Using WebSockets for Real-Time Communication in Node.js
In the world of modern web applications, providing real-time communication and interactive experiences has become a crucial aspect of user engagement. Whether you’re building a chat application, a collaborative tool, or a live update feature, using WebSockets can greatly enhance the real-time capabilities of your Node.js application. In this article, we’ll delve into the concept of WebSockets and explore how to implement them in Node.js to achieve seamless and instant communication between clients and servers.
Table of Contents
1. Understanding WebSockets
Before we dive into the implementation details, let’s briefly understand what WebSockets are and why they are so valuable for real-time communication.
1.1. What are WebSockets?
WebSockets are a protocol that provides full-duplex communication channels over a single TCP connection. Unlike the traditional request-response model of HTTP, where the client sends a request and waits for the server to respond, WebSockets allow both the client and the server to send messages to each other at any time. This bidirectional communication enables real-time data transfer, making it ideal for applications that require instant updates.
1.2. Benefits of Using WebSockets
- Real-Time Updates: WebSockets enable instant communication between clients and servers, allowing applications to provide real-time updates to users without the need for constant polling.
- Reduced Latency: Unlike traditional HTTP requests that involve overhead due to repeated connections, WebSockets maintain a persistent connection, minimizing latency and reducing data transfer overhead.
- Efficient Resource Usage: Since WebSockets maintain a single connection, they are more efficient in terms of resource usage compared to opening and closing multiple connections for each interaction.
- Bi-Directional Communication: With WebSockets, both the client and the server can send messages independently, facilitating interactive and dynamic experiences.
- Scalability: WebSockets can be scaled using technologies like load balancers, making them suitable for applications with a large number of concurrent users.
Now that we have a grasp of what WebSockets are and why they are advantageous, let’s move on to implementing them in a Node.js environment.
2. Implementing WebSockets in Node.js
In this section, we’ll walk through the steps to implement WebSockets in a Node.js application. We’ll be using the popular ws library, which provides a simple way to work with WebSockets.
Step 1: Setting Up the Project
First, let’s set up a new Node.js project and install the ws library:
bash mkdir websocket-demo cd websocket-demo npm init -y npm install ws
Step 2: Creating the WebSocket Server
Next, create a file named server.js and set up a WebSocket server:
javascript const WebSocket = require('ws'); const server = new WebSocket.Server({ port: 3000 }); server.on('connection', (socket) => { console.log('Client connected'); socket.on('message', (message) => { console.log(`Received: ${message}`); socket.send(`You said: ${message}`); }); socket.on('close', () => { console.log('Client disconnected'); }); });
In this code, we import the ws library and create a WebSocket server that listens on port 3000. When a client connects, we log the event and set up event listeners for incoming messages and the client closing the connection. When a message is received, we log it and send a response back to the client.
Step 3: Creating the WebSocket Client
Now, let’s create a simple WebSocket client in a file named client.js:
javascript const WebSocket = require('ws'); const socket = new WebSocket('ws://localhost:3000'); socket.on('open', () => { console.log('Connected to server'); socket.send('Hello, server!'); }); socket.on('message', (message) => { console.log(`Received from server: ${message}`); }); socket.on('close', () => { console.log('Connection closed'); });
In this code, we create a WebSocket client that connects to the server running on ws://localhost:3000. When the connection is established, we send a message to the server. We also set up event listeners to handle incoming messages and the connection being closed.
Step 4: Running the Application
With the server and client files in place, let’s run the application:
bash node server.js
In a separate terminal:
bash node client.js
You’ll see output indicating that the client has connected, sent a message, and received a response from the server.
3. Enhancing Real-Time Experiences
Now that you have a basic WebSocket implementation, you can build upon this foundation to create more advanced real-time experiences in your Node.js application.
3.1. Broadcasting Messages
To broadcast messages to all connected clients, you can modify the server code as follows:
javascript server.on('connection', (socket) => { console.log('Client connected'); socket.on('message', (message) => { console.log(`Received: ${message}`); // Broadcast the message to all clients server.clients.forEach((client) => { if (client !== socket && client.readyState === WebSocket.OPEN) { client.send(`Someone said: ${message}`); } }); }); socket.on('close', () => { console.log('Client disconnected'); }); });
Now, when a client sends a message, the server broadcasts that message to all connected clients except the sender.
3.2. Implementing Real-Time Notifications
Imagine you’re building a notification system. You can use WebSockets to instantly notify users about new events. Here’s a basic example:
javascript // Server server.on('connection', (socket) => { console.log('Client connected'); // Simulate a new event and send it to the client every 5 seconds const eventInterval = setInterval(() => { if (socket.readyState === WebSocket.OPEN) { socket.send('New event: You have a new notification!'); } }, 5000); socket.on('close', () => { clearInterval(eventInterval); console.log('Client disconnected'); }); });
3.3. Building a Real-Time Chat Application
WebSockets are perfect for building real-time chat applications. You can extend the WebSocket server to handle chat messages and implement the client-side UI to display messages in real time. Here’s a high-level overview:
- When a user sends a message in the chat UI, the client sends the message to the server via the WebSocket connection.
- The server receives the message and broadcasts it to all connected clients.
- Each client displays the incoming message in the chat UI.
- By implementing this flow, you can create a seamless chat experience where messages are instantly shared among participants.
Conclusion
In this tutorial, we’ve explored the power of WebSockets in enabling real-time communication between clients and servers in Node.js applications. We’ve covered the benefits of using WebSockets, walked through setting up a WebSocket server and client, and discussed ways to enhance real-time experiences.
WebSockets open up a world of possibilities for creating interactive and engaging applications. Whether you’re building a chat app, a live dashboard, or a collaborative tool, incorporating WebSockets can transform your user experience by providing instant updates and dynamic content. So go ahead, experiment with WebSockets in your Node.js projects, and elevate your applications to the next level of interactivity. Happy coding!
Table of Contents
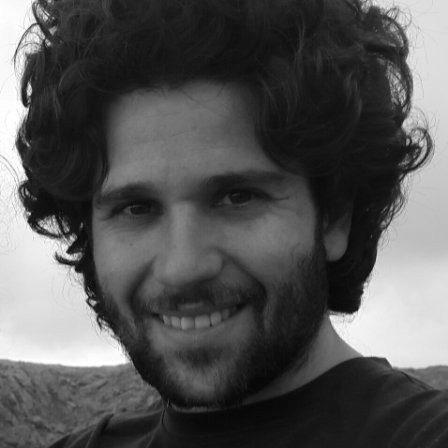
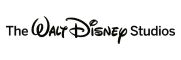