Objective-C Runtime: Exploring the Hidden Power of iOS Development
In the realm of iOS development, Objective-C has been a fundamental programming language for many years. It offers a wide range of features and capabilities that have enabled developers to build robust and innovative applications. One of the hidden gems within Objective-C is its powerful runtime system. Understanding and harnessing the potential of Objective-C Runtime can greatly enhance your iOS development skills and enable you to create more dynamic and flexible applications.
What is Objective-C Runtime?
At its core, Objective-C Runtime is a set of functions and data structures that forms the foundation of the Objective-C language. It is responsible for dynamic message dispatch, method swizzling, introspection, and other powerful features that make Objective-C a highly dynamic language. The runtime system allows you to perform tasks such as adding or modifying methods at runtime, changing class behavior dynamically, and even creating new classes programmatically.
Dynamic Method Dispatch
Dynamic method dispatch is one of the key features provided by Objective-C Runtime. Unlike static languages, where method calls are resolved at compile-time, Objective-C allows for dynamic resolution of method invocations at runtime. This means that you can send messages to objects and have them decide which method to execute based on the current state of the object.
Consider the following example:
objective @interface MyObject : NSObject - (void)doSomething; @end @implementation MyObject - (void)doSomething { NSLog(@"Doing something..."); } @end int main(int argc, char *argv[]) { MyObject *obj = [[MyObject alloc] init]; [obj doSomething]; return 0; }
In this example, the method doSomething is called on an instance of MyObject. At runtime, the Objective-C Runtime system determines which implementation of doSomething to execute based on the class of the object. This dynamic dispatch mechanism allows for great flexibility and extensibility in your code.
Method Swizzling
Method swizzling is another powerful feature enabled by Objective-C Runtime. It allows you to exchange the implementation of two methods dynamically at runtime. This technique can be useful in scenarios where you need to modify the behavior of existing classes without subclassing or making changes to their original implementation.
Here’s an example of method swizzling in action:
objective #import <objc/runtime.h> @interface MyObject : NSObject - (void)originalMethod; @end @implementation MyObject - (void)originalMethod { NSLog(@"Original method implementation"); } @end @interface MyObject (Swizzle) - (void)swizzledMethod; @end @implementation MyObject (Swizzle) - (void)swizzledMethod { NSLog(@"Swizzled method implementation"); [self swizzledMethod]; // Calling the original implementation } @end int main(int argc, char *argv[]) { MyObject *obj = [[MyObject alloc] init]; [obj originalMethod]; // Prints "Original method implementation" // Swizzling Method originalMethod = class_getInstanceMethod([MyObject class], @selector(originalMethod)); Method swizzledMethod = class_getInstanceMethod([MyObject class], @selector(swizzledMethod)); method_exchangeImplementations(originalMethod, swizzledMethod); [obj originalMethod]; // Prints "Swizzled method implementation" return 0; }
In this example, we define a class MyObject with an originalMethod. We then extend the class using a category to add a swizzledMethod. Using the method_exchangeImplementations function from the Objective-C Runtime, we swap the implementations of the two methods. As a result, when we invoke originalMethod, it executes the swizzled implementation, and vice versa. Method swizzling can be a powerful tool for debugging, adding logging, or even modifying the behavior of third-party classes.
Introspection
Objective-C Runtime also provides powerful introspection capabilities that allow you to inspect and manipulate classes and objects at runtime. You can dynamically query classes for their properties, methods, and protocols, and even create new classes programmatically. This opens up possibilities for building flexible frameworks, implementing serialization and deserialization mechanisms, and much more.
Here’s a simple example of using Objective-C Runtime for introspection:
objective #import <objc/runtime.h> @interface MyClass : NSObject @property (nonatomic, strong) NSString *name; - (void)sayHello; @end @implementation MyClass - (void)sayHello { NSLog(@"Hello, %@", self.name); } @end int main(int argc, char *argv[]) { MyClass *obj = [[MyClass alloc] init]; obj.name = @"John Doe"; // Introspection Class myClass = [obj class]; unsigned int propertyCount; objc_property_t *properties = class_copyPropertyList(myClass, &propertyCount); for (unsigned int i = 0; i < propertyCount; i++) { objc_property_t property = properties[i]; const char *propertyName = property_getName(property); NSLog(@"Property: %s", propertyName); } free(properties); return 0; }
In this example, we create a class MyClass with a single property name and a method sayHello. At runtime, we use the class_copyPropertyList function to retrieve the list of properties for the MyClass class and log their names. This introspection capability can be useful for various scenarios, such as automatic object mapping, dependency injection, and more.
Conclusion
Objective-C Runtime is a powerful tool that empowers iOS developers to create dynamic, flexible, and innovative applications. By understanding and utilizing the features provided by the runtime system, you can push the boundaries of iOS development and enhance your app’s capabilities. From dynamic method dispatch and method swizzling to introspection, Objective-C Runtime opens up a world of possibilities for iOS development. So, dive into the Objective-C Runtime and unlock the hidden power of iOS development.
Table of Contents
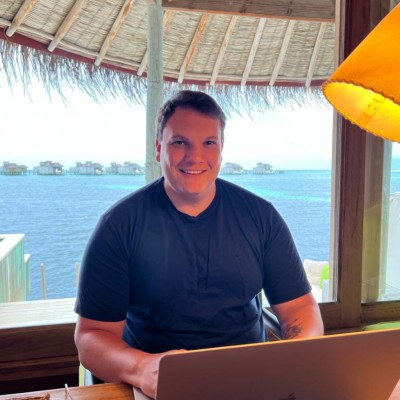
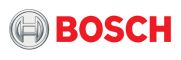