Objective-C Properties: Getters, Setters, and Beyond
Objective-C is a powerful and versatile programming language, especially when it comes to iOS and macOS app development. One of the fundamental concepts in Objective-C is properties. Properties allow you to define the interface for accessing and manipulating object attributes, encapsulating data and providing controlled access to it. In this comprehensive guide, we will delve into Objective-C properties, covering everything from basic getters and setters to advanced techniques that will help you become a more proficient iOS developer.
1. Understanding Properties in Objective-C
Properties are an essential part of object-oriented programming, providing a way to manage the state of objects. In Objective-C, properties are used to define the attributes of a class that can be accessed and modified from outside the class. These attributes can be anything from simple data types like integers and strings to more complex objects.
1.1 Declaring Properties
To declare a property in Objective-C, you use the @property keyword followed by the property’s data type and name. Here’s a basic example:
objective @property (nonatomic, strong) NSString *name;
In this example, we declare a property named name of type NSString. The (nonatomic, strong) part is an attribute that specifies the memory management behavior for this property. The nonatomic attribute means that the property is not thread-safe, while strong indicates that the property retains the assigned value.
1.2 Synthesizing Properties
After declaring a property, you need to synthesize it in your implementation file (.m) using the @synthesize keyword:
objective @synthesize name;
This step generates the getter and setter methods for your property, allowing you to access and modify its value.
2. Getters and Setters
Getters and setters are the two essential methods associated with properties. They are used to read and write property values, respectively.
2.1 Getters
A getter method retrieves the value of a property. In Objective-C, getter methods are automatically generated when you declare a property. For our name property, the getter method would be named name. Here’s how you would use it:
objective NSString *personName = [myPerson name];
Alternatively, you can use dot notation to access the property:
objective NSString *personName = myPerson.name;
2.2 Setters
A setter method assigns a new value to a property. Like getters, setter methods are automatically generated for properties. For our name property, the setter method would be named setName:. Here’s how you would use it:
objective [myPerson setName:@"John"];
Or using dot notation:
objective myPerson.name = @"John";
2.3 Custom Getters and Setters
While Objective-C generates default getters and setters for properties, you can also customize them to add additional logic. For example, you might want to validate input values or perform some actions when setting or getting a property. Here’s how you can define custom getters and setters:
objective - (NSString *)name { // Custom logic goes here return _name; // Assuming you have an instance variable named _name } - (void)setName:(NSString *)newName { // Custom logic goes here _name = newName; // Assuming you have an instance variable named _name }
Custom getters and setters allow you to encapsulate complex behavior within your class while still providing a simple and consistent interface to the outside world.
3. Property Attributes
In addition to the basic nonatomic and strong attributes mentioned earlier, Objective-C provides several other property attributes that allow you to fine-tune the behavior of your properties.
3.1 Readonly and Readwrite
By default, properties are readwrite, meaning they can be both read and written. However, you can make a property readonly by using the readonly attribute. Readonly properties can only be accessed through their getter method, and attempts to set them will result in a compilation error.
objective @property (nonatomic, readonly) NSString *readOnlyProperty;
3.2 Atomic and Nonatomic
The atomic attribute makes property access thread-safe, ensuring that multiple threads can’t interfere with each other when accessing or modifying the property’s value. However, it comes at a performance cost. The nonatomic attribute, as mentioned earlier, makes property access non-thread-safe but is faster.
objective @property (nonatomic, strong) NSString *nonAtomicProperty; @property (atomic, strong) NSString *atomicProperty;
3.3 Copy
The copy attribute is used when you want to ensure that a property holds an immutable copy of the assigned value. This is commonly used for properties that are NSString or other mutable objects to prevent them from being modified inadvertently.
objective @property (nonatomic, copy) NSString *copiedProperty;
3.4 Weak and Strong
The weak attribute is used for properties that should not retain the assigned object. It is commonly used to avoid strong reference cycles (retain cycles) in delegate relationships and other situations where two objects reference each other. The strong attribute, as mentioned earlier, retains the assigned object.
objective @property (nonatomic, weak) NSObject *weakProperty;
3.5 Assign
The assign attribute is used for properties that hold primitive data types like NSInteger or CGFloat. It does not retain or copy the assigned value and is commonly used for properties that don’t own their values.
objective @property (nonatomic, assign) NSInteger integerValue;
3.5 Retain
The retain attribute is used as an alternative to strong and is mainly used in Objective-C code written before the introduction of Automatic Reference Counting (ARC). It indicates that the property should retain the assigned object.
objective @property (nonatomic, retain) NSString *retainedProperty;
3.6 Getter and Setter Methods
You can also customize the names of the generated getter and setter methods using the getter and setter attributes:
objective @property (nonatomic, strong, getter=getFullName, setter=setFullName:) NSString *name;
In this example, the getter method will be named getFullName, and the setter method will be named setFullName:.
4. Advanced Property Techniques
Objective-C properties offer more than just simple getters and setters. Here are some advanced techniques that can help you write cleaner and more efficient code:
4.1 Lazy Initialization
Lazy initialization is a technique where you delay the creation of an object until it’s actually needed. This can be useful for optimizing memory usage in your app. You can implement lazy initialization in your getter method like this:
objective - (NSString *)name { if (!_name) { _name = [[NSString alloc] initWithString:@"Default Name"]; } return _name; }
With lazy initialization, the _name property is only created when it’s accessed for the first time.
4.2 Computed Properties
Computed properties are properties that don’t have a backing instance variable but instead calculate their value based on other properties or data. You can use custom getter methods to implement computed properties. Here’s an example:
objective - (NSString *)fullName { return [NSString stringWithFormat:@"%@ %@", self.firstName, self.lastName]; }
In this example, the fullName property is computed based on the firstName and lastName properties.
4.3 Key-Value Coding (KVC)
Objective-C provides Key-Value Coding (KVC), which allows you to access and modify properties using string-based keys. This can be particularly handy when working with dictionaries and dynamic data structures. To enable KVC for a property, you need to use the @synthesize keyword with the property name.
objective @synthesize name = _name;
With this setup, you can access and modify the name property using strings:
objective [myPerson setValue:@"John" forKey:@"name"]; NSString *personName = [myPerson valueForKey:@"name"];
4.4 Property Observers
Property observers allow you to monitor changes to a property and take action when the property’s value is set or modified. You can use the willChangeValueForKey: and didChangeValueForKey: methods to implement property observers:
objective - (void)setName:(NSString *)newName { [self willChangeValueForKey:@"name"]; _name = newName; [self didChangeValueForKey:@"name"]; }
Property observers are particularly useful for implementing features like automatic UI updates when a property changes.
Conclusion
Objective-C properties are a fundamental concept in iOS and macOS app development. They provide a clean and efficient way to manage object attributes, encapsulate data, and control access to it. Understanding how to declare, synthesize, and customize properties, as well as how to use advanced techniques like lazy initialization and computed properties, is essential for becoming a proficient Objective-C developer.
In this guide, we’ve covered the basics of Objective-C properties, including getters and setters, and explored various property attributes that allow you to fine-tune their behavior. We’ve also introduced advanced property techniques like lazy initialization, computed properties, Key-Value Coding, and property observers. Armed with this knowledge, you’re well-equipped to make the most of properties in your Objective-C projects, creating more robust and maintainable code. Happy coding!
Table of Contents
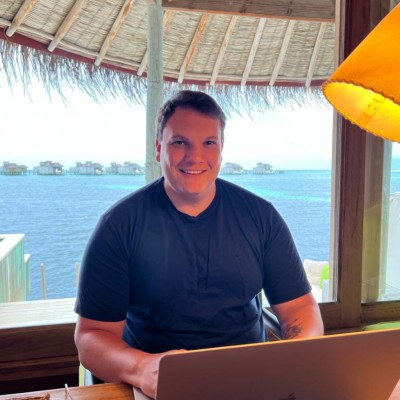
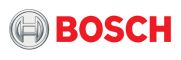