Building Serverless Applications with Node.js and AWS Lambda
In the world of modern application development, the concept of serverless computing has gained tremendous momentum. This innovative approach allows developers to focus solely on code without concerning themselves with server provisioning, scaling, and maintenance. Amazon Web Services (AWS) Lambda, coupled with the versatility of Node.js, presents a formidable combination for building serverless applications. In this guide, we’ll delve into the fundamentals of building serverless applications with Node.js and AWS Lambda, exploring the key concepts, benefits, and providing step-by-step examples.
Table of Contents
1. Understanding Serverless Architecture
1.1. What is Serverless Computing?
Serverless computing is a cloud computing model where developers can build and run applications without provisioning or managing servers. In this paradigm, the cloud provider handles the server management, scaling, and maintenance, allowing developers to focus solely on writing code. AWS Lambda, as a serverless compute service, enables developers to upload their code, specify the triggering events, and Lambda takes care of executing the code in response to those events.
1.2. Advantages of Serverless Architecture
The serverless architecture brings several benefits, including reduced operational overhead, automatic scalability, and cost optimization. Developers can deploy individual functions rather than entire applications, leading to better resource utilization and faster deployment times. Additionally, the pay-as-you-go billing model ensures cost efficiency as you only pay for the actual execution time of your functions.
1.3. AWS Lambda: An Overview
AWS Lambda is a compute service that lets you run code without provisioning or managing servers. It supports multiple programming languages, and in this guide, we’ll focus on using Node.js. Lambda functions are triggered by a variety of events, such as changes to data in an Amazon S3 bucket, updates to a DynamoDB table, or HTTP requests through Amazon API Gateway.
2. Getting Started with AWS Lambda and Node.js
2.1. Prerequisites
Before diving into building serverless applications with Node.js and AWS Lambda, you’ll need an AWS account and the AWS Command Line Interface (CLI) installed on your system. Additionally, basic knowledge of Node.js will be beneficial.
2.2. Creating Your First Lambda Function
Let’s start by creating a simple “Hello, Serverless!” Lambda function using Node.js.
javascript // index.js exports.handler = async (event) => { return { statusCode: 200, body: JSON.stringify('Hello, Serverless!'), }; };
This code defines a Lambda function that returns a “Hello, Serverless!” message with an HTTP 200 status code. To deploy this function, zip the index.js file and upload it via the AWS Management Console or CLI.
2.3. Triggering Lambda Functions
Lambda functions can be triggered by various AWS services. For example, let’s trigger the “Hello, Serverless!” function when an object is created in an S3 bucket.
javascript // index.js exports.handler = async (event) => { console.log('S3 Object Created:', event.Records[0].s3.object.key); return { statusCode: 200, body: JSON.stringify('Hello, Serverless!'), }; };
In this example, the function logs the key of the created S3 object and returns the same “Hello, Serverless!” response.
3. Serverless Application Design and Development
3.1. Deciding Function Granularity
One of the key considerations in serverless architecture is defining the granularity of your functions. Each function should serve a specific purpose and handle a single responsibility. This ensures efficient resource usage and enables easier debugging and maintenance.
3.2. Managing State and Persistence
Since Lambda functions are stateless and short-lived, managing state and persistence requires careful planning. You can use external storage solutions like Amazon DynamoDB or S3 for persistent data storage between function invocations.
3.3. Handling Dependencies
When developing Lambda functions, you often need external dependencies. Use Node.js modules and libraries as needed, but be mindful of keeping your deployment package size small. Leverage Lambda Layers to manage shared dependencies across functions.
3.4. Logging and Monitoring
Proper logging and monitoring are crucial for maintaining the health of your serverless applications. AWS CloudWatch provides tools for collecting and analyzing logs, setting up alarms, and gaining insights into the performance of your Lambda functions.
4. Integrating Lambda with Other AWS Services
4.1. Using API Gateway for HTTP Triggers
API Gateway allows you to create RESTful APIs that trigger your Lambda functions. This enables you to expose your serverless functions over HTTP endpoints. You can define API resources, methods, and integrate them with Lambda functions.
yaml # serverless.yml service: my-serverless-app provider: name: aws runtime: nodejs14.x functions: hello: handler: index.handler events: - http: path: /hello method: GET
In this example, the hello Lambda function is triggered by an HTTP GET request to the /hello path.
4.2. Working with S3 and Lambda
Lambda functions can be triggered by S3 events, such as object creation, deletion, or modification. This makes it easy to build serverless applications that react to changes in your S3 buckets.
javascript // index.js exports.handler = async (event) => { const objectKey = event.Records[0].s3.object.key; console.log(`S3 Object Created: ${objectKey}`); // Process the S3 object return { statusCode: 200, body: JSON.stringify('S3 Object Processed'), }; };
4.3. Event Streaming with Lambda and Kinesis
Amazon Kinesis enables real-time data streaming and processing. You can integrate Kinesis streams with Lambda to process and analyze streaming data. This is particularly useful for scenarios like clickstream analysis, IoT data processing, and more.
javascript // index.js exports.handler = async (event) => { for (const record of event.Records) { const payload = Buffer.from(record.kinesis.data, 'base64').toString('utf-8'); console.log('Kinesis Record:', payload); // Process the Kinesis record } return { statusCode: 200, body: JSON.stringify('Kinesis Records Processed'), }; };
5. Best Practices for Serverless Development
5.1. Optimizing Cold Starts
Cold starts can impact the performance of serverless functions. To mitigate this, use provisioned concurrency, keep deployment packages small, and implement warming strategies like scheduled invocations.
5.2. Security Considerations
Follow AWS security best practices, such as configuring IAM roles with the least privilege principle, encrypting sensitive data, and implementing proper network isolation using Virtual Private Cloud (VPC) configurations.
5.3. Testing Strategies
Unit tests, integration tests, and end-to-end tests play a vital role in ensuring the reliability of your serverless applications. Leverage frameworks like mocha and chai for testing Node.js functions.
5.4. Continuous Deployment
Implement continuous deployment practices using tools like AWS CodePipeline and AWS CodeBuild. Automate the deployment process to ensure that changes are quickly and consistently pushed to your serverless environment.
6. Case Study: Building a Serverless Image Processing Pipeline
6.1. Architecture Overview
Imagine a scenario where you need to build an image processing pipeline that resizes and stores images in S3 when they’re uploaded. Here’s how you can implement this using Lambda:
An S3 bucket triggers a Lambda function when an image is uploaded.
The Lambda function processes the image, resizes it, and stores it in another S3 bucket.
Creating Lambda Functions
javascript // resizeImage.js const AWS = require('aws-sdk'); const sharp = require('sharp'); const s3 = new AWS.S3(); exports.handler = async (event) => { const sourceBucket = event.Records[0].s3.bucket.name; const sourceKey = decodeURIComponent(event.Records[0].s3.object.key.replace(/\+/g, ' ')); const targetBucket = 'resized-images-bucket'; try { const image = await s3.getObject({ Bucket: sourceBucket, Key: sourceKey }).promise(); const resizedImage = await sharp(image.Body).resize(300, 200).toBuffer(); await s3.putObject({ Bucket: targetBucket, Key: sourceKey, Body: resizedImage }).promise(); return { statusCode: 200, body: JSON.stringify('Image Resized and Stored'), }; } catch (error) { console.error('Error:', error); return { statusCode: 500, body: JSON.stringify('Error Processing Image'), }; } };
6.2. Wiring Up the Pipeline
Configure the S3 bucket to trigger the resizeImage Lambda function whenever a new image is uploaded.
yaml # serverless.yml service: image-processing-pipeline provider: name: aws runtime: nodejs14.x functions: resizeImage: handler: resizeImage.handler events: - s3: bucket: source-images-bucket event: s3:ObjectCreated:*
6.3. Handling Failures and Retries
In a real-world scenario, you’d want to implement proper error handling and retry mechanisms for the Lambda function. You can use dead-letter queues, CloudWatch Alarms, and CloudWatch Events to monitor and manage failures.
Conclusion
Building serverless applications with Node.js and AWS Lambda opens the door to a more efficient, scalable, and cost-effective approach to application development. By abstracting away server management and infrastructure concerns, developers can focus on writing code that directly addresses business logic. From handling simple HTTP requests to processing streaming data, Lambda and Node.js offer a powerful combination that can accelerate your development processes. By understanding the core concepts, applying best practices, and exploring real-world use cases, you’re well on your way to mastering the art of serverless application development.
Table of Contents
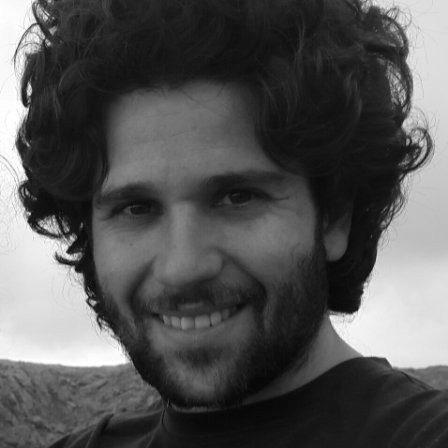
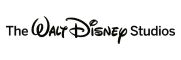