A Beginner’s Guide to Objective-C: Getting Started with iOS Development
Are you eager to dive into iOS development and build amazing apps for Apple devices? Look no further! This beginner’s guide will walk you through the fundamentals of Objective-C, the programming language used for iOS and macOS development. Whether you have prior programming experience or are completely new to coding, this guide will equip you with the knowledge and skills you need to start building your own iOS applications. Let’s get started!
1. Understanding Objective-C: An Introduction
Objective-C is a powerful and widely-used programming language that forms the foundation of iOS and macOS app development. This section provides an overview of Objective-C, its features, and its role in the Apple ecosystem.
1.1 What is Objective-C?
Objective-C is an object-oriented programming language that adds Smalltalk-style messaging to the C programming language. It was developed by Brad Cox and Tom Love in the early 1980s and has since become the primary language used for iOS and macOS app development.
1.2 Key Features of Objective-C
Objective-C combines the power and efficiency of the C language with object-oriented programming paradigms. Some key features of Objective-C include:
- Dynamic runtime: Objective-C leverages a dynamic runtime, allowing for dynamic method dispatch, introspection, and the ability to add new methods at runtime.
- Message passing: Objective-C uses message passing to invoke methods on objects, which allows for highly flexible and dynamic behavior.
- Integration with C: Objective-C can directly call C functions and use C data types, making it highly compatible with existing C codebases.
2. Setting Up Your Development Environment
Before we dive into coding, let’s set up your development environment to write Objective-C code and build iOS apps. This section covers the essential tools and resources you need to get started.
2.1 Xcode: The IDE for iOS Development
Xcode is the integrated development environment (IDE) provided by Apple for iOS and macOS app development. It includes a suite of tools, such as a code editor, debugger, and interface builder, to help you create, test, and debug your apps.
2.2 Installing Xcode
To install Xcode, follow these steps:
- Open the App Store on your Mac.
- Search for “Xcode” and click “Get” to download and install it.
- Once the installation is complete, launch Xcode and proceed with the initial setup.
3. Objective-C Basics: Syntax and Concepts
Now that you have Xcode set up, let’s explore the basics of Objective-C syntax and some fundamental concepts that will help you understand and write Objective-C code.
3.1 Classes and Objects
Objective-C follows a class-based object-oriented programming model. A class is a blueprint for creating objects, while objects are instances of a class. Here’s an example of a basic Objective-C class:
objectivec @interface Person : NSObject @property (nonatomic, copy) NSString *name; - (void)introduce; @end @implementation Person - (void)introduce { NSLog(@"Hello, my name is %@", self.name); } @end
3.2 Properties and Methods
Objective-C uses properties to encapsulate data within objects and methods to define the behavior of objects. Properties declare instance variables and provide accessors and mutators for those variables. Methods define the actions an object can perform. Here’s an example:
objectivec @interface Car : NSObject @property (nonatomic, copy) NSString *model; - (void)startEngine; @end @implementation Car - (void)startEngine { NSLog(@"Engine started for %@", self.model); } @end
4. Building Your First iOS App with Objective-C
Now that you understand the basics, let’s dive into building your first iOS app using Objective-C. In this section, we’ll walk through the steps of creating a simple “Hello World” app.
4.1 Creating a New Project
Launch Xcode and follow these steps to create a new iOS project:
- Click on “Create a new Xcode project” in the welcome window.
- Select “App” under the iOS tab and choose “Single View App.”
- Provide a product name, organization identifier, and select Objective-C as the language.
- Choose a location to save your project and click “Create.”
4.2 Adding UI Elements and Logic
In the Xcode interface builder, you can design your app’s user interface by dragging and dropping UI elements onto the canvas. Once you’ve designed your interface, you can connect the UI elements to your Objective-C code to add functionality. Here’s an example of connecting a button to an action:
objc // ViewController.h @interface ViewController : UIViewController - (IBAction)buttonTapped:(id)sender; @end // ViewController.m @implementation ViewController - (IBAction)buttonTapped:(id)sender { NSLog(@"Button tapped!"); } @end
5. Next Steps and Resources
Congratulations! You’ve built your first iOS app with Objective-C. As you continue your iOS development journey, here are some next steps and resources to help you deepen your knowledge:
Learning Objective-C: Dive deeper into Objective-C by exploring advanced topics such as memory management, protocols, and categories. Books like “Programming in Objective-C” by Stephen Kochan can provide in-depth learning.
Apple Developer Documentation: Explore Apple’s official documentation to learn about the iOS frameworks, libraries, and best practices.
Online Communities and Tutorials: Join iOS developer communities, such as Stack Overflow and GitHub, to connect with other developers and access tutorials and code samples.
Conclusion:
Objective-C is a versatile and powerful programming language for iOS and macOS app development. This beginner’s guide has provided you with a solid foundation to start building your own iOS applications. From understanding the basics of Objective-C syntax to creating your first app, you’re now equipped with the knowledge and resources to continue your iOS development journey. Happy coding!
Table of Contents
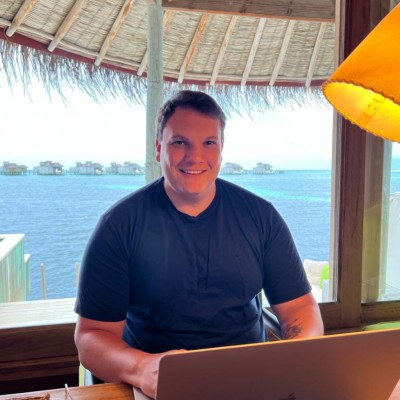
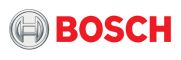