Node.js Security Best Practices: Protecting Your Applications
In today’s digital landscape, where the majority of applications are connected to the internet, security has become an indispensable aspect of software development. Node.js, a powerful and widely-used JavaScript runtime, enables developers to build efficient and scalable server-side applications. However, like any technology, Node.js applications are not immune to security vulnerabilities. This blog post outlines essential Node.js security best practices that every developer should follow to safeguard their applications against potential threats and attacks.
Table of Contents
1. Introduction
In the digital era, where cyber threats are ever-evolving, it’s crucial to prioritize security in your Node.js applications. Regardless of whether you’re developing a small web application or a large-scale API service, neglecting security measures can have dire consequences, including data breaches, unauthorized access, and service disruptions. By adhering to the Node.js security best practices outlined below, you can significantly reduce the risk of such threats and build applications that users can trust.
2. Keep Dependencies Up to Date
One of the most common entry points for attackers is through outdated dependencies. As Node.js applications rely on numerous third-party packages, it’s imperative to keep them updated to avoid using versions with known vulnerabilities.
2.1 Regularly Update Packages
Regularly update your application’s dependencies to include the latest security patches. You can use the following commands to check for outdated packages and update them:
bash # Check for outdated packages npm outdated # Update a specific package npm update package-name # Update all packages npm update
2.2 Use Package-Locking
Utilize a package-lock.json or npm-shrinkwrap.json file to lock down the versions of your dependencies. This ensures that your application uses the same versions of packages across different environments and prevents unintended updates.
3. Input Validation and Sanitization
User input is a common vector for attacks like Cross-Site Scripting (XSS) and SQL injection. Properly validating and sanitizing user input can mitigate these risks.
3.1 Validate User Input
Always validate user input on both the client and server sides. Utilize validation libraries like validator to ensure that input adheres to expected formats.
javascript const validator = require('validator'); if (validator.isEmail(email)) { // Valid email address } else { // Invalid email address }
3.2 Implement Content Security Policies (CSP)
Implement CSP headers to mitigate the risks of XSS attacks. CSP defines from where resources can be loaded, reducing the likelihood of malicious script execution.
javascript app.use((req, res, next) => { res.setHeader('Content-Security-Policy', "default-src 'self'"); next(); });
4. Authentication and Authorization
Proper authentication and authorization mechanisms are paramount to prevent unauthorized access to your Node.js applications.
4.1 Implement Strong Authentication
Utilize robust authentication methods such as JSON Web Tokens (JWT) or OAuth 2.0 for securing API endpoints. Store user passwords securely using hashing and salting techniques.
javascript const jwt = require('jsonwebtoken'); const token = jwt.sign({ userId: user.id }, 'secretKey', { expiresIn: '1h' });
4.2 Manage Authorization Levels
Implement role-based access control (RBAC) to ensure that users only have access to resources they are authorized to access. Assign roles and permissions to users and enforce them in your application logic.
javascript // Check if the user has the necessary role to access a resource if (user.role === 'admin') { // Grant access } else { // Deny access }
5. Protecting Against Cross-Site Scripting (XSS)
XSS attacks occur when malicious scripts are injected into your application, potentially compromising user data and security.
5.1 Sanitize User-Generated Content
Utilize libraries like dompurify to sanitize user-generated content before rendering it in the browser. This prevents the execution of malicious scripts.
javascript const createDOMPurify = require('dompurify'); const { JSDOM } = require('jsdom'); const window = new JSDOM('').window; const DOMPurify = createDOMPurify(window); const sanitizedHTML = DOMPurify.sanitize(userInput);
5.2 Utilize Security Libraries
Use security libraries like helmet to automatically set HTTP headers that can protect your application from common vulnerabilities.
javascript const helmet = require('helmet'); app.use(helmet());
6. Securing Data and Communication
Protecting data at rest and during transmission is essential to maintain the confidentiality and integrity of your application’s information.
6.1 Encrypt Data
Utilize encryption algorithms to protect sensitive data stored in databases or other storage systems. Use libraries like crypto to implement encryption and decryption processes.
javascript const crypto = require('crypto'); const encryptedData = crypto.encrypt(data, 'encryptionKey');
6.2 Use HTTPS
Always use HTTPS to encrypt data during transmission. Obtain and install SSL/TLS certificates to ensure secure communication between clients and your Node.js server.
7. Preventive Measures Against SQL Injection
Sanitize and validate user inputs to prevent SQL injection attacks. Utilize parameterized queries or prepared statements when interacting with databases.
javascript const userInput = req.body.username; const query = `SELECT * FROM users WHERE username = ?`; db.query(query, [userInput], (err, results) => { // Handle results });
8. Implement Proper Error Handling
Proper error handling prevents attackers from gaining insights into your application’s structure and potential vulnerabilities.
javascript try { // Code that might throw an error } catch (error) { console.error('An error occurred:', error.message); res.status(500).send('Something went wrong'); }
9. Implementing Security Headers
Set security-related HTTP headers to enhance your application’s security posture. These headers can mitigate various types of attacks.
javascript app.use((req, res, next) => { res.setHeader('X-Content-Type-Options', 'nosniff'); res.setHeader('X-Frame-Options', 'deny'); res.setHeader('X-XSS-Protection', '1; mode=block'); next(); });
10. Monitoring and Logging
Regularly monitor your application’s logs and set up alerts for suspicious activities. Proper logging helps you track and investigate potential security incidents.
Conclusion
Node.js has revolutionized server-side development, but it’s essential to recognize that security is an ongoing process, not a one-time task. By following these Node.js security best practices, you can fortify your applications against common vulnerabilities and ensure a safer digital experience for your users. Remember that staying informed about the latest security threats and keeping up with advancements in security practices is crucial to staying one step ahead of potential attackers.
Table of Contents
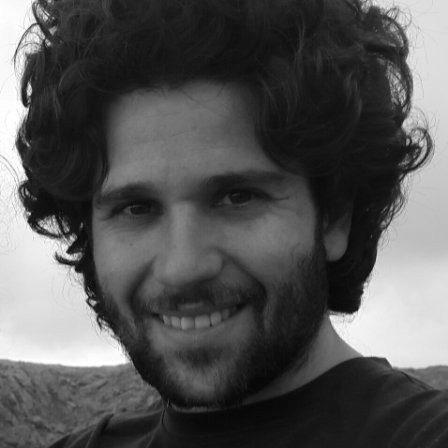
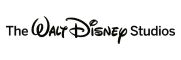