Debugging and Testing in Node.js: Tips and Tricks
As a Node.js developer, you’re likely familiar with the challenges of identifying and resolving bugs in your applications. Debugging and testing are essential aspects of the development process that help ensure the reliability and robustness of your code. In this blog, we’ll delve into the world of debugging and testing in Node.js, uncovering valuable tips and tricks that can significantly enhance your development workflow.
Table of Contents
1. Understanding the Importance of Debugging and Testing
Before we dive into the specifics, let’s briefly discuss why debugging and testing are crucial for Node.js applications. Debugging involves identifying and resolving issues in your code, ranging from syntax errors to logical flaws. On the other hand, testing involves systematically evaluating your application’s functionality to ensure it behaves as expected in different scenarios. These processes not only catch bugs early but also contribute to creating a stable and maintainable codebase.
2. Debugging in Node.js
Debugging in Node.js can be made efficient with the right tools and practices. Let’s explore some essential techniques:
2.1. Console.log() Statements
The simplest yet effective way to debug is by strategically placing console.log() statements in your code to track the flow of execution and variable values. While this approach is straightforward, it might become overwhelming in larger projects.
javascript function calculateTotal(price, quantity) { console.log("Calculating total..."); console.log(`Price: ${price}, Quantity: ${quantity}`); const total = price * quantity; console.log(`Total: ${total}`); return total; }
2.2. Node.js Debugger
Node.js provides a built-in debugger that allows you to set breakpoints, inspect variables, and step through your code. To use it, start your script with the –inspect flag and open Chrome DevTools.
bash node --inspect my-script.js
2.3. Debugging with VS Code
Visual Studio Code offers a powerful debugging environment for Node.js developers. Set breakpoints directly in the editor, inspect variables, and control the execution flow seamlessly.
2.4. Logging Libraries
Utilize logging libraries like winston or pino to create structured logs with various log levels. These libraries offer more control over what information is logged and allow you to analyze logs effectively.
javascript const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.File({ filename: 'app.log' }) ] }); logger.info('This is an informational log message.');
3. Testing in Node.js
Comprehensive testing ensures that your code functions as expected across different scenarios. Let’s explore testing techniques in Node.js:
3.1. Unit Testing with Mocha and Chai
Mocha is a popular testing framework that provides a flexible environment for writing test suites. Chai is an assertion library that works seamlessly with Mocha to make your tests more expressive.
Install Mocha and Chai using npm:
bash npm install mocha chai --save-dev
Example unit test using Mocha and Chai:
javascript const { expect } = require('chai'); const { calculateTotal } = require('./my-module'); describe('calculateTotal', () => { it('should calculate the total correctly', () => { const total = calculateTotal(10, 5); expect(total).to.equal(50); }); it('should handle zero quantity', () => { const total = calculateTotal(10, 0); expect(total).to.equal(0); }); });
3.2. Integration Testing
Integration tests focus on the interactions between different components of your application. Tools like Supertest can help you simulate HTTP requests and responses, allowing you to test your API endpoints thoroughly.
3.3. End-to-End Testing with Cypress
Cypress is an excellent tool for conducting end-to-end tests. It provides a real-time preview of your application as tests run, making it easier to identify issues visually.
4. Best Practices for Debugging and Testing
To maximize the effectiveness of your debugging and testing efforts, consider the following best practices:
4.1. Automate Tests
Set up automated testing pipelines using tools like Jenkins or GitHub Actions to ensure that tests are run consistently before deployment.
4.2. Mock Dependencies
When writing tests, use mock libraries like Sinon to simulate dependencies and external services. This keeps your tests isolated and focused on specific components.
4.3. Use Debugger-friendly Editors
IDEs and code editors like Visual Studio Code offer integrated debugging tools that streamline the debugging process. Familiarize yourself with these features to enhance your efficiency.
4.4. Continuous Integration and Continuous Deployment (CI/CD)
Implement CI/CD pipelines to automate the process of testing and deploying code changes. This approach ensures that code is thoroughly tested before reaching production.
Conclusion
Debugging and testing are not just tasks you perform as an afterthought; they are integral parts of the development lifecycle. With the right techniques and tools, you can uncover and eliminate bugs early, resulting in more reliable and efficient Node.js applications. By following the tips and tricks outlined in this blog, you’ll be better equipped to create code that stands up to real-world challenges and delivers a superior user experience. So, go ahead and embrace debugging and testing as your allies on the journey to becoming a Node.js development pro!
Table of Contents
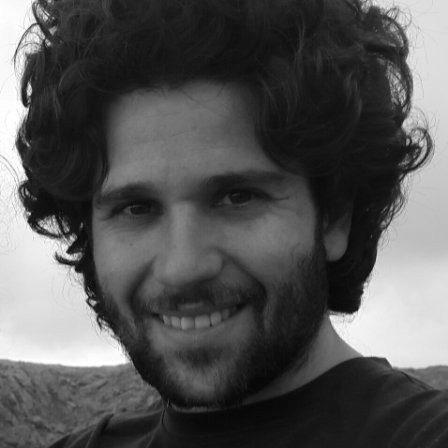
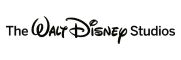