Objective-C Networking: Communicating with Web Services in iOS Apps
In the ever-evolving world of mobile app development, one aspect remains constant – the need to connect with web services. Whether it’s fetching data from a remote server or sending user-generated content, networking is an integral part of iOS app development. In this comprehensive guide, we will explore Objective-C networking, equipping you with the knowledge and tools necessary to seamlessly communicate with web services in your iOS apps.
1. Introduction to Objective-C Networking
1.1 Understanding the Role of Networking in iOS Apps
Networking is the backbone of many iOS applications. It enables apps to communicate with remote servers, retrieve data, send updates, and more. Common use cases for networking in iOS apps include fetching news articles, streaming media, authenticating users, and posting user-generated content.
1.2 Why Objective-C for Networking?
Objective-C, with its robust and time-tested libraries, remains a solid choice for networking in iOS development. While Swift has gained popularity, Objective-C continues to be a valuable language, especially for projects with legacy codebases or where interoperability with C and C++ is required. In this guide, we will focus on Objective-C to demonstrate how networking can be achieved effectively.
2. Getting Started with NSURLSession
NSURLSession is the cornerstone of networking in Objective-C. It provides a powerful and flexible API for making network requests. Let’s get started with the basics.
2.1 Creating NSURLSession
To begin using NSURLSession, you need to create a session configuration and a session itself. Here’s how you can create a default configuration and session:
objective NSURLSessionConfiguration *defaultConfig = [NSURLSessionConfiguration defaultSessionConfiguration]; NSURLSession *session = [NSURLSession sessionWithConfiguration:defaultConfig];
This code creates a default session configuration and then a session object using that configuration.
2.2 Making GET and POST Requests
Now that you have a session, you can use it to make GET and POST requests to web services. Here’s an example of making a GET request:
objective NSString *urlString = @"https://api.example.com/data"; NSURL *url = [NSURL URLWithString:urlString]; NSURLRequest *request = [NSURLRequest requestWithURL:url]; NSURLSessionDataTask *task = [session dataTaskWithRequest:request completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) { if (error) { // Handle the error NSLog(@"Error: %@", error.localizedDescription); } else { // Process the data NSString *responseString = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding]; NSLog(@"Response: %@", responseString); } }]; [task resume];
In this code, we create a URL, a request with that URL, and then use dataTaskWithRequest:completionHandler: to perform the GET request asynchronously. The completion handler is called when the request is complete, allowing you to handle the response or any errors.
For POST requests, you can create a NSMutableURLRequest and set the HTTP method to “POST.” Then, you can use uploadTaskWithRequest:fromData:completionHandler: to send data to the server.
2.3 Handling Responses and Errors
When the network request is complete, the completion handler is called with three parameters: data, response, and error. You can use these parameters to handle the response data and any errors that may have occurred during the request.
data: Contains the response data received from the server.
response: Represents the HTTP response, including status code and headers.
error: Contains any error information if the request fails.
3. Parsing JSON Data
JSON (JavaScript Object Notation) is a widely used format for data interchange in web services. To work with JSON data in Objective-C, you can use the NSJSONSerialization class.
3.1 Why JSON?
JSON is lightweight, human-readable, and easy to parse. It’s the preferred format for many web APIs because of its simplicity and efficiency.
3.2 Using NSJSONSerialization
To parse JSON data in Objective-C, follow these steps:
Convert the response data into a JSON object using NSJSONSerialization.
Handle any errors that may occur during parsing.
Here’s an example of how to parse JSON data received from a web service:
objective NSError *jsonError; NSDictionary *jsonObject = [NSJSONSerialization JSONObjectWithData:data options:kNilOptions error:&jsonError]; if (jsonError) { // Handle JSON parsing error NSLog(@"JSON Parsing Error: %@", jsonError.localizedDescription); } else { // Process the JSON data NSLog(@"Parsed JSON: %@", jsonObject); }
In this code, JSONObjectWithData:options:error: is used to convert the data into a JSON object. If an error occurs during parsing, you can catch it in the jsonError variable.
4. Asynchronous Networking
Asynchronous networking is crucial in iOS app development to ensure that the user interface remains responsive while network requests are being made. Grand Central Dispatch (GCD) is a powerful tool for managing concurrency in Objective-C.
4.1 GCD (Grand Central Dispatch)
GCD provides a way to perform tasks concurrently without the complexity of managing threads manually. You can use GCD to dispatch tasks to background queues, ensuring that network requests do not block the main UI thread.
Here’s an example of how to perform a network request on a background queue and update the UI on the main queue:
objective dispatch_queue_t backgroundQueue = dispatch_queue_create("com.example.networking", NULL); dispatch_async(backgroundQueue, ^{ // Perform network request // ... dispatch_async(dispatch_get_main_queue(), ^{ // Update UI with the results // ... }); });
In this code, we create a background queue using dispatch_queue_create and use dispatch_async to perform the network request on that queue. Once the request is complete, we use dispatch_get_main_queue() to update the UI on the main queue, ensuring a smooth user experience.
4.2 Avoiding UI Blocking
Blocking the UI thread with synchronous network requests can result in an unresponsive app, leading to a poor user experience. Asynchronous networking and GCD are essential for avoiding UI blocking and maintaining app responsiveness.
5. Best Practices for Objective-C Networking
Effective networking in iOS apps goes beyond writing code that works; it involves following best practices to ensure reliability and security.
5.1 Error Handling
Always handle errors gracefully when making network requests. Check for network connectivity, server availability, and possible timeouts. Use appropriate error codes and messages to provide meaningful feedback to the user.
5.2 Security Concerns
Security is paramount when dealing with networking in apps. Implement secure communication protocols such as HTTPS, avoid hardcoding sensitive information like API keys, and consider using libraries like Keychain Services to store credentials securely.
5.3 Testing and Debugging
Thoroughly test your networking code under various conditions, including poor network connectivity and server failures. Use debugging tools and techniques to identify and fix issues quickly.
Conclusion
In this guide, we’ve explored the fundamentals of Objective-C networking for iOS app development. We started by understanding the importance of networking in iOS apps and why Objective-C is a viable choice for this task. We then delved into NSURLSession for making network requests, parsing JSON data, and ensuring asynchronous networking using GCD. Finally, we discussed best practices for robust and secure networking in Objective-C.
Networking is a critical aspect of iOS app development, and mastering it is essential for building high-quality apps that interact seamlessly with web services. By following the principles and code samples outlined in this guide, you’ll be well-equipped to tackle networking challenges in your iOS projects.
So, what’s next? Dive into Objective-C networking, experiment with different APIs, and keep exploring the ever-evolving world of iOS app development. Happy coding!
Table of Contents
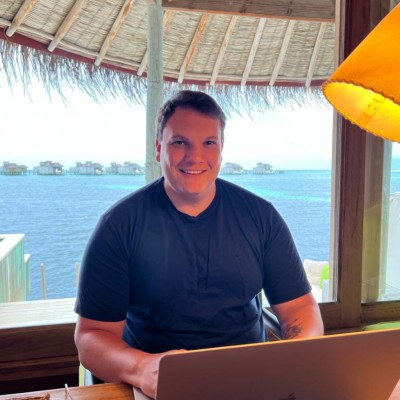
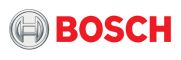