Objective-C and Augmented Reality: Creating Immersive iOS Experiences
Augmented Reality (AR) has rapidly transformed from science fiction into a cutting-edge technology with countless real-world applications. With Apple’s ARKit framework, developers can seamlessly integrate AR experiences into iOS apps. In this blog, we’ll explore the exciting synergy between Objective-C and Augmented Reality, and discover how to create immersive iOS experiences that captivate users.
Table of Contents
1. Introduction to Augmented Reality
1.1. What is Augmented Reality?
Augmented Reality blends the digital and physical worlds by overlaying computer-generated information onto the user’s view of the real world. Unlike Virtual Reality (VR), which immerses users in entirely virtual environments, AR enhances the real world with digital elements.
1.2. The Rise of AR in iOS
Apple has played a pivotal role in popularizing AR with the introduction of ARKit. This framework empowers developers to leverage the power of AR on iOS devices, opening up endless possibilities for interactive and immersive applications.
2. The Role of Objective-C in iOS Development
Objective-C, while no longer the primary language for iOS development, still plays a vital role in maintaining and extending existing iOS applications. If you have legacy projects or simply prefer Objective-C, it’s entirely feasible to incorporate AR capabilities.
2.1. Setting Up Your Environment
Before diving into AR development, ensure you have Xcode, Apple’s integrated development environment, installed. Additionally, familiarity with Objective-C is essential for this journey.
3. Getting Started with ARKit in Objective-C
Now that you’re all set up, let’s take the first step in creating immersive iOS AR experiences with Objective-C.
3.1. Creating a New AR Project
Open Xcode and create a new project. Select the “Augmented Reality App” template, which provides a solid starting point for AR development.
3.2. Understanding ARSCNView
The heart of your AR app is the ARSCNView, a subclass of SCNView that combines SceneKit with ARKit. This view renders the camera feed and allows you to overlay 3D content onto the real world.
Here’s a code snippet to initialize the ARSCNView:
objective // Import necessary headers #import <ARKit/ARKit.h> #import <SceneKit/SceneKit.h> // Create and configure the ARSCNView ARSCNView *arSceneView = [[ARSCNView alloc] initWithFrame:self.view.frame]; arSceneView.session = [ARSession new]; arSceneView.delegate = self; [self.view addSubview:arSceneView];
3.3. Adding 3D Objects
One of the most exciting aspects of AR development is placing 3D objects in the real world. ARKit makes this surprisingly straightforward. Here’s an example of how to add a 3D cube:
objective SCNBox *cube = [SCNBox boxWithWidth:0.1 height:0.1 length:0.1 chamferRadius:0]; SCNNode *cubeNode = [SCNNode nodeWithGeometry:cube]; cubeNode.position = SCNVector3Make(0, 0, -0.5); // Place the cube 50 centimeters in front of the camera [arSceneView.scene.rootNode addChildNode:cubeNode];
3.4. Running Your AR App
Build and run your AR app on a compatible iOS device. You’ll be amazed at how the virtual cube seamlessly integrates with the real world through the device’s camera.
4. Interactivity and User Engagement
Creating an immersive AR experience goes beyond static 3D objects. You can engage users by allowing them to interact with AR elements.
4.1. Detecting Touch Gestures
Recognizing touch gestures is vital for user interaction. Here’s a sample of how to detect a tap gesture:
objective UITapGestureRecognizer *tapGesture = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(handleTap:)]; [arSceneView addGestureRecognizer:tapGesture];
And the corresponding handleTap: method:
objective - (void)handleTap:(UITapGestureRecognizer *)gesture { CGPoint tapLocation = [gesture locationInView:arSceneView]; NSArray<ARHitTestResult *> *hitTestResults = [arSceneView hitTest:tapLocation types:ARHitTestResultTypeExistingPlaneUsingExtent]; if (hitTestResults.count > 0) { // Handle the tap on an existing plane ARHitTestResult *hitResult = [hitTestResults firstObject]; // Perform your desired action here } }
4.2. Adding Virtual Buttons
Buttons provide a familiar interface for users. You can create virtual buttons in AR by adding 2D overlays that respond to touch events. Here’s a simplified example:
objective UIButton *virtualButton = [UIButton buttonWithType:UIButtonTypeRoundedRect]; [virtualButton setTitle:@"Tap Me" forState:UIControlStateNormal]; [virtualButton addTarget:self action:@selector(virtualButtonTapped:) forControlEvents:UIControlEventTouchUpInside]; // Position the virtual button in 3D space virtualButton.frame = CGRectMake(0, 0, 100, 40); virtualButton.center = CGPointMake(0, 0, -0.5); // Adjust the position as needed [arSceneView addSubview:virtualButton];
5. Advanced AR Techniques in Objective-C
5.1. World Tracking
ARKit’s world tracking capabilities enable your app to understand the environment, allowing for more realistic and stable AR experiences. To enable world tracking, simply set the ARSession configuration:
objective ARWorldTrackingConfiguration *worldTrackingConfiguration = [ARWorldTrackingConfiguration new]; [arSceneView.session runWithConfiguration:worldTrackingConfiguration];
5.2. Occlusion
ARKit can handle occlusion, which means that virtual objects can appear behind real-world objects, enhancing the illusion of immersion. To enable occlusion:
objective ARWorldTrackingConfiguration *worldTrackingConfiguration = [ARWorldTrackingConfiguration new]; worldTrackingConfiguration.environmentTexturing = ARWorldTrackingConfigurationEnvironmentTexturingAutomatic; [arSceneView.session runWithConfiguration:worldTrackingConfiguration];
6. Tips for Optimal AR Development
Creating immersive AR experiences requires attention to detail. Here are some tips to ensure your AR app shines:
6.1. Performance Optimization
AR experiences can be demanding on device resources. Profile your app and optimize performance for a smooth user experience.
6.2. Realistic Lighting
Pay attention to lighting to ensure virtual objects blend seamlessly with the real world.
6.3. User Guidance
Provide clear instructions or hints to guide users on how to interact with AR elements.
6.4. Testing in Different Environments
Test your AR app in various real-world environments to ensure it works reliably.
Conclusion
Objective-C and Augmented Reality are a powerful combination for creating immersive iOS experiences. With ARKit, you can seamlessly integrate AR into your Objective-C projects, opening up a world of possibilities for interactive and engaging applications. Start experimenting with AR today, and you’ll be amazed at the immersive experiences you can create for your users.
Table of Contents
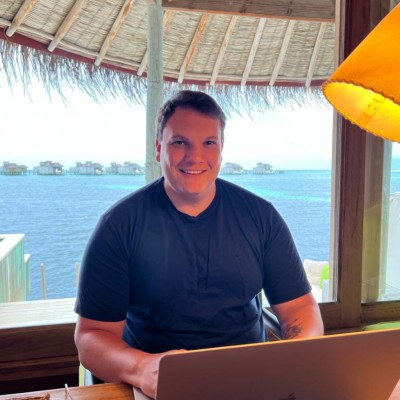
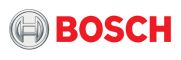