Mastering Objective-C: A Comprehensive Guide for iOS Developers
Objective-C has been the primary programming language for iOS app development for many years. While Swift has gained popularity in recent years, Objective-C remains an essential skill for iOS developers. Whether you’re new to iOS development or an experienced Swift developer looking to enhance your skills, mastering Objective-C is a valuable asset that can open doors to a wider range of opportunities.
In this comprehensive guide, we will explore Objective-C from its fundamentals to advanced concepts. By the end, you will have a solid understanding of the language and be able to build robust and efficient iOS applications.
1. Introduction to Objective-C
1.1 History and Background
Objective-C was developed in the early 1980s and became the primary programming language for macOS and iOS development. It is a superset of the C programming language, adding object-oriented features and dynamic runtime capabilities. Many foundational frameworks and libraries in iOS are written in Objective-C, making it crucial for developers to have a good understanding of the language.
1.2 Advantages of Objective-C
Objective-C has several advantages that have contributed to its longevity and widespread usage in the iOS development community. One of its main strengths is its dynamic runtime, which enables features like introspection and dynamic method resolution. This flexibility allows for powerful runtime manipulations and is a key component of many iOS frameworks.
1.3 Setting Up the Development Environment
To begin mastering Objective-C, you’ll need to set up your development environment. Install Xcode, Apple’s integrated development environment (IDE), which provides everything you need to build iOS apps. Xcode includes the Objective-C compiler, debugger, and other essential tools.
2. Objective-C Basics
2.1 Syntax and Structure
Objective-C syntax may seem unfamiliar at first, especially if you’re coming from a language like Swift or Java. However, it follows a set of conventions that make it readable and expressive. Understanding the syntax and structure is crucial to writing Objective-C code effectively.
objective #import <Foundation/Foundation.h> // Sample Objective-C code @interface Person : NSObject @property (nonatomic, strong) NSString *name; @property (nonatomic, assign) NSInteger age; - (void)sayHello; @end @implementation Person - (void)sayHello { NSLog(@"Hello, my name is %@!", self.name); } @end int main(int argc, const char * argv[]) { @autoreleasepool { Person *person = [[Person alloc] init]; person.name = @"John"; person.age = 25; [person sayHello]; } return 0; }
2.2 Data Types and Variables
Objective-C has built-in data types like integers, floating-point numbers, and characters, as well as more complex types like strings and arrays. Understanding these data types and how to work with them is essential for building iOS applications.
2.3 Control Flow Statements
Control flow statements like if-else, for loops, and switch statements allow you to control the flow of execution in your Objective-C code. Learning how to use these statements effectively will help you make decisions and iterate over collections.
2.4 Arrays and Collections
Objective-C provides various collection classes like NSArray and NSDictionary that allow you to store and manipulate groups of objects. These collections are widely used in iOS development, and understanding how to work with them is essential.
2.5 Memory Management
Objective-C uses manual memory management with reference counting. Learning about memory management techniques, including retain, release, and autorelease, is critical to avoiding memory leaks and managing object lifetimes.
3. Object-Oriented Programming in Objective-C
3.1 Classes and Objects
Objective-C is an object-oriented language, and understanding classes and objects is fundamental to building iOS applications. Learn how to define classes, create objects, and access their properties and methods.
3.2 Properties and Methods
Properties define the state of an object, while methods define its behavior. Objective-C provides a rich set of syntax and conventions for declaring and implementing properties and methods.
3.3 Inheritance and Polymorphism
Inheritance allows you to create specialized classes that inherit the properties and methods of a base class. Polymorphism enables objects of different classes to be treated interchangeably, providing flexibility and code reuse.
3.4 Protocols and Delegates
Protocols define a set of methods that a class can implement to adhere to a particular behavior. Delegates, based on protocols, allow objects to communicate and respond to events. Understanding protocols and delegates is crucial for iOS development.
3.5 Categories and Extensions
Categories and extensions allow you to extend existing classes without subclassing them. They are powerful tools for adding functionality to classes and organizing your code effectively.
4. Working with UIKit
4.1 Building User Interfaces
UIKit is the primary framework for building user interfaces in iOS. Learn how to create and customize views, handle user input, and respond to events using UIKit.
4.2 Responding to User Input
Interacting with users is a core part of any iOS app. Learn how to handle touch events, gestures, and user input effectively using UIKit.
4.3 Navigation and View Controllers
Navigation controllers and view controllers are essential components of iOS app development. Master the concepts of navigation stacks, push and pop operations, and the view controller lifecycle.
4.4 Table Views and Collection Views
Table views and collection views are used to display and manage large sets of data in iOS apps. Learn how to populate data, handle selection, and implement custom layouts for these view types.
4.5 Gesture Recognizers and Animations
Gesture recognizers allow you to detect and respond to gestures like taps, swipes, and rotations. Animations bring life to your UI by adding visual effects and transitions. Discover how to incorporate these features into your app.
5. Advanced Objective-C Techniques
5.1 Blocks and Grand Central Dispatch
Blocks are a powerful language feature that allows you to encapsulate and pass around units of code. Grand Central Dispatch (GCD) is a framework that provides easy-to-use APIs for concurrent programming. Learn how to leverage blocks and GCD for multi-threading and asynchronous operations.
5.2 Key-Value Coding (KVC) and Key-Value Observing (KVO)
Key-Value Coding (KVC) enables indirect access to an object’s properties using strings. Key-Value Observing (KVO) allows objects to observe changes in properties and respond accordingly. These mechanisms are widely used in iOS development.
5.3 Error Handling and Exception Handling
Learn how to handle errors and exceptions gracefully in Objective-C. Understand the differences between NSError and exceptions, and when to use each mechanism in your code.
5.4 Runtime Programming
Objective-C’s dynamic runtime provides powerful capabilities for introspection and runtime manipulation. Discover how to use the runtime to dynamically create classes, modify method implementations, and perform other advanced tasks.
6. Best Practices and Tips for Objective-C Development
6.1 Code Organization and Structure
Organizing your Objective-C code effectively is crucial for maintainability and readability. Learn about common code organization patterns and best practices, including file structure, naming conventions, and code documentation.
6.2 Debugging and Troubleshooting
Debugging is an essential skill for any developer. Discover how to use Xcode’s debugging tools effectively, set breakpoints, inspect variables, and diagnose and fix common issues in Objective-C code.
6.3 Performance Optimization
Learn techniques to optimize your Objective-C code for performance. From memory management and efficient algorithms to profiling and optimizing critical code sections, these practices will help your app run smoothly.
6.4 Unit Testing and Documentation
Writing unit tests ensures the correctness of your code, while documentation enhances its understandability. Explore techniques for writing effective unit tests and documenting your Objective-C code.
6.5 Version Control and Collaboration
Learn how to use version control systems like Git effectively to manage your Objective-C projects and collaborate with other developers. Understand branching, merging, and other essential version control concepts.
7. Transitioning from Objective-C to Swift
7.1 Interoperability between Objective-C and Swift
Objective-C and Swift can coexist within the same project, allowing you to leverage both languages’ strengths. Learn how to use Objective-C code from Swift and vice versa.
7.2 Converting Objective-C Code to Swift
If you’re considering migrating your existing Objective-C codebase to Swift, learn about strategies, tools, and techniques to make the transition smoother.
7.3 Choosing the Right Language for Your Project
Understand the strengths and weaknesses of Objective-C and Swift to make informed decisions when starting a new project or maintaining an existing one.
Conclusion:
Mastering Objective-C is a valuable skill for iOS developers. Whether you’re building new apps or maintaining legacy codebases, a deep understanding of Objective-C will enable you to tackle a wider range of challenges and build robust, efficient, and scalable iOS applications. This comprehensive guide has covered the fundamentals, advanced techniques, and best practices of Objective-C development, empowering you to take your iOS development skills to the next level. So dive in, practice, and explore the vast world of iOS development with Objective-C.
Table of Contents
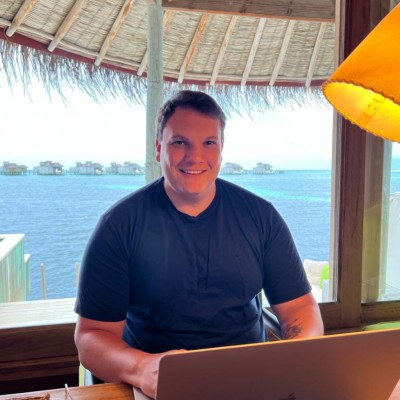
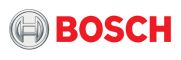