Scaling Node.js Applications: Strategies and Approaches
In today’s fast-paced digital landscape, the success of an application often hinges on its ability to seamlessly handle growing user demands. Node.js has gained popularity due to its non-blocking, event-driven architecture, making it a popular choice for building scalable applications. However, as your application gains more users and traffic, it’s crucial to have a solid plan for scaling to maintain performance and reliability. In this article, we will explore strategies and approaches for effectively scaling your Node.js applications.
Table of Contents
1. Understanding the Need for Scaling
As your application gains traction and attracts more users, the demand on your server resources increases. Without proper scaling, you risk encountering slow response times, downtime, and an overall poor user experience. Scaling involves expanding your application’s capacity to handle increased traffic and ensuring that the user experience remains smooth.
2. Horizontal vs. Vertical Scaling
Scaling can be approached in two main ways: horizontal and vertical scaling.
2.1. Horizontal Scaling
Horizontal scaling involves adding more machines to your infrastructure to distribute the incoming traffic. This approach can be achieved through load balancing, where incoming requests are evenly distributed among multiple servers. Node.js applications can greatly benefit from horizontal scaling due to their event-driven nature, which makes it easier to distribute tasks across different instances.
Here’s a basic example of implementing horizontal scaling using the cluster module in Node.js:
javascript const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { // Fork workers for each CPU for (let i = 0; i < numCPUs; i++) { cluster.fork(); } } else { // Workers handle incoming requests http.createServer((req, res) => { res.writeHead(200); res.end('Hello, Node.js scaling!'); }).listen(8000); }
2.2. Vertical Scaling
Vertical scaling involves upgrading the resources of a single machine, such as increasing the CPU, RAM, or storage. While this approach can provide an immediate boost in performance, it might have limitations in the long run. Node.js applications, being single-threaded, can face limitations in utilizing enhanced resources effectively.
3. Load Balancing
Load balancing is a key technique for distributing incoming traffic across multiple servers, ensuring even resource utilization and preventing any single server from becoming a bottleneck. There are several ways to implement load balancing, including:
3.1. Nginx as a Load Balancer
Nginx can act as a reverse proxy server, distributing incoming requests to multiple Node.js instances. Here’s a simplified configuration snippet for Nginx:
nginx http { upstream nodejs_servers { server 127.0.0.1:8000; server 127.0.0.1:8001; # Add more server entries as needed } server { listen 80; server_name yourdomain.com; location / { proxy_pass http://nodejs_servers; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; } } }
3.2. Using a Load Balancer Module
Node.js provides modules like http-proxy that allow you to create a custom load balancer within your application. Here’s a basic example using the http-proxy module:
javascript const http = require('http'); const httpProxy = require('http-proxy'); const proxy = httpProxy.createProxyServer(); const server = http.createServer((req, res) => { // Perform any pre-processing if needed proxy.web(req, res, { target: 'http://localhost:8000', // Point to your Node.js instances }); }); server.listen(80);
Load balancing ensures efficient resource utilization and better fault tolerance, enhancing the scalability of your Node.js application.
4. Caching for Performance
Caching can significantly improve the performance of your application by storing frequently accessed data in memory. Node.js applications can utilize caching to reduce the load on the database and decrease response times. Two common types of caching are:
4.1. In-Memory Caching with Redis
Redis is an in-memory data store that can serve as a cache for frequently used data. It’s highly efficient and can significantly reduce the load on your main database. Here’s an example of using Redis for caching in a Node.js application:
javascript const redis = require('redis'); const client = redis.createClient(); app.get('/user/:id', (req, res) => { const userId = req.params.id; // Check if data is cached client.get(userId, (err, cachedData) => { if (cachedData) { res.json(JSON.parse(cachedData)); } else { // Fetch data from the main database const userData = fetchUserDataFromDatabase(userId); // Cache the data for future requests client.setex(userId, 3600, JSON.stringify(userData)); // Cache for an hour res.json(userData); } }); });
4.2. Content Delivery Networks (CDNs)
CDNs distribute static assets like images, CSS files, and JavaScript libraries to servers located geographically closer to the user. This reduces the latency and load on your main server, improving the overall application performance.
5. Microservices Architecture
Microservices architecture involves breaking down your application into smaller, independent services that can be developed, deployed, and scaled individually. This approach promotes flexibility, scalability, and easier maintenance. Node.js is well-suited for microservices due to its lightweight nature and event-driven model.
Each microservice can have its own database, API, and functionality. Communication between microservices can be established through APIs or messaging systems like RabbitMQ or Kafka.
6. Monitoring and Auto-scaling
Continuous monitoring of your application’s performance is crucial for identifying bottlenecks and making informed scaling decisions. Tools like New Relic, Datadog, and Prometheus can help you collect and analyze performance data. Additionally, setting up auto-scaling rules allows your infrastructure to automatically adjust the number of instances based on predefined metrics such as CPU usage or response time.
Conclusion
Scaling Node.js applications is a critical aspect of ensuring their performance, reliability, and user experience as traffic grows. By understanding the differences between horizontal and vertical scaling, implementing effective load balancing strategies, leveraging caching techniques, adopting a microservices architecture, and utilizing monitoring and auto-scaling tools, you can confidently tackle the challenges of scaling and take your Node.js applications to new heights of success. Remember, every application is unique, so it’s essential to evaluate your specific requirements and choose the scaling strategies that best fit your needs.
Table of Contents
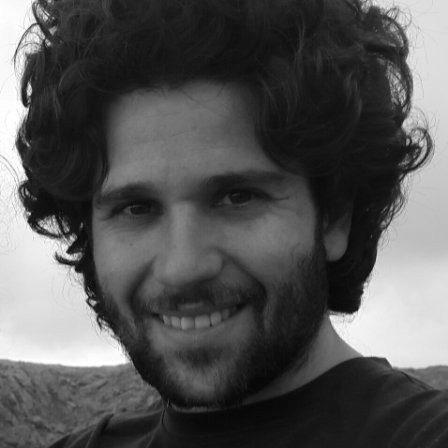
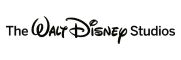