Node.js Performance Optimization: Techniques and Tools
In today’s fast-paced digital landscape, performance optimization is a crucial aspect of any successful application. Node.js, a powerful runtime environment that executes JavaScript code on the server-side, has gained immense popularity due to its efficiency and scalability. However, like any technology, Node.js applications can also suffer from performance bottlenecks that impact user experience and business outcomes. In this article, we will delve into various techniques and tools that can help you optimize the performance of your Node.js applications and ensure they run at their best.
Table of Contents
1. Understanding the Importance of Node.js Performance
Before diving into optimization techniques, it’s essential to understand why Node.js performance matters. In an era where users demand lightning-fast applications, slow-loading pages can lead to frustrated users and increased bounce rates. Moreover, search engines consider page speed as a ranking factor, making performance optimization crucial for SEO as well.
2. The Event-Driven, Non-Blocking Nature of Node.js
Node.js is built on an event-driven, non-blocking architecture, making it highly efficient for handling concurrent connections. However, improper handling of asynchronous operations can lead to performance issues. Let’s explore some techniques to enhance Node.js performance.
2.1. Efficient Asynchronous Programming
Asynchronous programming is at the core of Node.js, allowing applications to handle multiple tasks concurrently. However, incorrect use of callbacks or promises can result in callback hell or the infamous “pyramid of doom.” This not only makes the code hard to read and maintain but can also impact performance.
Solution: Utilize Async/Await
The introduction of async/await in ECMAScript simplifies asynchronous code, making it look more like synchronous code. This readability improvement enhances collaboration among team members and helps prevent performance bottlenecks caused by convoluted code.
javascript async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return data; } catch (error) { console.error('Error fetching data:', error); throw error; } }
2.2. Scalable Architecture
A well-designed architecture forms the foundation of a high-performance Node.js application. Implementing a scalable architecture allows your application to handle increased load without sacrificing performance.
Microservices Architecture
Breaking down your application into microservices enables independent scaling of components, reducing the risk of a single point of failure. This approach enhances resource utilization and optimizes performance.
2.3. Caching for Performance
Caching involves storing frequently accessed data in memory for quick retrieval. This technique significantly reduces the time and resources required to fetch data from databases or external APIs.
In-Memory Caching with Redis
Redis is an open-source, in-memory data structure store that can serve as a cache. It excels at storing and retrieving data rapidly, making it an excellent choice for caching in Node.js applications.
javascript const redis = require('redis'); const client = redis.createClient(); function getCachedData(key) { return new Promise((resolve, reject) => { client.get(key, (error, data) => { if (error) { reject(error); } else { resolve(data); } }); }); }
2.4. Load Balancing
Load balancing involves distributing incoming traffic across multiple servers to prevent overload on a single server. This technique improves response times and enhances fault tolerance.
Using the Cluster Module
Node.js provides the built-in cluster module, allowing you to create multiple child processes (workers) to handle incoming requests. This enables better utilization of available CPU cores and enhanced application performance.
javascript const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { for (let i = 0; i < numCPUs; i++) { cluster.fork(); } } else { http.createServer((req, res) => { res.writeHead(200); res.end('Hello, Node.js!'); }).listen(8000); }
2.5. Profiling and Monitoring
Continuous monitoring and profiling of your Node.js application are essential to identify performance bottlenecks and areas for improvement.
Using Node.js Profiler
Node.js comes with a built-in profiler that allows you to collect CPU and memory usage data. Analyzing this data helps pinpoint performance issues and optimize critical parts of your code.
bash node --prof your-app.js node --prof-process isolate-0xnnnnnnnnnnnn-v8.log > processed.txt
2.6. Performance Testing
Regular performance testing simulates various user scenarios to gauge how your application performs under different conditions. This step is crucial to identifying bottlenecks and ensuring your optimizations are effective.
Load Testing with Artillery
Artillery is a powerful load testing tool that allows you to simulate user traffic and measure your application’s response times. Writing test scenarios in YAML makes it easy to define complex testing scenarios.
yaml config: target: 'https://your-app.com' scenarios: - flow: - get: url: '/endpoint'
Conclusion
Optimizing the performance of your Node.js applications is a continuous process that requires vigilance and the right tools. By understanding the event-driven nature of Node.js, adopting efficient asynchronous programming techniques, implementing a scalable architecture, and utilizing tools like caching, load balancing, profiling, and performance testing, you can ensure that your applications deliver optimal speed and responsiveness.
Remember, every application is unique, and performance optimization may require a tailored approach. Regular monitoring, analysis of metrics, and a commitment to ongoing improvement will help you keep your Node.js applications running smoothly and efficiently in the ever-evolving digital landscape.
Table of Contents
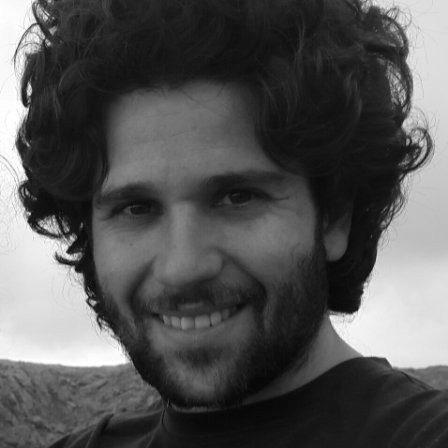
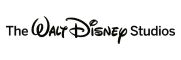