Objective-C Memory Management: Tips and Best Practices
Objective-C, the primary programming language for iOS app development, employs manual memory management. While this approach provides developers with granular control over memory allocation and deallocation, it also poses challenges. Inefficient memory management can lead to crashes, performance issues, and memory leaks. Therefore, it is crucial to understand the concepts, techniques, and best practices for effective Objective-C memory management. In this blog, we will explore various tips and best practices to help you optimize memory usage and ensure a stable and efficient iOS app.
1. Understanding Objective-C Memory Management:
1.1 Retain-Release Model:
Objective-C uses a retain-release model, where objects have a reference count that tracks how many times they have been retained or released. The retain method increments the reference count, while the release method decrements it. When the reference count reaches zero, the object is deallocated.
1.2 Reference Counting:
Reference counting is the core mechanism of Objective-C memory management. Developers explicitly manage the reference count by retaining and releasing objects. It is essential to maintain a balanced and accurate reference count to prevent memory leaks or premature deallocation.
1.3 Autorelease Pool:
Autorelease pool allows you to defer the release of an object until the current autorelease pool is drained. Autorelease pools are useful when you need to create temporary objects within a loop or during method invocations. Autorelease pools prevent objects from being released too early.
2. Tips for Effective Memory Management:
2.1 Use ARC (Automatic Reference Counting):
Automatic Reference Counting (ARC) is a compiler feature introduced in Objective-C, which automatically inserts retain and release calls for you. ARC manages most of the memory management for you, significantly reducing the chance of memory leaks and other memory-related issues.
2.2 Avoid Strong Reference Cycles:
Strong reference cycles, also known as retain cycles, occur when two objects hold strong references to each other. This prevents their reference counts from reaching zero, leading to memory leaks. To avoid strong reference cycles, use weak or unowned references when appropriate, such as when dealing with delegate relationships.
2.3 Be Mindful of Retain Cycles:
Retain cycles can also occur with blocks, where blocks capture the objects they reference. When a block captures a strong reference to an object, and the object also retains the block, a retain cycle is formed. Use weak or __block references inside blocks to break retain cycles.
2.4 Use Weak References:
Weak references are useful when you want to reference an object without incrementing its reference count. Weak references automatically become nil when the object they refer to is deallocated, preventing crashes due to accessing a released object.
2.5 Use Autorelease Pool Effectively:
When dealing with loops or situations where temporary objects are created frequently, it’s important to use autorelease pool effectively. By creating and draining autorelease pools inside the loop, you ensure that temporary objects are released in a timely manner and don’t accumulate unnecessary memory usage.
3. Best Practices for Memory Optimization:
3.1 Avoid Unnecessary Object Creation:
Creating too many unnecessary objects can result in excessive memory usage. Consider reusing objects or using value types like structs where appropriate. Avoid creating unnecessary intermediate objects during calculations or loops.
3.2 Release Unused Objects:
It is essential to release objects when they are no longer needed. Failing to release unused objects can lead to memory leaks. Be mindful of objects that may retain references to other objects and ensure that their reference counts are appropriately managed.
3.3 Use Lazy Loading:
Lazy loading is a technique where objects are created only when they are first accessed. This approach reduces memory consumption by deferring the creation of objects until they are actually needed. Consider using lazy loading for objects that may not be used immediately or frequently.
3.4 Avoid Excessive Autoreleases:
While autorelease is a powerful mechanism, excessive use can lead to increased memory usage. Be cautious when using autorelease, especially in performance-critical code paths. Instead, consider using explicit retain and release calls for objects that need immediate memory management.
3.5 Implement Custom Memory Management if Necessary:
In some cases, you may need to implement custom memory management techniques beyond reference counting. This might involve implementing object pooling, caching, or other strategies specific to your app’s requirements. Evaluate your app’s memory usage patterns and consider custom memory management when appropriate.
Code Samples:
Here’s an example demonstrating the use of weak references:
objectivec __weak MyViewController *weakRef = self; dispatch_async(dispatch_get_main_queue(), ^{ MyViewController *strongRef = weakRef; if (strongRef) { // Use the strong reference to MyViewController } });
In the code above, we use a weak reference to avoid creating a strong reference cycle between the block and the view controller.
Conclusion:
Effective memory management is crucial for developing stable and efficient iOS apps. By understanding Objective-C memory management concepts and implementing the tips and best practices outlined in this blog, you can optimize memory usage, prevent memory leaks, and ensure your app’s performance. Remember to leverage ARC, be mindful of retain cycles, use weak references appropriately, and implement efficient memory optimization techniques tailored to your app’s requirements.
Table of Contents
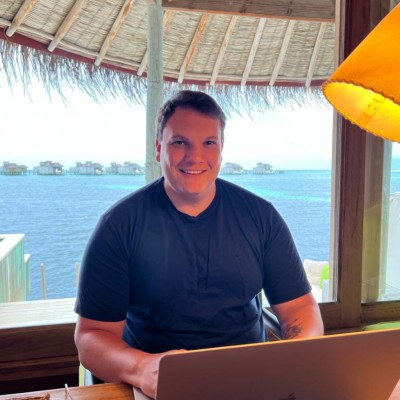
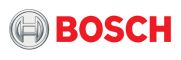