Objective-C Data Types and Variables: A Comprehensive Overview
Objective-C, a powerful and widely-used programming language, offers a wide range of data types and variables to handle different kinds of data. Understanding these data types and variables is essential for building robust and efficient applications. In this comprehensive overview, we will explore the fundamental concepts of Objective-C data types and variables, including their definitions, usage, and examples. Whether you’re a beginner or an experienced developer looking to refresh your knowledge, this guide will serve as a valuable resource to enhance your understanding of Objective-C programming.
1. Overview of Objective-C Data Types
Objective-C provides a wide range of data types to handle different kinds of data in your programs. These data types can be categorized into two main groups: primitive data types and object data types.
1.1 Primitive Data Types:
Primitive data types are the basic building blocks of any programming language. In Objective-C, primitive data types include integers, floating-point numbers, characters, and booleans. These data types are used to represent simple values and have predefined ranges and operations.
1.2 Object Data Types:
Objective-C is an object-oriented language, and object data types are an integral part of its syntax. Object data types are used to represent complex data structures and are based on classes and frameworks. Objective-C provides a wide range of object data types through its built-in classes and the Foundation framework.
2. Declaring Variables in Objective-C
Before using a data type, you need to declare a variable of that type. In Objective-C, variable declaration involves specifying the data type and an optional identifier (variable name).
2.1 Syntax for Variable Declaration:
objective dataType variableName; Naming Conventions:
Objective-C follows camel case naming conventions for variables. It is recommended to use meaningful names that reflect the purpose and content of the variable.
2.2 Initializing Variables:
Variables can be assigned initial values at the time of declaration using the assignment operator (=). Here’s an example:
objective int age = 25;
3. Primitive Data Types
Objective-C provides several primitive data types to represent different kinds of values.
3.1 Integers:
Integers represent whole numbers without any fractional part. Objective-C supports different sizes of integers, including int (4 bytes), short (2 bytes), long (4 or 8 bytes), and long long (8 bytes).
objective int myInt = 42;
3.2 Floating-Point Numbers:
Floating-point numbers are used to represent numbers with a fractional part. Objective-C supports float (4 bytes) and double (8 bytes) data types for floating-point numbers.
objective float myFloat = 3.14;
3.3 Characters and Strings:
Objective-C uses the char data type to represent individual characters and strings. A single character is enclosed in single quotes (‘ ‘), while a string is enclosed in double quotes (” “).
objective char myChar = 'A'; NSString *myString = @"Hello, World!";
3.4 Booleans:
The BOOL data type is used to represent boolean values, which can be either YES or NO.
objective BOOL isTrue = YES;
4. Object Data Types
Objective-C’s object data types are based on classes and frameworks. These data types represent complex data structures and provide extensive functionality through methods and properties.
4.1 Objective-C Classes:
Objective-C allows you to define your own classes to create custom object types. Classes encapsulate data and behavior into a single entity, allowing you to create objects and perform operations on them.
objective @interface Person : NSObject @property NSString *name; @property int age; @end @implementation Person @end Person *person = [[Person alloc] init]; person.name = @"John"; person.age = 30;
Foundation Framework Classes:
Objective-C provides a rich set of classes through the Foundation framework. These classes cover a wide range of functionalities, including collections, strings, dates, and more.
objective NSArray *array = @[@"Apple", @"Banana", @"Orange"]; NSString *name = [NSString stringWithFormat:@"John Doe"];
5. Working with Data Types and Variables
Objective-C provides various features and techniques to work with data types and variables efficiently.
5.1 Type Conversion:
Objective-C supports automatic type conversion between compatible data types. However, explicit type conversion is required when assigning values of different types or when performing operations involving different data types.
objective int age = 25; float height = (float)5.8;
5.2 Type Inference:
Objective-C uses type inference to deduce the type of a variable based on its assigned value. This feature allows you to omit the explicit declaration of the data type.
objective var height = 5.8; // Inferred as double
5.3 Constants and Literals:
Objective-C allows you to define constants using the const keyword. Constants are variables whose values cannot be changed once assigned.
objective const int MAX_SIZE = 100;
Conclusion
In this comprehensive overview, we explored the fundamental concepts of Objective-C data types and variables. We discussed the different types of data available in Objective-C, including primitive data types and object data types. We also learned about variable declaration, initialization, and naming conventions.
Understanding data types and variables is crucial for developing efficient and reliable Objective-C applications. By grasping these concepts, you can manipulate data effectively and write clean and maintainable code. With the knowledge gained from this guide, you can confidently utilize Objective-C’s powerful data types and variables to build robust applications that meet your specific requirements.
Table of Contents
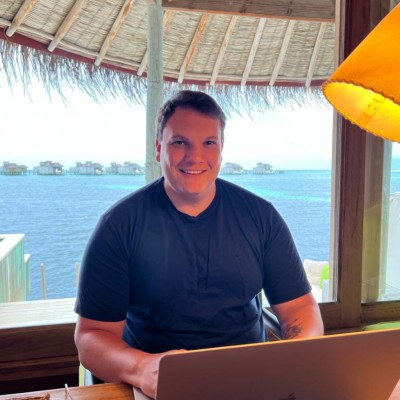
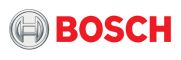