Exploring the Event Loop in Node.js
Node.js has gained immense popularity in the world of server-side programming due to its asynchronous and event-driven architecture. At the core of Node.js lies the Event Loop, a powerful mechanism that enables non-blocking I/O operations and efficient concurrency handling. Understanding the Event Loop is crucial for writing performant and scalable applications in Node.js. In this blog post, we’ll take a deep dive into the Event Loop, dissect its components, explore asynchronous programming, and provide practical code examples.
Table of Contents
1. Understanding Asynchronous Programming
1.1 Synchronous vs. Asynchronous
In synchronous programming, code execution occurs sequentially. Each operation must complete before the next one starts. Asynchronous programming, on the other hand, allows multiple operations to be executed concurrently, without waiting for each operation to finish before starting the next one. This is crucial for handling I/O-bound tasks efficiently, such as network requests or file system operations.
1.2 Benefits of Asynchronous Programming
Asynchronous programming provides several advantages, including improved application responsiveness, better resource utilization, and the ability to handle a large number of concurrent requests without significant performance degradation.
2. The Node.js Event Loop: An Overview
At the heart of Node.js is the Event Loop—a mechanism that enables asynchronous execution of code. The Event Loop continuously monitors the program’s execution context for tasks, processes them, and manages the order in which they’re executed. This architecture allows Node.js to handle multiple client requests simultaneously.
2.1 The Event Loop Lifecycle
The Event Loop follows a continuous cycle: It waits for events, processes the events in the order they’re received, and delegates tasks to appropriate handlers. The lifecycle involves several phases, including timers, I/O operations, and callbacks.
2.2 Phases of the Event Loop
The Event Loop consists of several phases, each representing a set of tasks to be processed. These phases include:
- Timers: Handle callbacks scheduled using functions like setTimeout().
- Pending I/O Callbacks: Execute I/O-related callbacks from completed asynchronous operations.
- Idle, Prepare: Used internally for optimizations.
- Poll: Retrieve new I/O events and process their callbacks.
- Check: Execute setImmediate() callbacks.
- Close Callbacks: Handle cleanup tasks for closed resources.
3. Callbacks and the Role of Callback Queue
Callbacks are the backbone of asynchronous programming in Node.js. When asynchronous operations complete, their corresponding callbacks are placed in the Callback Queue. The Event Loop picks up these callbacks during the “Poll” phase and executes them in the order they were added to the queue.
javascript // Example of a callback function const fetchData = (url, callback) => { // Simulating an asynchronous operation setTimeout(() => { const data = fetchFromUrl(url); callback(data); }, 1000); }; fetchData('https://example.com/data', (data) => { console.log('Fetched data:', data); });
4. Promises: A Higher-Level Abstraction
Promises provide a cleaner and more structured way to handle asynchronous operations. A Promise represents the eventual completion or failure of an asynchronous operation and allows you to chain actions in a readable manner.
javascript const fetchData = (url) => { return new Promise((resolve, reject) => { setTimeout(() => { const data = fetchFromUrl(url); if (data) { resolve(data); } else { reject(new Error('Failed to fetch data')); } }, 1000); }); }; fetchData('https://example.com/data') .then((data) => { console.log('Fetched data:', data); }) .catch((error) => { console.error('Error:', error.message); });
5. Async/Await: The Modern Approach
Async/Await is a syntactic improvement built on top of Promises, making asynchronous code look and feel more like synchronous code. It allows developers to write asynchronous code that is easier to read and understand.
javascript const fetchData = async (url) => { try { const data = await fetchFromUrl(url); return data; } catch (error) { throw new Error('Failed to fetch data'); } }; (async () => { try { const data = await fetchData('https://example.com/data'); console.log('Fetched data:', data); } catch (error) { console.error('Error:', error.message); } })();
6. Timers and the Event Loop
Timers, such as those created using setTimeout() and setInterval(), play a pivotal role in the Event Loop. These functions schedule callbacks to be executed after a specified amount of time.
javascript console.log('Start'); setTimeout(() => { console.log('Timeout callback executed'); }, 1000); console.log('End');
7. Event Emitters and Custom Events
Node.js provides the EventEmitter class, which enables you to create custom events and listeners. This is useful for building applications that involve event-driven architecture, such as real-time applications and APIs.
javascript const EventEmitter = require('events'); class MyEmitter extends EventEmitter {} const myEmitter = new MyEmitter(); myEmitter.on('customEvent', (message) => { console.log('Custom event:', message); }); myEmitter.emit('customEvent', 'Hello, World!');
8. Case Study: Building a Simple Asynchronous Application
Let’s build a basic example to showcase the power of asynchronous programming in Node.js. Imagine a scenario where we need to fetch data from multiple URLs concurrently.
javascript const fetch = require('node-fetch'); const urls = ['https://example.com/data1', 'https://example.com/data2', 'https://example.com/data3']; const fetchData = async (url) => { const response = await fetch(url); const data = await response.json(); return data; }; (async () => { const promises = urls.map((url) => fetchData(url)); const results = await Promise.all(promises); console.log('Fetched data:', results); })();
9. Concurrency and Thread Safety in Node.js
Node.js uses a single-threaded, event-driven architecture to handle asynchronous tasks efficiently. This eliminates the complexities of managing multiple threads and ensures thread safety. However, developers must be cautious when dealing with shared resources to prevent potential race conditions.
10. Best Practices for Event Loop Efficiency
- Utilize asynchronous patterns (Promises, Async/Await) for cleaner and more maintainable code.
- Avoid blocking the Event Loop with CPU-intensive operations; delegate them to worker threads or external services.
- Be mindful of callback hell by utilizing modularization and control flow libraries like async or bluebird.
- Use appropriate timeouts to prevent long-running asynchronous operations from causing bottlenecks.
Conclusion
Mastering the Event Loop in Node.js is essential for developing high-performance applications that can handle a large number of concurrent requests efficiently. With a solid understanding of asynchronous programming, the Event Loop, and modern patterns like Promises and Async/Await, you’re well-equipped to build robust and scalable Node.js applications.
In this blog post, we’ve covered the fundamental concepts behind the Event Loop, explored different asynchronous programming patterns, and provided practical code examples. Armed with this knowledge, you’re ready to embark on your journey to becoming a Node.js asynchronous programming expert. Happy coding!
Table of Contents
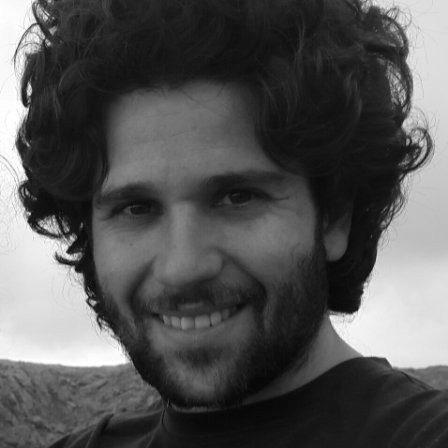
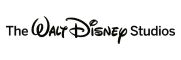