Exploring Node.js Frameworks: Hapi, Koa, and Sails
Node.js has revolutionized the way we build server-side applications with its non-blocking, event-driven architecture. To make the development process even smoother, various frameworks have emerged that provide structure, utilities, and abstractions for building robust web applications. In this article, we will dive into three popular Node.js frameworks: Hapi, Koa, and Sails. We will explore their features, use cases, and provide code samples to demonstrate their strengths.
Table of Contents
1. Introduction to Node.js Frameworks
Node.js frameworks are tools that simplify the process of building web applications by providing a structured foundation, predefined libraries, and conventions. These frameworks help developers focus on application logic rather than boilerplate code, speeding up development and enhancing maintainability.
2. Hapi: Emphasis on Configuration
2.1. Core Features of Hapi
Hapi, often pronounced as “happy,” is a powerful and flexible framework that emphasizes configuration-driven development. It offers a plethora of plugins and a modular architecture that allows developers to create custom configurations tailored to their project’s needs.
2.2. Hapi’s key features include:
- Configuration-Centric: Hapi’s philosophy centers around configuration-driven development, making it easier to manage complex applications.
- Plugin System: The framework offers a rich ecosystem of plugins that can be seamlessly integrated to add functionality without excessive coding.
- Input Validation: Hapi comes with built-in input validation and serialization, enhancing security and minimizing common vulnerabilities.
- Caching: It provides built-in support for caching strategies, helping to improve application performance.
- Authentication and Authorization: Hapi offers robust authentication and authorization mechanisms to secure your endpoints.
2.3. Use Cases for Hapi
Hapi is an excellent choice for projects that require extensive configuration and demand a high level of control over the application’s architecture. It is often chosen for large-scale applications, API development, and projects that prioritize security and performance.
2.4. Code Sample: Creating a Basic Hapi Server
javascript const Hapi = require('@hapi/hapi'); const init = async () => { const server = Hapi.server({ port: 3000, host: 'localhost' }); server.route({ method: 'GET', path: '/', handler: (request, h) => { return 'Hello, Hapi!'; } }); await server.start(); console.log('Server running on %s', server.info.uri); }; init();
3. Koa: Focus on Middleware
3.1. Key Features of Koa
Koa is a more lightweight and expressive framework compared to its predecessor, Express. It is designed around the concept of middleware, allowing developers to focus on composing functions that execute in a sequential manner. This approach enhances flexibility and makes it easier to manage asynchronous code.
3.2. Koa’s main features include:
- Async/Await Support: Koa takes full advantage of JavaScript’s async/await, making asynchronous code cleaner and more readable.
- Contextual Middleware: The context object in Koa holds the state and request information, simplifying communication between middleware functions.
- Error Handling: Koa provides a graceful error-handling mechanism using try/catch blocks within middleware.
- Lightweight Core: Koa’s core is minimalistic, but its extensible middleware system lets you add features as needed.
3.3. When to Use Koa
Koa is an excellent choice for projects that value a lightweight framework with a focus on flexibility. It is particularly well-suited for applications that require fine-grained control over middleware execution and asynchronous flows.
3.4. Code Sample: Implementing Middleware in Koa
javascript const Koa = require('koa'); const app = new Koa(); // Logger Middleware app.use(async (ctx, next) => { console.log('Request URL:', ctx.url); await next(); }); // Response Middleware app.use(async (ctx) => { ctx.body = 'Hello, Koa!'; }); app.listen(3000);
4. Sails: Real-time Web Applications
4.1. Highlights of Sails Framework
Sails is a full-featured MVC framework that emphasizes real-time functionality and rapid development. It brings the concepts of conventions and “scaffolding” to Node.js development, making it easy to build dynamic and interactive applications.
4.2. Key features of Sails include:
- Model-View-Controller (MVC) Structure: Sails enforces an MVC architecture, making it easier to organize and maintain code.
- Real-time WebSockets: Sails comes with built-in support for WebSockets, making real-time communication between clients and the server seamless.
- Blueprint API: Sails provides a powerful blueprint API for automatically generating routes and CRUD operations.
- Data-Driven APIs: With Sails, you can quickly create APIs based on your data models, reducing manual coding.
4.3. Ideal Scenarios for Sails
Sails is an excellent choice for projects that require real-time features, such as chat applications, collaborative tools, and interactive dashboards. Its convention-over-configuration approach is beneficial for teams that want to follow established patterns and minimize decision-making overhead.
4.4. Code Example: Building a Real-time App with Sails
javascript const sails = require('sails'); sails.lift({ port: 3000, // Other configuration options }, (err) => { if (err) { console.error(err); return; } console.log('Sails app lifted'); });
5. Choosing the Right Framework
When selecting a Node.js framework, consider the following factors:
- Project Requirements: Choose a framework that aligns with your project’s needs, whether it’s real-time functionality, lightweight architecture, or configuration control.
- Team Expertise: Consider your team’s familiarity with the framework and its ecosystem. A framework your team is comfortable with will boost productivity.
- Community and Documentation: A strong community and comprehensive documentation are essential for getting help, finding plugins, and resolving issues.
Conclusion
Node.js frameworks offer a wide range of options for building web applications, each catering to different preferences and project requirements. Hapi, Koa, and Sails showcase distinct approaches to development, from configuration-driven flexibility to middleware-focused simplicity and real-time interactivity. By understanding their strengths and use cases, you can make an informed decision when choosing the best framework for your next Node.js project. Happy coding!
Remember, the key to successful framework selection is aligning the framework’s strengths with your project’s goals and your team’s expertise. Whether you prioritize configurability, lightweight middleware, or real-time functionality, these Node.js frameworks have you covered. Choose wisely and enjoy smoother development and more robust applications.
Table of Contents
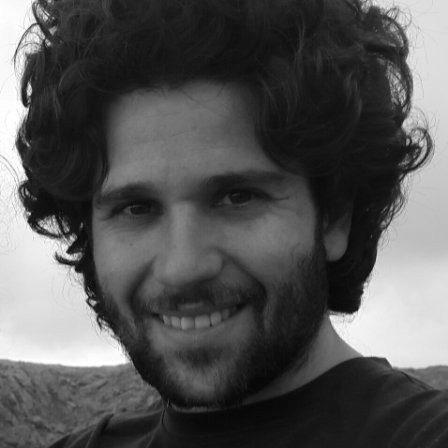
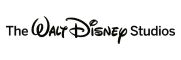