Building Microservices with Node.js and Docker
Microservices architecture has revolutionized the way we build and deploy applications. Instead of building monolithic applications, developers now opt for a modular approach where each component or service focuses on a specific task. This leads to better scalability, maintainability, and flexibility. In this guide, we’ll explore how to build microservices using Node.js and Docker, two powerful technologies that complement each other perfectly.
Table of Contents
1. Introduction to Microservices Architecture
Microservices architecture is an architectural style where a large application is broken down into smaller, loosely coupled services that can be developed, deployed, and scaled independently. Each microservice is responsible for a specific business capability and communicates with other microservices through APIs.
2. Benefits of Microservices Architecture
- Scalability: With microservices, you can scale individual services independently based on their workload. This leads to efficient resource utilization and cost savings.
- Flexibility: Microservices enable you to use different technologies for different services. This flexibility is especially helpful when dealing with diverse requirements.
- Maintainability: Since each microservice is self-contained, changes and updates can be made to one service without affecting others. This simplifies maintenance and reduces downtime.
- Faster Development: Smaller teams can work on individual microservices simultaneously, speeding up the development process.
3. Getting Started with Node.js and Docker
3.1. Setting Up the Development Environment
Before we dive into building microservices, let’s set up our development environment. We’ll need Node.js for writing our services and Docker for containerization.
- Install Node.js: Download and install Node.js from the official website (https://nodejs.org/). Node.js will allow us to write server-side JavaScript code.
- Install Docker: Install Docker from the Docker website (https://www.docker.com/). Docker will help us package our microservices into containers.
3.2. Creating Your First Microservice with Node.js
Let’s start by creating a basic microservice using Node.js. We’ll build a simple “Hello World” service that listens on a specific port and responds with a greeting.
Initialize a New Node.js Project: Open your terminal and navigate to your desired project directory. Run the following commands:
bash mkdir hello-microservice cd hello-microservice npm init -y
Install Dependencies: For our microservice, we’ll use the Express.js framework. Install it using the following command:
bash npm install express
Create the Microservice Code: Create a file named app.js and add the following code:
javascript const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello from the Microservice!'); }); app.listen(port, () => { console.log(`Microservice listening at http://localhost:${port}`); });
Run the Microservice: In your terminal, run the following command to start the microservice:
bash node app.js
3.3. Containerizing the Microservice with Docker
Now that we have a basic microservice, let’s containerize it using Docker. Containerization ensures that the microservice and its dependencies are packaged together, making deployment consistent across different environments.
Create a Dockerfile: In your project directory, create a file named Dockerfile (without any file extensions) and add the following lines:
Dockerfile # Use the official Node.js image as the base image FROM node:14 # Set the working directory inside the container WORKDIR /usr/src/app # Copy package.json and package-lock.json to the container COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code COPY . . # Expose the port the microservice will run on EXPOSE 3000 # Command to run the microservice CMD ["node", "app.js"]
Build the Docker Image: In the terminal, navigate to your project directory and run the following command to build the Docker image:
bash docker build -t hello-microservice .
Run the Docker Container: Once the image is built, you can run a Docker container based on it:
bash docker run -p 3000:3000 hello-microservice
4. Creating More Microservices and Communication
Building a single microservice is just the beginning. In a real-world scenario, you’ll have multiple microservices interacting with each other. Let’s explore how to create and communicate between multiple microservices.
4.1. Creating a Second Microservice
Let’s create another microservice that communicates with our existing “Hello World” service. This new microservice will act as a basic user management system.
Set Up the New Microservice Project: Create a new directory for the user management microservice and set up the project similar to what we did earlier.
Install Dependencies: Again, install Express.js as a dependency:
bash npm install express
Create the User Management Code: In the new microservice directory, create a file named user-service.js and add the following code:
javascript const express = require('express'); const app = express(); const port = 4000; app.get('/users', (req, res) => { // In a real scenario, fetch user data from a database const users = [ { id: 1, name: 'User 1' }, { id: 2, name: 'User 2' }, ]; res.json(users); }); app.listen(port, () => { console.log(`User Management Microservice listening at http://localhost:${port}`); });
Update Dockerfile: In the new microservice’s directory, create a Dockerfile just like before, and don’t forget to change the port to 4000 in the EXPOSE and CMD lines.
Build and Run the New Microservice: Build the Docker image and run the container as we did previously.
4.2. Communicating Between Microservices
Now that we have two microservices running, let’s make them communicate. Our “Hello World” service can greet users, and the user management service can provide user data. Let’s modify the “Hello World” service to fetch and display user data from the user management service.
Modify the “Hello World” Service: Update the app.js file of the “Hello World” service to make an HTTP request to the user management service:
javascript const express = require('express'); const axios = require('axios'); // Add this line const app = express(); const port = 3000; app.get('/', async (req, res) => { // Make a request to the user management service try { const response = await axios.get('http://user-service:4000/users'); // Notice the URL const users = response.data; const greeting = 'Hello from the Microservice! Users: ' + users.map(user => user.name).join(', '); res.send(greeting); } catch (error) { res.status(500).send('Error fetching user data'); } }); app.listen(port, () => { console.log(`Microservice listening at http://localhost:${port}`); });
Update Docker Compose: To manage the communication between microservices, let’s use Docker Compose. Create a docker-compose.yml file in your project directory and add the following content:
yaml version: '3' services: hello-microservice: build: context: . dockerfile: Dockerfile ports: - '3000:3000' user-service: build: context: ./user-service dockerfile: Dockerfile ports: - '4000:4000'
Run Docker Compose: In the terminal, navigate to your project directory and run the following command to start both microservices using Docker Compose:
bash docker-compose up
Conclusion
Building microservices with Node.js and Docker opens up a world of possibilities for creating scalable and maintainable applications. We’ve just scratched the surface of what’s possible in this guide. As you continue your microservices journey, you can explore advanced topics like service discovery, load balancing, and orchestrating containers using tools like Kubernetes. By combining the power of Node.js and Docker, you’re equipped to create robust and efficient microservices architectures that can handle the complexities of modern software development.
In this comprehensive guide, we’ve covered the basics of microservices architecture, setting up a development environment with Node.js and Docker, creating and containerizing microservices, and enabling communication between microservices. Remember that building microservices is an ongoing process that involves continuous learning and refinement. With the knowledge gained from this guide, you’re well on your way to architecting successful microservices-based applications.
Table of Contents
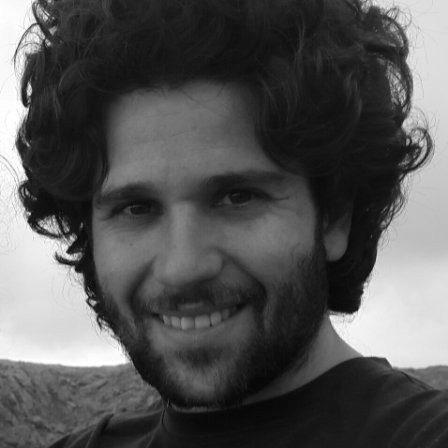
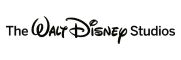