Objective-C File Handling: Reading and Writing Data in iOS
File handling is a fundamental aspect of iOS app development. Whether you’re saving user preferences, caching data, or working with external files, understanding how to read and write data is essential. In this guide, we’ll explore Objective-C’s file handling capabilities in iOS, covering everything from reading to writing data. By the end, you’ll have the knowledge you need to work with files effectively in your iOS applications.
Table of Contents
1. Understanding File Paths in iOS
Before diving into reading and writing data, it’s crucial to understand how iOS manages file paths. iOS uses a sandboxed file system, which means that each app has its own isolated file storage space. You won’t have direct access to the entire device’s file system. Instead, you’ll work within your app’s designated directory.
1.1. App’s Document Directory
The primary location for storing user-generated content is the app’s document directory. You can access it using the NSSearchPathForDirectoriesInDomains function:
objective NSArray *documentPaths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES); NSString *documentDirectory = [documentPaths objectAtIndex:0];
Now that we have a basic understanding of file paths in iOS, let’s explore how to read and write data within our app’s sandboxed file system.
2. Reading Data from a File
Reading data from a file is a common task when working with files in iOS. You might want to load configuration settings, retrieve saved user data, or parse a JSON file. Objective-C provides straightforward methods to accomplish this.
2.1. Reading Text Files
To read the contents of a text file, you can use the NSString class’s method stringWithContentsOfFile:encoding:error::
objective NSString *filePath = [documentDirectory stringByAppendingPathComponent:@"example.txt"]; NSError *error; NSString *fileContents = [NSString stringWithContentsOfFile:filePath encoding:NSUTF8StringEncoding error:&error]; if (error) { NSLog(@"Error reading file: %@", [error localizedDescription]); } else { NSLog(@"File contents: %@", fileContents); }
This code snippet loads the contents of the “example.txt” file from the app’s document directory and stores it in the fileContents variable. Be sure to handle any potential errors, as shown in the code above.
2.2. Reading Binary Files
When working with binary data, such as images or custom file formats, you can use the NSData class to read the contents of a file:
objective NSString *filePath = [documentDirectory stringByAppendingPathComponent:@"image.png"]; NSData *fileData = [NSData dataWithContentsOfFile:filePath]; if (fileData) { UIImage *image = [UIImage imageWithData:fileData]; // Use the image as needed } else { NSLog(@"Failed to read file or file does not exist."); }
Here, we load the binary data from the “image.png” file and create a UIImage from it. You can adapt this code to handle other binary file formats as required.
3. Writing Data to a File
Now that we know how to read data, let’s explore how to write data to files in Objective-C.
3.1. Writing Text Files
To write text data to a file, you can use the NSString class’s method writeToFile:atomically:encoding:error::
objective NSString *textToWrite = @"Hello, File Handling in iOS!"; NSString *filePath = [documentDirectory stringByAppendingPathComponent:@"output.txt"]; NSError *error; BOOL success = [textToWrite writeToFile:filePath atomically:YES encoding:NSUTF8StringEncoding error:&error]; if (success) { NSLog(@"Data written successfully to file: %@", filePath); } else { NSLog(@"Error writing file: %@", [error localizedDescription]); }
In this example, we create a string textToWrite and write it to the “output.txt” file in the app’s document directory. Make sure to handle any errors that may occur during the writing process.
3.2. Writing Binary Files
When dealing with binary data, such as saving images or custom file formats, you can use the NSData class to write data to a file:
objective UIImage *imageToSave = [UIImage imageNamed:@"example.png"]; NSData *imageData = UIImagePNGRepresentation(imageToSave); NSString *filePath = [documentDirectory stringByAppendingPathComponent:@"saved_image.png"]; BOOL success = [imageData writeToFile:filePath atomically:YES]; if (success) { NSLog(@"Image saved successfully to file: %@", filePath); } else { NSLog(@"Error saving image to file."); }
Here, we save an image as binary data to the “saved_image.png” file in the app’s document directory. Ensure that you handle errors gracefully in your app to provide a smooth user experience.
4. Checking File Existence
Before reading or writing data, it’s essential to check if a file exists at the specified path. You can do this using the NSFileManager class:
objective NSFileManager *fileManager = [NSFileManager defaultManager]; NSString *filePath = [documentDirectory stringByAppendingPathComponent:@"example.txt"]; if ([fileManager fileExistsAtPath:filePath]) { NSLog(@"File exists at path: %@", filePath); } else { NSLog(@"File does not exist at path: %@", filePath); }
This code checks if a file named “example.txt” exists in the app’s document directory. Depending on the result, you can decide whether to read or write to the file.
5. Deleting Files
Deleting files is another common file handling task. You may want to remove temporary files or clean up data that is no longer needed. To delete a file, use the NSFileManager class:
objective NSString *filePathToDelete = [documentDirectory stringByAppendingPathComponent:@"file_to_delete.txt"]; NSFileManager *fileManager = [NSFileManager defaultManager]; NSError *error; BOOL success = [fileManager removeItemAtPath:filePathToDelete error:&error]; if (success) { NSLog(@"File deleted successfully: %@", filePathToDelete); } else { NSLog(@"Error deleting file: %@", [error localizedDescription]); }
This code snippet deletes a file named “file_to_delete.txt” from the app’s document directory. Remember to handle any errors that might occur during the deletion process.
6. Working with Directories
In addition to handling individual files, you may need to work with directories. For example, you might want to create a directory to organize related files. Here’s how you can create a directory using the NSFileManager class:
objective NSString *directoryToCreate = [documentDirectory stringByAppendingPathComponent:@"MyDirectory"]; NSFileManager *fileManager = [NSFileManager defaultManager]; NSError *error; BOOL success = [fileManager createDirectoryAtPath:directoryToCreate withIntermediateDirectories:YES attributes:nil error:&error]; if (success) { NSLog(@"Directory created successfully: %@", directoryToCreate); } else { NSLog(@"Error creating directory: %@", [error localizedDescription]); }
This code creates a directory named “MyDirectory” within the app’s document directory. You can customize the directory’s name and location to suit your app’s needs.
Conclusion
File handling is a crucial aspect of iOS app development. With Objective-C, you have a powerful set of tools at your disposal to read, write, check, and delete files and directories within your app’s sandboxed file system. By mastering these file handling techniques, you’ll be better equipped to build robust and data-driven iOS applications. Whether you’re working with text or binary data, understanding file paths, or managing directories, you now have the knowledge to handle files effectively in your iOS projects. Happy coding!
Table of Contents
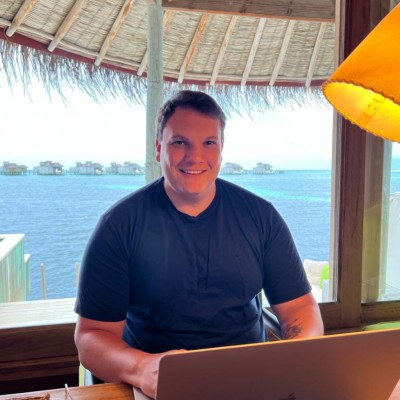
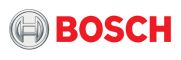