
Using Kafka Streams with Node.js, you can process data from Kafka topics and perform transformations. Here’s a basic example of a Kafka stream processor that converts incoming messages to uppercase:
javascript const { Kafka } = require('kafkajs'); const kafka = new Kafka({ clientId: 'my-stream-processor', brokers: ['broker1:9092', 'broker2:9092'], }); const streamProcessing = async () => { const consumer = kafka.consumer({ groupId: 'my-group' }); const producer = kafka.producer(); await consumer.connect(); await producer.connect(); await consumer.subscribe({ topic: 'input-topic' }); await consumer.run({ eachMessage: async ({ topic, partition, message }) => { const originalValue = message.value.toString(); const transformedValue = originalValue.toUpperCase(); await producer.send({ topic: 'output-topic', messages: [{ value: transformedValue }], }); }, }); }; streamProcessing().catch(console.error);
5. Best Practices for Building Data Streaming Applications
5.1. Error Handling and Retry Mechanisms
Data streaming applications should be resilient to failures. Implement robust error handling and retry mechanisms to handle network issues, service interruptions, and other errors that can occur during data transmission. Consider implementing backoff strategies to prevent overwhelming downstream systems with retries.
5.2. Monitoring and Logging
Visibility into the health and performance of your data streaming application is crucial. Integrate logging and monitoring tools to track the flow of data, detect anomalies, and troubleshoot issues. Tools like Prometheus, Grafana, and application-specific logging can provide valuable insights into the behavior of your streaming pipelines.
5.3. Testing and Mocking Streams
Testing data streaming applications can be challenging due to their real-time nature. However, consider using techniques like mocking streams, simulating data events, and conducting end-to-end tests to ensure the correctness of your application. Libraries like stream-mock can help you create mock streams for testing purposes.
6. Future Trends in Data Streaming
As technology evolves, data streaming is expected to play an even more significant role in various industries. Machine learning integration, enhanced real-time analytics capabilities, and seamless integration with serverless architectures are some of the trends we can anticipate. Node.js will continue to be a vital tool for building data streaming applications, thanks to its performance, scalability, and vibrant ecosystem.
Conclusion
Data streaming with Node.js opens up a world of possibilities for building real-time applications that can process, analyze, and respond to data as it arrives. Whether you’re building real-time analytics platforms, fraud detection systems, or social media monitoring tools, Node.js provides the tools and capabilities to make it happen. By harnessing the power of data streaming, you can unlock insights, enhance user experiences, and drive innovation in the fast-paced digital landscape.
Table of Contents
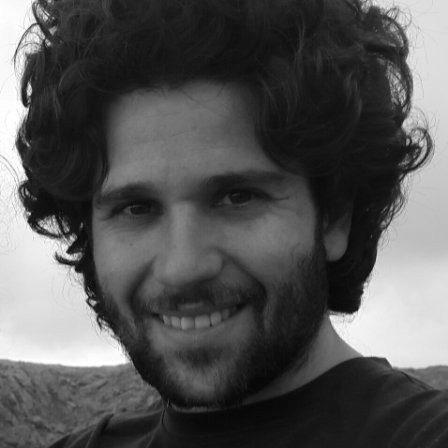
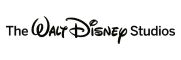