Building Real-Time Chat Applications with Node.js and WebSocket
In today’s fast-paced digital world, instant communication is a necessity. Real-time chat applications have become a cornerstone of modern online interactions, enabling seamless conversations and collaboration across various platforms. To achieve this, developers often turn to technologies like Node.js and WebSockets. In this tutorial, we’ll explore how to build real-time chat applications using Node.js and WebSocket, empowering you to create engaging, dynamic, and responsive chat platforms.
Table of Contents
1. Introduction to Real-Time Chat Applications
1.1. Understanding the Importance of Real-Time Communication
Real-time communication is the foundation of instant messaging, collaborative tools, and social media platforms. Traditional HTTP requests, while effective for many purposes, lack the responsiveness required for dynamic conversations. Imagine waiting for a page to refresh every time a new message arrives in a chat. Real-time chat applications solve this problem by enabling immediate message delivery and display, providing a more fluid and engaging user experience.
1.2. How WebSockets Revolutionize Chat Applications
WebSockets are a key technology for building real-time applications. Unlike traditional HTTP connections, which are stateless and require repeated requests, WebSockets allow for persistent, bidirectional communication channels between clients (usually browsers) and servers. This means that once a WebSocket connection is established, the server can send data to clients, and vice versa, without the need to continually request information.
2. Setting Up the Development Environment
2.1. Installing Node.js and npm
Before we dive into building our real-time chat application, make sure you have Node.js and npm (Node Package Manager) installed on your system. You can download them from the official Node.js website. Once installed, you can verify the installation by running the following commands in your terminal:
bash node -v npm -v
2.2. Creating a New Node.js Project
To start a new Node.js project, create a new directory for your project and navigate to it in your terminal:
bash mkdir real-time-chat-app cd real-time-chat-app
Next, initialize a new Node.js project by running:
bash npm init -y
This command creates a package.json file that will track your project’s dependencies and configuration.
2.3. Adding Dependencies for WebSocket Functionality
To implement WebSocket functionality in our project, we’ll use the popular ws library. It provides an easy way to set up WebSocket servers and clients. Install it using npm:
bash npm install ws
With the necessary dependencies in place, we can now move on to implementing WebSocket communication.
3. Implementing WebSocket Communication
3.1. Creating a WebSocket Server
In your project directory, create a new file named server.js. This file will contain the code for setting up our WebSocket server. Here’s a basic structure to get you started:
javascript const WebSocket = require('ws'); // Create a WebSocket server instance const server = new WebSocket.Server({ port: 3000 }); // Event listener for handling incoming WebSocket connections server.on('connection', (socket) => { // Handle the connection });
In this code snippet, we import the ws library and create a new WebSocket server instance on port 3000. We also set up an event listener for the ‘connection’ event, which fires whenever a new WebSocket connection is established.
3.2. Handling WebSocket Connections
Inside the ‘connection’ event listener, we can handle the WebSocket connections. We’ll use the socket object to interact with individual clients. Here’s how you can send and receive messages:
javascript server.on('connection', (socket) => { console.log('Client connected'); // Event listener for handling messages from clients socket.on('message', (message) => { console.log(`Received: ${message}`); // Sending a message back to the client socket.send(`You sent: ${message}`); }); });
In this example, whenever a client sends a message, the server receives it and sends a response back to that specific client.
3.3. Broadcasting Messages to All Connected Clients
A key feature of chat applications is the ability to broadcast messages to all connected clients. To achieve this, we’ll maintain an array of connected sockets and loop through them to send messages to everyone. Modify your code as follows:
javascript const connectedSockets = []; server.on('connection', (socket) => { connectedSockets.push(socket); socket.on('message', (message) => { console.log(`Received: ${message}`); // Broadcast the message to all connected clients connectedSockets.forEach((clientSocket) => { if (clientSocket !== socket) { clientSocket.send(`Someone said: ${message}`); } }); }); });
With this addition, whenever a client sends a message, the server will broadcast it to all other connected clients except the sender.
4. Building the Frontend
4.1. Designing the Chat Interface with HTML and CSS
Before we connect our frontend to the WebSocket server, let’s create a simple chat interface using HTML and CSS. Create an index.html file in your project directory and add the following code:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Real-Time Chat</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="chat-container"> <div class="chat-messages"></div> <input type="text" id="messageInput" placeholder="Type your message..."> <button id="sendButton">Send</button> </div> <script src="client.js"></script> </body> </html>
Create a styles.css file in the same directory and add some basic styling to structure the chat interface:
css /* Add your CSS styling here */
4.2. Establishing WebSocket Connection from the Browser
To connect the frontend to the WebSocket server, create a client.js file in your project directory. In this file, we’ll establish a WebSocket connection and handle incoming and outgoing messages:
javascript const socket = new WebSocket('ws://localhost:3000'); socket.addEventListener('open', (event) => { console.log('Connected to WebSocket server'); }); socket.addEventListener('message', (event) => { const message = event.data; // Display the message in the chat interface }); // Get DOM elements const messageInput = document.getElementById('messageInput'); const sendButton = document.getElementById('sendButton'); // Send a message when the send button is clicked sendButton.addEventListener('click', () => { const message = messageInput.value; if (message) { // Send the message through the WebSocket connection socket.send(message); messageInput.value = ''; } });
In this code snippet, we create a WebSocket connection to the server running on ws://localhost:3000. We listen for the ‘open’ event to confirm the connection and the ‘message’ event to handle incoming messages. The user’s input is sent as a message when they click the send button.
4.3. Handling Incoming and Outgoing Messages
To display incoming messages and clear the input field after sending a message, update the ‘message’ event listener in client.js:
javascript socket.addEventListener('message', (event) => { const message = event.data; const chatMessages = document.querySelector('.chat-messages'); const messageElement = document.createElement('div'); messageElement.textContent = message; chatMessages.appendChild(messageElement); chatMessages.scrollTop = chatMessages.scrollHeight; }); // ... sendButton.addEventListener('click', () => { const message = messageInput.value; if (message) { socket.send(message); messageInput.value = ''; } });
With this code, incoming messages will be added to the chat interface, and the chat window will automatically scroll to show the latest messages.
5. Enhancing the Chat Experience
5.1. User Authentication and Identification
For a more comprehensive chat application, you can implement user authentication and identification. This can be done using various techniques, such as integrating with third-party authentication providers or using JWT (JSON Web Tokens) for token-based authentication.
5.2. Typing Indicators and Read Receipts
To enhance the user experience, you can implement typing indicators and read receipts. Typing indicators inform users when someone is currently typing a message, and read receipts indicate when a message has been read by the recipient. This involves adding event listeners to track user keystrokes and message read status.
5.3. Storing Chat History and Data Persistence
To maintain a history of chat messages and ensure data persistence, you can integrate a database such as MongoDB or PostgreSQL. Storing chat messages allows users to view previous conversations even after logging out and logging back in.
6. Deploying the Application
6.1. Choosing a Hosting Provider
Before deploying your real-time chat application, you need to choose a hosting provider. Options like Heroku, DigitalOcean, and AWS offer easy deployment solutions for Node.js applications.
6.2. Configuring Production Environment
When deploying to a production environment, make sure to configure environment variables, security settings, and performance optimizations. Use tools like Nginx as a reverse proxy and set up HTTPS for secure communication.
6.3. Launching Your Real-Time Chat Application
Once you’ve configured your production environment, deploy your application using the chosen hosting provider’s documentation. This typically involves pushing your code to a remote repository, setting up the necessary server configurations, and starting the application on the server.
Congratulations! You’ve successfully built a real-time chat application using Node.js and WebSockets. Your users can now enjoy instant and dynamic communication on your platform.
Conclusion
Real-time chat applications have transformed the way we communicate online. By harnessing the power of Node.js and WebSockets, developers can create engaging and responsive chat platforms that facilitate instant conversations. In this tutorial, we covered the importance of real-time communication, the role of WebSockets, setting up the development environment, implementing WebSocket communication, building the frontend, enhancing the chat experience, and deploying the application to a production environment. With these skills, you’re well-equipped to create your own real-time chat applications and contribute to the world of dynamic online interactions.
Table of Contents
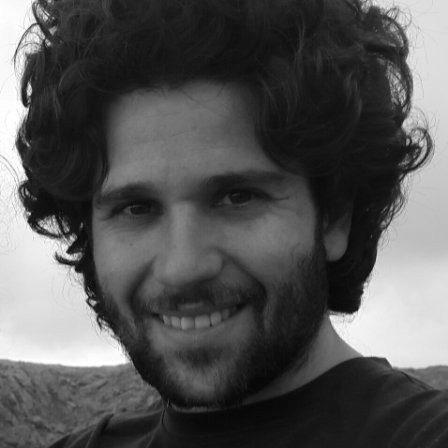
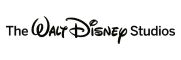