Objective-C and App Extensions: Extending iOS Functionality
Mobile app development is an ever-evolving field, and creating innovative and user-friendly apps has become a significant challenge for developers. With iOS, Apple has provided developers with a robust platform and a wide array of tools to build powerful and engaging applications. One such tool is Objective-C, a programming language that has been a staple in iOS app development for years. In this blog, we will explore Objective-C and its role in creating App Extensions, a feature that extends the functionality of iOS apps.
Table of Contents
1. Understanding Objective-C
1.1. Introduction to Objective-C
Objective-C is a powerful and flexible programming language that has been the primary language for iOS and macOS app development for decades. It combines elements of C and Smalltalk, providing developers with a unique and expressive way to build applications. While Swift has gained popularity in recent years, Objective-C still plays a crucial role in the Apple ecosystem, especially in maintaining legacy codebases.
1.2. Key Features of Objective-C
1.2.1. Dynamic Typing
Objective-C is known for its dynamic typing system, which allows objects to be treated in a flexible manner at runtime. This feature enables developers to create highly adaptable and extensible code.
1.2.2. Message Passing
In Objective-C, objects communicate with each other through message passing. This messaging system allows objects to send messages to other objects, leading to a highly decoupled and modular code structure.
1.2.3. Automatic Reference Counting (ARC)
Objective-C introduced Automatic Reference Counting, a memory management system that simplifies memory management by automatically handling object retention and release. This feature reduces the likelihood of memory leaks and makes memory management more straightforward.
2. App Extensions: Enhancing iOS Functionality
2.1. What Are App Extensions?
App Extensions are a way to enhance the functionality of iOS apps. They allow developers to create additional features that can be accessed from various parts of the iOS ecosystem, such as the Today View, Share Sheet, or even from other apps. These extensions provide a seamless experience for users and extend the capabilities of your app beyond its main interface.
2.2. Types of App Extensions
2.2.1. Today Extension
A Today Extension, also known as a widget, allows you to display relevant information from your app in the Today View on the iOS device’s home screen. This feature provides users with quick access to essential data without opening the full app.
2.2.2. Share Extension
A Share Extension allows users to share content from other apps or web browsers directly to your app. This extension type is particularly useful for apps that deal with content sharing, such as social media or note-taking apps.
2.2.3. Action Extension
An Action Extension lets users perform actions on content from within other apps. For example, you can create an Action Extension that translates text, shares it to social media, or performs custom operations on the selected content.
2.2.4. Photo Editing Extension
A Photo Editing Extension allows users to edit photos using your app’s features without leaving the Photos app. This extension type is beneficial for apps that offer image editing or filters.
2.3. Why Use App Extensions?
App Extensions provide several benefits for both developers and users:
Improved User Experience: Extensions make it easier for users to interact with your app’s features without launching the full app, resulting in a more streamlined and convenient experience.
Increased App Engagement: By offering extensions, you can encourage users to interact with your app more frequently, increasing user engagement and retention.
Enhanced Accessibility: App Extensions make your app’s functionality accessible from various parts of the iOS ecosystem, making it more visible and accessible to users.
3. Creating App Extensions with Objective-C
Now that we understand the significance of App Extensions, let’s dive into how to create them using Objective-C. We’ll explore the process step by step, including code samples to illustrate key concepts.
3.1. Prerequisites
Before you begin, ensure you have the following prerequisites in place:
- A Mac computer with Xcode installed.
- Basic knowledge of Objective-C and iOS app development.
Step 1: Create a New Target
To add an App Extension to your existing iOS app, follow these steps:
- Open your Xcode project.
- Go to the File menu and select “New” -> “Target…”
- In the template chooser, select the type of extension you want to create (e.g., Today Extension, Share Extension).
- Configure the extension settings and click “Finish.”
Step 2: Configure the Extension
Once you’ve created the extension target, you need to configure it. This involves setting up the user interface, specifying the extension’s capabilities, and providing necessary permissions. Here’s an example of configuring a Today Extension:
objective // TodayViewController.m #import "TodayViewController.h" #import <NotificationCenter/NotificationCenter.h> @interface TodayViewController () <NCWidgetProviding> @end @implementation TodayViewController - (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view from its nib. } - (void)widgetPerformUpdateWithCompletionHandler:(void (^)(NCUpdateResult))completionHandler { // Perform any updates to your widget's content, optionally calling the completion handler when done. completionHandler(NCUpdateResultNewData); } @end
Step 3: Communicate with the Main App
App Extensions typically need to communicate with the main app to fetch or update data. You can use various techniques to establish communication between the extension and the app, such as App Groups and UserDefaults. Here’s a code snippet demonstrating how to use App Groups for data sharing:
objective // TodayViewController.m NSString *appGroupName = @"group.com.yourappname.appgroup"; NSUserDefaults *sharedDefaults = [[NSUserDefaults alloc] initWithSuiteName:appGroupName]; [sharedDefaults setObject:@"Shared Data" forKey:@"key"]; [sharedDefaults synchronize]; // In the main app: NSString *appGroupName = @"group.com.yourappname.appgroup"; NSUserDefaults *sharedDefaults = [[NSUserDefaults alloc] initWithSuiteName:appGroupName]; NSString *sharedData = [sharedDefaults objectForKey:@"key"];
Step 4: Build and Test
Once you’ve implemented your App Extension, build and test it on your iOS device or simulator. Ensure that the extension functions as expected and integrates seamlessly with your main app.
Step 5: Distribute Your App with Extensions
When you’re satisfied with your App Extension, you can distribute your app on the App Store. Ensure that you provide clear descriptions of your app’s extensions in the App Store listing to inform users about their functionality.
4. Best Practices for App Extensions
Creating effective and user-friendly App Extensions requires adhering to best practices:
4.1. Keep It Simple
Design your extensions to be straightforward and easy to use. Users should be able to accomplish tasks quickly without unnecessary complexity.
4.2. Optimize for Performance
Efficiency is critical for extensions. Ensure that your code is well-optimized to minimize resource consumption and load times.
4.3. Maintain Consistency
Keep the user interface and functionality consistent with your main app to provide a seamless experience.
4.4. Test Across Devices
Test your extensions on various iOS devices and screen sizes to ensure compatibility and responsiveness.
4.5. Stay Updated
Regularly update your extensions to fix bugs, add new features, and remain compatible with the latest iOS versions.
Conclusion
Objective-C continues to play a vital role in iOS app development, especially when it comes to extending app functionality through App Extensions. By creating well-designed extensions and following best practices, you can enhance the user experience, increase user engagement, and make your app more accessible and visible within the iOS ecosystem. So, explore the power of Objective-C and App Extensions to take your iOS app to the next level. Happy coding!
In this comprehensive guide, we’ve delved into the world of Objective-C and App Extensions, exploring their significance and how to create them step by step. Armed with this knowledge, you can now extend the functionality of your iOS apps and provide users with a more immersive and accessible experience. Don’t hesitate to experiment with App Extensions and unlock new possibilities for your iOS app development journey.
Table of Contents
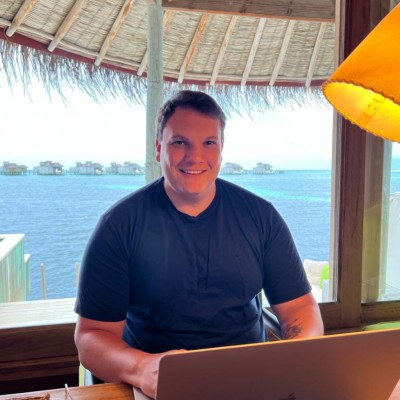
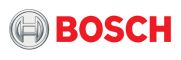