Node.js Demystified: Your Complete Guide to Mastering Server-Side JavaScript
The world of web development has seen a considerable evolution over the past few years, with numerous tools and technologies transforming the way we build applications. At the forefront of this revolution is Node.js, a powerful platform that has led to a growing demand to hire Node.js developers. These professionals use JavaScript on the server side, harnessing the capabilities of this unique platform. In this guide, perfect for those looking to hire Node.js developers or anyone interested in the technology, we will delve into the nitty-gritty of Node.js, its fundamentals, and its best practices. We will explore how to use this game-changing technology through real-life examples, showcasing the skill sets you should expect when you hire Node.js developers.
1. Introduction to Node.js
Node.js is a JavaScript runtime environment built on Chrome’s V8 JavaScript engine. Launched in 2009 by Ryan Dahl, it was designed to build scalable network applications. Unlike JavaScript that runs in your browser (client-side), Node.js allows you to run JavaScript on your computer or server (server-side). This has a plethora of advantages, like utilizing JavaScript on both the client and server sides, making development more cohesive and efficient.
One of the core characteristics of Node.js, which makes it highly sought-after by businesses looking to hire Node.js developers, is its non-blocking, event-driven architecture. This key feature enables Node.js to handle concurrent requests efficiently. Consequently, it is ideal for developing real-time applications such as chat platforms, gaming servers, collaborative tools, and streaming services. Therefore, when you hire Node.js developers, you are investing in professionals capable of leveraging this unique architecture to build fast, efficient and scalable applications.
2. Setting Up Node.js
Before diving into the code, we need to ensure that Node.js is installed on your system. You can download and install it from the official Node.js website. Once installed, you can verify the installation by opening your terminal or command line and typing:
```bash node -v ```
This command should return the installed version of Node.js.
3. Understanding The Basics
3.1 Hello World in Node.js
Now that we have Node.js installed, let’s create our first Node.js application – a traditional “Hello, World!” example. Create a new file called `app.js` and add the following lines of code:
```javascript console.log('Hello, World!'); ```
You can run this Node.js program by opening your terminal or command line, navigating to the directory where you saved `app.js`, and typing:
```bash node app.js ```
3.2 Importing Modules in Node.js
One of Node.js’s core features is its extensive use of modules, which are JavaScript libraries that you can include in your projects. Node.js includes a set of built-in modules, but you can also create your own or use third-party ones.
Let’s use the HTTP module, a built-in Node.js module, to create a simple server:
```javascript var http = require('http'); http.createServer(function (req, res) { res.writeHead(200, {'Content-Type': 'text/html'}); res.end('Hello, World!'); }).listen(8080); ```
In this code, the `require` function is used to include the HTTP module. The `http.createServer()` method creates a server that listens on port 8080. When you open your browser and navigate to `http://localhost:8080`, you should see “Hello, World!”.
4. Asynchronous Programming and Callbacks
A key part of mastering Node.js involves understanding asynchronous programming. Unlike traditional synchronous programming, where operations are performed one after another, asynchronous operations can execute concurrently.
In Node.js, most I/O operations (like reading from the network, accessing a database, or querying the filesystem) are performed asynchronously. Callbacks are often used to handle these operations. A callback is a function passed to another function as an argument and is executed after the parent function has completed.
Here’s an example of reading a file asynchronously using the `fs` (file system) module in Node.js:
```javascript var fs = require('fs'); fs.readFile('input.txt', 'utf8', function(err, data) { if (err) throw err; console.log(data); }); console.log('Reading file...'); ```
In this example, `fs.readFile` is an asynchronous function that reads a file and then uses a callback to print the file contents. The ‘Reading file…’ message will be displayed before the file contents, demonstrating the non-blocking nature of Node.js.
5. Event-Driven Programming
Node.js is inherently event-driven, meaning it uses events and event handlers to drive its architecture. An event can be anything from a user clicking a button to a server receiving a request. When an event occurs, an event handler (or callback) is invoked to handle that event.
The `events` module allows us to create and handle custom events:
```javascript var events = require('events'); var eventEmitter = new events.EventEmitter(); var myEventHandler = function () { console.log('I hear a scream!'); } eventEmitter.on('scream', myEventHandler); eventEmitter.emit('scream'); ```
Here, an event called ‘scream’ is created. When this event is fired using `eventEmitter.emit(‘scream’)`, the handler function `myEventHandler` is called.
6. Express.js: A Fast Opinionated Web Framework
Express.js is a lightweight, flexible Node.js web application framework that provides a robust set of features for building single-page, multi-page, and hybrid web applications. It simplifies the process of building web applications by providing easy-to-use methods for routing, middleware configuration, and template engine setup.
Here’s an example of creating a basic Express.js application:
```javascript var express = require('express'); var app = express(); app.get('/', function (req, res) { res.send('Hello World!'); }); app.listen(3000, function () { console.log('App listening on port 3000!'); }); ```
In this example, when you navigate to `http://localhost:3000` in your browser, you’ll see the text “Hello World!”.
Conclusion
Node.js has established itself as a go-to technology not only for Node.js developers but also for businesses looking to hire Node.js developers. This powerful player in the realm of server-side programming boasts flexibility and the capability to use JavaScript on both the client and server side, making it an ideal choice for a wide range of web development projects. From understanding the basics to mastering event-driven programming and using Express.js, this guide provides valuable insights for those who wish to hire Node.js developers. It covers essential areas to help both budding and seasoned Node.js developers get started and master Node.js. So whether you’re a developer looking to enhance your skills or a business aiming to hire Node.js developers, keep exploring and learning. Harnessing the true potential of this remarkable technology can significantly elevate your coding or project outcomes. Happy coding!
Table of Contents
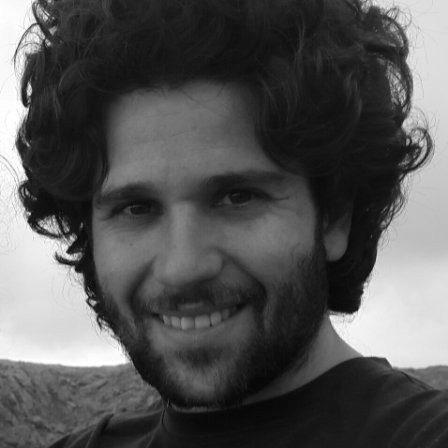
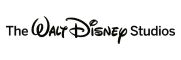