The Node.js Ecosystem: Essential Tools and Libraries
In the world of modern web development, Node.js has emerged as a powerful and versatile platform that enables developers to build server-side applications using JavaScript. Its non-blocking, event-driven architecture has paved the way for the creation of highly efficient and scalable applications. However, Node.js is not just a runtime; it’s a thriving ecosystem that includes a plethora of tools and libraries designed to simplify development, enhance performance, and extend functionality. In this article, we’ll delve into the essential tools and libraries within the Node.js ecosystem that can supercharge your development workflow.
Table of Contents
1. Introduction to the Node.js Ecosystem
1.1. What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that executes code on the server-side. It was created by Ryan Dahl in 2009 and has gained immense popularity due to its efficiency and versatility.
1.2. The Power of JavaScript on the Server
One of the standout features of Node.js is its use of JavaScript for both client-side and server-side development. This unification of the programming language allows developers to work seamlessly across different parts of an application, reducing context switching and enhancing productivity.
1.3. Non-blocking I/O and Event Loop
Node.js is built on a non-blocking, event-driven architecture, which means that it can handle multiple requests concurrently without getting blocked. The event loop is a crucial part of this architecture, ensuring that asynchronous operations are managed efficiently.
2. Essential Node.js Tools
2.1. npm (Node Package Manager)
npm is the default package manager for Node.js and is used to install, manage, and share packages of code with others. It boasts an extensive repository of open-source libraries, making it effortless to integrate third-party functionalities into your project.
bash # Installing a package using npm npm install package-name
2.2. Yarn Package Manager
Yarn is another package manager that offers faster and more reliable package installation by utilizing a local cache. It also provides features like deterministic dependency resolution and parallel execution.
bash # Installing a package using Yarn yarn add package-name nvm (Node Version Manager)
nvm allows developers to manage multiple versions of Node.js on the same machine. This is incredibly useful when working on projects that require different Node.js versions.
bash # Installing nvm curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.38.0/install.sh | bash # Installing and using a specific Node.js version nvm install 14.17.3 nvm use 14.17.3
3. Core Libraries for Node.js Development
3.1. fs (File System)
The fs module provides an API for interacting with the file system. It enables tasks like reading, writing, and manipulating files.
javascript const fs = require('fs'); fs.readFile('file.txt', 'utf8', (err, data) => { if (err) { console.error('Error reading file:', err); return; } console.log('File content:', data); });
3.2. http (HTTP)
The http module allows you to create HTTP servers and make HTTP requests.
javascript const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello, Node.js!'); }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
3.3. path (File Paths)
The path module assists in working with file paths in a cross-platform manner.
javascript const path = require('path'); const filePath = path.join(__dirname, 'files', 'example.txt'); console.log('File path:', filePath);
3.4. util (Utilities)
The util module provides various utility functions, including formatting and debugging tools.
javascript const util = require('util'); const debugLog = util.debuglog('app'); debugLog('This is a debug message');
4. Enhancing Expressiveness with Middleware
4.1. How Middleware Works
Middleware functions in Node.js (especially within frameworks like Express) are functions that sit between the incoming request and the outgoing response. They can perform tasks like logging, authentication, or modifying request/response objects.
javascript const express = require('express'); const app = express(); // Middleware function const logMiddleware = (req, res, next) => { console.log('Request received at:', new Date()); next(); // Continue to the next middleware or route handler }; app.use(logMiddleware); // Route handler app.get('/', (req, res) => { res.send('Hello, Middleware!'); }); app.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
5. Database Connectivity and ORM Libraries
5.1. mongoose (MongoDB ODM)
mongoose is a popular Object-Document Mapper for MongoDB, making it easier to work with MongoDB in a more structured and object-oriented manner.
javascript const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/myapp', { useNewUrlParser: true, useUnifiedTopology: true, }); const schema = new mongoose.Schema({ name: String, age: Number, }); const Person = mongoose.model('Person', schema); // Creating a new person const person = new Person({ name: 'John Doe', age: 30, }); person.save((err) => { if (err) { console.error('Error saving person:', err); } else { console.log('Person saved successfully'); } });
5.2. sequelize (SQL ORM)
sequelize is a promise-based ORM for SQL databases, providing a higher-level API for interacting with databases like PostgreSQL, MySQL, and SQLite.
javascript const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const User = sequelize.define('User', { username: { type: DataTypes.STRING, allowNull: false, }, email: { type: DataTypes.STRING, allowNull: false, }, }); (async () => { await sequelize.sync({ force: true }); const user = await User.create({ username: 'john_doe', email: 'john@example.com' }); console.log('User created:', user.toJSON()); })();
6. Real-time Applications with WebSockets
6.1. Introduction to WebSockets
WebSockets enable real-time, two-way communication between the client and server over a single, long-lived connection. This is in contrast to the traditional request-response model of HTTP.
6.2. Using the socket.io Library for Real-time Communication
socket.io is a widely used library for adding real-time functionality to applications. It abstracts the complexities of WebSocket connections and provides a simple API.
javascript const http = require('http'); const server = http.createServer(); const io = require('socket.io')(server); io.on('connection', (socket) => { console.log('A user connected'); socket.on('chat message', (msg) => { console.log('Received message:', msg); io.emit('chat message', msg); // Broadcast the message to all connected clients }); socket.on('disconnect', () => { console.log('A user disconnected'); }); }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
7. Asynchronous Control Flow Libraries
7.1. async (Control Flow)
The async library provides a rich set of control flow functions for managing asynchronous operations, making complex asynchronous code easier to understand and maintain.
javascript const async = require('async'); async.parallel([ (callback) => { setTimeout(() => { console.log('Task 1'); callback(null, 'Result 1'); }, 200); }, (callback) => { setTimeout(() => { console.log('Task 2'); callback(null, 'Result 2'); }, 100); }, ], (err, results) => { if (err) { console.error('Error:', err); } else { console.log('Results:', results); } });
7.2. Promises and async/await
Node.js introduced native support for Promises, and later, the async and await syntax. These features simplify asynchronous code and make it appear more synchronous.
javascript const fetch = require('node-fetch'); async function fetchExample() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log('Fetched data:', data); } catch (error) { console.error('Error fetching data:', error); } } fetchExample();
8. Testing and Assertion Libraries
8.1. Mocha and Jest
Mocha and Jest are popular testing frameworks for Node.js. They provide tools for structuring test suites, defining test cases, and making assertions about your code’s behavior.
javascript const assert = require('assert'); function add(a, b) { return a + b; } describe('Addition', () => { it('should add two numbers', () => { const result = add(2, 3); assert.strictEqual(result, 5); }); });
9. Building User Interfaces with Node.js
9.1. React and the MERN Stack
React is a JavaScript library for building user interfaces, and when combined with Node.js, MongoDB, and Express, it forms the MERN stack—a powerful choice for creating dynamic web applications.
9.2. Server-Side Rendering with Next.js
Next.js is a framework that builds on React and offers features like server-side rendering (SSR) and static site generation (SSG), improving performance and SEO.
10. Authentication and Security Libraries
10.1. Passport.js: User Authentication
Passport.js is a widely used library for implementing authentication strategies in Node.js applications, supporting various authentication methods such as local, OAuth, and more.
10.2. bcrypt: Password Hashing
bcrypt is a popular library for securely hashing passwords. It ensures that user passwords are not stored in plain text, enhancing security.
11. Monitoring and Performance Optimization
11.1. pm2: Process Manager for Node.js
pm2 is a production process manager that simplifies application deployment and management. It provides features like monitoring, auto-restart, and load balancing.
11.2. New Relic: Application Performance Monitoring
New Relic is a monitoring tool that helps developers identify performance bottlenecks, optimize resource usage, and improve overall application speed.
12. Deployment and Containerization
12.1. Deploying Node.js Applications
Deploying Node.js applications often involves setting up a production environment, configuring web servers, and managing deployment pipelines.
12.2. Dockerizing Node.js Apps
Docker allows you to package your Node.js application along with its dependencies into a container, ensuring consistency across different environments.
Conclusion
The Node.js ecosystem is a rich and evolving landscape that offers a wide range of tools and libraries to streamline your development process, enhance your application’s performance, and unlock new possibilities. Whether you’re building real-time applications, working with databases, optimizing performance, or ensuring security, the Node.js ecosystem has something for every aspect of development. By leveraging the power of these tools and libraries, developers can create robust and feature-rich applications that cater to the modern demands of web development. So, dive in, explore, and make the most of the incredible Node.js ecosystem!
Table of Contents
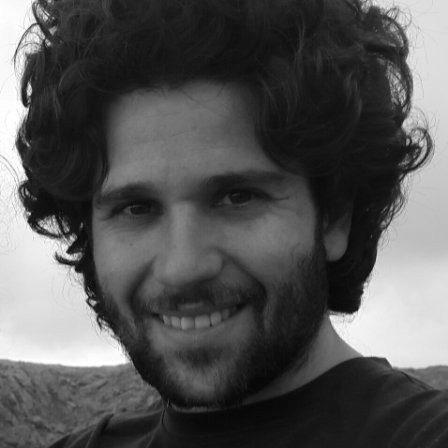
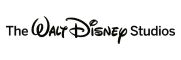