Objective-C and In-App Purchases: Monetizing iOS Applications
In the ever-evolving world of mobile app development, creating a stunning and useful iOS application is only half the battle. To turn your hard work into a rewarding endeavor, you need a solid monetization strategy. One of the most effective ways to generate revenue from your iOS app is through in-app purchases. In this blog, we’ll dive into the world of Objective-C and explore how you can effectively monetize your iOS applications using in-app purchases.
Table of Contents
1. Understanding the Power of In-App Purchases
In-app purchases, often abbreviated as IAPs, are a key component of the iOS ecosystem. They allow developers to offer additional content, features, or services within their apps that users can buy. Whether you’re offering a premium version of your app, virtual goods, or subscription services, in-app purchases provide a flexible monetization model that can suit a wide range of applications.
1.1. Benefits of In-App Purchases
Increased Revenue: In-app purchases can significantly boost your app’s revenue compared to a one-time purchase model.
- User Engagement: They keep users engaged by offering new content or features, increasing user retention.
- Flexibility: You can experiment with various pricing models, such as one-time purchases, subscriptions, or consumable items.
- Ad Revenue Enhancement: If your app is ad-supported, in-app purchases can provide an additional income stream, reducing your reliance on ads.
- Dynamic Updates: You can add new content or features post-launch, keeping your app fresh and appealing.
2. Setting Up Your Project
Before diving into the code, make sure you have an Xcode project ready to work with. If you don’t have one yet, create a new project or open an existing one.
2.1. Importing StoreKit
To work with in-app purchases in Objective-C, you’ll need to import the StoreKit framework. StoreKit is a framework provided by Apple that enables interactions with the App Store for making purchases and managing transactions.
objective #import <StoreKit/StoreKit.h>
3. Creating In-App Purchase Products
To monetize your app through in-app purchases, you’ll need to create products within App Store Connect, Apple’s platform for managing app distribution and monetization.
- Log in to App Store Connect: Go to App Store Connect and sign in with your Apple ID.
- Access Your App: Select the app you want to add in-app purchases to from the “My Apps” section.
- In-App Purchases: In the left sidebar, click on “In-App Purchases.”
- Create New In-App Purchase: Click the “+” button to create a new in-app purchase product.
- Choose the Type: Apple offers various types of in-app purchase products, including consumables, non-consumables, subscriptions, and more. Select the type that best suits your app’s monetization strategy.
- Fill in Product Details: Provide all the necessary details for your in-app purchase, including its name, description, and pricing. Make sure to set the product ID, which you’ll use in your Objective-C code.
- Save: Save your in-app purchase product.
4. Coding In-App Purchases in Objective-C
Now that you’ve set up your project and created in-app purchase products, let’s dive into the code. Here’s a step-by-step guide to integrating in-app purchases into your Objective-C app:
4.1. Request User Authorization
Before making any in-app purchases, you need to request the user’s authorization. This is a crucial step to ensure compliance with Apple’s guidelines and provide a seamless user experience.
objective - (void)requestAuthorization { [SKPaymentQueue.defaultQueue addTransactionObserver:self]; [[SKPaymentQueue defaultQueue] restoreCompletedTransactions]; }
The requestAuthorization method adds an observer to the payment queue and initiates the restoration of any completed transactions. You should call this method when your app launches or when the user navigates to a screen where in-app purchases are available.
4.2. Implement the Payment Queue Observer
As an observer of the payment queue, your app will receive notifications about transactions and their status changes. Implement the following delegate methods to handle these notifications:
objective - (void)paymentQueue:(SKPaymentQueue *)queue updatedTransactions:(NSArray<SKPaymentTransaction *> *)transactions { for (SKPaymentTransaction *transaction in transactions) { switch (transaction.transactionState) { case SKPaymentTransactionStatePurchased: // Handle a successful purchase [self completeTransaction:transaction]; break; case SKPaymentTransactionStateFailed: // Handle a failed transaction [self failedTransaction:transaction]; break; case SKPaymentTransactionStateRestored: // Handle a restored transaction (e.g., for non-consumable products) [self restoreTransaction:transaction]; break; default: break; } } }
The updatedTransactions method is called whenever there is a change in the transaction state. You’ll typically handle three states: purchased, failed, and restored. In the completeTransaction, failedTransaction, and restoreTransaction methods, you should implement logic to handle each of these states accordingly.
4.3. Making a Purchase
When the user decides to make a purchase, you’ll initiate the process by creating a SKPayment object and adding it to the payment queue.
objective - (void)purchaseProductWithID:(NSString *)productID { SKProduct *product = [self.products objectForKey:productID]; if (product) { SKPayment *payment = [SKPayment paymentWithProduct:product]; [[SKPaymentQueue defaultQueue] addPayment:payment]; } else { // Handle product not found error } }
In this code, productID is the identifier of the in-app purchase product you want to buy. Make sure to retrieve the product details from the SKProduct object using the product ID.
4.4. Completing a Successful Purchase
When a purchase is successful, you should unlock the content or feature associated with the purchase and finish the transaction.
objective - (void)completeTransaction:(SKPaymentTransaction *)transaction { // Unlock content or feature for the user // ... // Finish the transaction [[SKPaymentQueue defaultQueue] finishTransaction:transaction]; }
The completeTransaction method is where you should provide the purchased item to the user and then call finishTransaction to mark the transaction as complete.
4.5. Handling Failed Transactions
If a transaction fails, you should inform the user and finish the transaction.
objective - (void)failedTransaction:(SKPaymentTransaction *)transaction { if (transaction.error.code != SKErrorPaymentCancelled) { // Show an error message to the user } // Finish the transaction [[SKPaymentQueue defaultQueue] finishTransaction:transaction]; }
For failed transactions that are not canceled by the user, you can display an appropriate error message. It’s important to finish the transaction even in case of failure to avoid reprocessing it.
4.6. Restoring Previous Purchases
Allowing users to restore their previous purchases is essential, especially for non-consumable products or when users switch to a new device.
objective - (void)restoreTransaction:(SKPaymentTransaction *)transaction { // Restore the content or feature for the user // ... // Finish the transaction [[SKPaymentQueue defaultQueue] finishTransaction:transaction]; }
In the restoreTransaction method, provide the user with the restored content or feature and finish the transaction.
5. Testing In-App Purchases
Before submitting your app to the App Store, it’s crucial to thoroughly test your in-app purchases to ensure they work correctly. Apple provides a sandbox environment that simulates the App Store but doesn’t involve real money.
To test in-app purchases in the sandbox environment:
- Create a Test User: In App Store Connect, go to “Users and Access” and create a test user account.
- Enable Sandbox Mode: On your test device, open “Settings,” navigate to “iTunes & App Store,” and sign out of your regular Apple ID. Then sign in using the test user account.
- Use the App: Open your app and make test purchases. You won’t be charged real money, and you can test the entire purchase flow.
- Check Transaction Logs: In Xcode, open the “Console” tab to view transaction logs and ensure everything is working as expected.
6. Monetization Strategies
In-app purchases offer a range of monetization strategies you can explore for your iOS app. Here are some popular options:
6.1. Consumable Purchases
Consumable purchases are items that users can buy and use, then buy again. Examples include virtual currency, power-ups, or in-game items. These encourage ongoing spending within your app.
6.2. Non-Consumable Purchases
Non-consumable purchases are items that users buy once and keep forever. Examples include unlocking premium features or removing ads. This model offers a one-time revenue boost.
6.3. Subscriptions
Subscriptions are ideal for apps with regularly updated content or services. Users pay on a recurring basis (e.g., monthly or annually) to access premium content or features. This provides a steady income stream.
6.4. Free Trials
Offering free trials for subscription-based services is an effective way to attract users and convert them into paying customers. Users can experience the premium features before committing to a subscription.
6.5. Tiered Pricing
For subscription-based apps, consider offering multiple subscription tiers with varying levels of access. This allows users to choose a plan that fits their needs and budget.
7. Best Practices for In-App Purchases
To maximize your app’s revenue and provide a positive user experience, consider these best practices:
7.1. Clear Pricing Information
Ensure that your pricing and subscription details are transparent to users. Display the benefits of each purchase option clearly.
7.2. Offer Value
Provide real value to users through your in-app purchases. Users should feel that the purchase enhances their experience or solves a problem.
7.3. Personalization
Consider personalizing in-app purchase offers based on user behavior and preferences. This can increase conversion rates.
7.4. Optimize the Purchase Flow
Make the purchase process as simple and intuitive as possible. Minimize the number of steps and distractions.
7.5. Handle Errors Gracefully
Implement error handling to address any issues that may occur during the purchase process. Ensure that users receive helpful error messages.
7.6. Monitor and Analyze
Use analytics tools to track in-app purchase performance. Monitor conversion rates, revenue, and user feedback to make informed decisions.
Conclusion
Monetizing your iOS applications using in-app purchases in Objective-C is a powerful way to generate revenue and enhance user engagement. By following best practices, implementing a robust payment flow, and thoroughly testing your in-app purchases, you can create a successful monetization strategy for your app. Remember that a user-centric approach, offering value, and clear communication of your in-app purchase options are key to building trust and retaining satisfied customers.
In today’s competitive app market, it’s not just about building great apps; it’s also about sustaining your development efforts and ensuring a steady income stream. In-app purchases, when implemented thoughtfully, can help you achieve that while delivering a superior user experience. So, go ahead and start monetizing your iOS applications effectively using Objective-C and in-app purchases. Your app and your bottom line will thank you for it.
Table of Contents
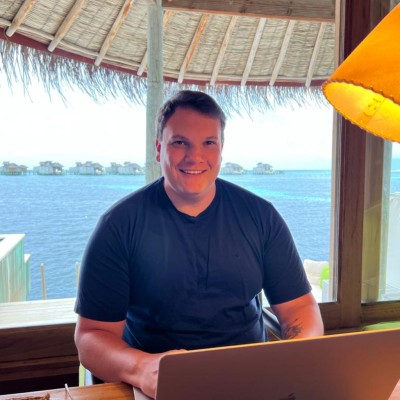
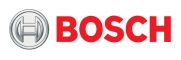