Understanding Objective-C: The Foundation of iOS Development
Objective-C, the primary programming language for developing iOS and macOS applications, has been the foundation of Apple’s software ecosystem for many years. Understanding Objective-C is crucial for aspiring iOS developers as it forms the basis for building robust and scalable applications for Apple’s devices. In this blog, we will delve into the essentials of Objective-C, including its syntax, object-oriented principles, and provide code samples to illustrate key concepts.
1. What is Objective-C?
Objective-C is a general-purpose, object-oriented programming language that builds upon the C programming language. It was initially developed in the early 1980s and gained prominence when Apple adopted it as the primary language for iOS and macOS development. Objective-C combines the low-level capabilities of C with dynamic runtime features and object-oriented principles, making it a powerful language for building robust applications.
1.1 Origins and Evolution
Objective-C was created by Brad Cox and Tom Love as an extension of the C programming language. It was developed with the intention of providing a more flexible and dynamic alternative to C++. Apple embraced Objective-C in the late 1980s and made it the language of choice for building applications on their platforms.
Over the years, Objective-C evolved and gained popularity among developers due to its dynamic nature and compatibility with existing C codebases. However, in recent years, Apple introduced Swift as a modern alternative to Objective-C, encouraging developers to adopt the new language. Despite this, Objective-C continues to play a vital role in maintaining and updating legacy codebases.
1.2 Object-Oriented Programming
Objective-C is an object-oriented language, which means it revolves around the concept of objects. Objects are instances of classes, which define the structure and behavior of the objects. Object-oriented programming (OOP) allows developers to organize code into reusable, modular components, enhancing code readability and maintainability.
2. Objective-C Syntax
Understanding Objective-C syntax is crucial for writing effective code. Let’s explore some of the key syntax elements:
2.1 Classes and Objects
Classes are the building blocks of Objective-C programs. They encapsulate data and behavior within objects. To define a class, developers use the @interface and @end keywords. Here’s an example:
objective @interface Person : NSObject { NSString *_name; NSInteger _age; } - (instancetype)initWithName:(NSString *)name age:(NSInteger)age; - (void)sayHello; @end
In the above example, we define a class named “Person” that inherits from the “NSObject” class. The instance variables _name and _age store the name and age of the person, respectively. The class also declares two methods: initWithName:age: for initializing the object and sayHello for displaying a greeting.
To create an instance of the “Person” class, we use the alloc and init methods:
objective Person *person = [[Person alloc] initWithName:@"John" age:25];
2.2 Methods and Messages
Methods in Objective-C are equivalent to functions in other programming languages. They define the behavior of objects and can be invoked by sending messages to objects. Objective-C uses square brackets to send messages. Here’s an example:
objective - (void)sayHello { NSLog(@"Hello, my name is %@ and I'm %ld years old.", _name, _age); }
In the above code, the sayHello method uses the NSLog function to print a greeting, including the person’s name and age.
To send a message to an object, we use the square bracket syntax:
objective [person sayHello];
2.3 Properties and Instance Variables
Properties provide a convenient way to encapsulate data within objects. They automatically generate accessors and mutators, also known as getters and setters. Here’s an example:
objective @property (nonatomic, copy) NSString *name;
In the above code, we declare a property named “name” of type NSString. The nonatomic attribute ensures that the property is not affected by concurrent access. The copy attribute specifies that the property should make a copy of the assigned value.
To access or modify the value of a property, we use dot notation:
objective person.name = @"Alice"; NSString *personName = person.name;
2.4 Memory Management
Objective-C uses reference counting for memory management. Developers must explicitly manage the allocation and deallocation of objects to prevent memory leaks. Key memory management methods include retain, release, and autorelease.
With the introduction of Automatic Reference Counting (ARC), the compiler automatically inserts memory management code based on certain rules, reducing the burden on developers. However, it’s essential to understand the underlying memory management principles to ensure efficient resource utilization.
3. Objective-C Fundamentals
Objective-C provides several fundamental concepts that enhance code modularity and flexibility.
3.1 Inheritance and Polymorphism
Inheritance allows developers to create new classes based on existing ones, inheriting their properties and behaviors. This promotes code reuse and enables polymorphism, where objects of different classes can be treated interchangeably. Objective-C supports single inheritance, meaning a class can inherit from only one superclass.
3.2 Categories and Protocols
Categories provide a way to extend existing classes without subclassing them. They allow developers to add new methods to classes at runtime, enhancing code modularity and reusability. Protocols define a set of methods that a class can conform to, enabling the definition of common interfaces.
3.3 Dynamic Typing
Objective-C supports dynamic typing, allowing objects to be assigned to variables of different types at runtime. This flexibility enables late binding and dynamic method resolution, enhancing code adaptability.
4. Code Samples
Let’s explore some code samples to solidify our understanding of Objective-C.
4.1 Creating Classes and Objects
objective @interface Car : NSObject @property (nonatomic, copy) NSString *make; @property (nonatomic, copy) NSString *model; - (void)startEngine; @end @implementation Car - (void)startEngine { NSLog(@"The %@ %@'s engine is running.", self.make, self.model); } @end Car *myCar = [[Car alloc] init]; myCar.make = @"Toyota"; myCar.model = @"Camry"; [myCar startEngine];
4.2 Implementing Methods
objective @interface Calculator : NSObject - (NSInteger)addNumber:(NSInteger)num1 toNumber:(NSInteger)num2; @end @implementation Calculator - (NSInteger)addNumber:(NSInteger)num1 toNumber:(NSInteger)num2 { return num1 + num2; } @end Calculator *myCalculator = [[Calculator alloc] init]; NSInteger sum = [myCalculator addNumber:5 toNumber:3];
4.3 Working with Properties
objective @interface Rectangle : NSObject @property (nonatomic) CGFloat width; @property (nonatomic) CGFloat height; @property (nonatomic, readonly) CGFloat area; @end @implementation Rectangle - (CGFloat)area { return self.width * self.height; } @end Rectangle *myRectangle = [[Rectangle alloc] init]; myRectangle.width = 5.0; myRectangle.height = 3.0; CGFloat rectangleArea = myRectangle.area;
4.4 Memory Management in Objective-C
objective @interface Person : NSObject @property (nonatomic, copy) NSString *name; @end @implementation Person - (void)dealloc { [_name release]; [super dealloc]; } @end
Conclusion
Objective-C serves as the foundation of iOS and macOS development, providing developers with a powerful and flexible language to build applications for Apple’s platforms. By understanding Objective-C’s syntax, object-oriented principles, and fundamental concepts, aspiring iOS developers can embark on a successful journey of creating compelling and feature-rich applications. Start exploring Objective-C today, and unlock endless possibilities in the world of iOS development.
Table of Contents
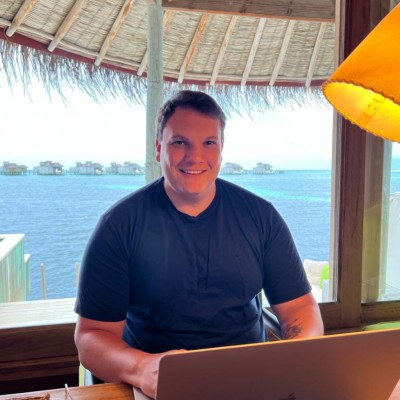
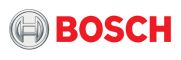