Laravel and PHP: A Perfect Match for Web Development
In the vast landscape of web development frameworks and programming languages, Laravel and PHP stand out as a perfect match for building robust and scalable web applications. Laravel, a popular PHP framework, provides developers with a clean and elegant syntax, a rich set of features, and excellent community support. This blog aims to explore why Laravel and PHP form an unbeatable combination and how they empower developers to create dynamic and feature-rich web applications. We’ll delve into the core features of Laravel and showcase some code samples to illustrate the power of this powerful duo.
The PHP Advantage
To truly appreciate the strength of the Laravel and PHP combination, it’s crucial to understand the advantages that PHP brings to the table. PHP, an open-source scripting language designed specifically for web development, has been the backbone of countless successful projects. Here are some reasons why PHP is a popular choice for developers:
- Easy to Learn and Use: PHP has a gentle learning curve, making it accessible to beginners. Its syntax is similar to other C-style languages, making it easier for developers to switch to PHP.
- Vast Community Support: PHP boasts a massive and active community of developers who contribute to the language’s growth. This active ecosystem ensures regular updates, bug fixes, and a vast array of libraries and frameworks.
- Versatile and Scalable: PHP is highly versatile, allowing developers to build web applications ranging from simple websites to complex enterprise-level systems. It also scales well, making it suitable for projects of any size.
Introducing Laravel
Laravel, created by Taylor Otwell in 2011, quickly gained popularity for its intuitive syntax, expressive code, and comprehensive features. Let’s dive into some key features that make Laravel an exceptional choice for web development:
- Elegant Syntax: Laravel follows the “Convention over Configuration” principle, providing developers with a clean and expressive syntax. It simplifies common tasks and reduces boilerplate code, resulting in faster development cycles.
- MVC Architecture: Laravel embraces the Model-View-Controller (MVC) architectural pattern, separating application logic from presentation. This separation enhances code maintainability, reusability, and testability.
- Routing and Middleware: Laravel’s powerful routing system allows developers to define clean and SEO-friendly URLs effortlessly. Middleware enables developers to handle HTTP requests at various stages, enhancing security, authentication, and request processing.
Let’s take a closer look at some code samples to see Laravel’s syntax in action:
php // Define a route that responds to a GET request Route::get('/products', function () { return view('products.index'); });
In this example, we define a route that maps the “/products” URL to a function that returns a view named “products.index.” Laravel’s expressive syntax makes it easy to define routes and associate them with corresponding actions.
php // Retrieve a collection of products from the database $products = Product::where('category', 'electronics') ->orderBy('price', 'desc') ->get();
This code snippet showcases how Laravel simplifies database operations. Using the Eloquent ORM, developers can query the database in an elegant and expressive manner, resulting in cleaner code.
Database and Eloquent ORM
Laravel’s database layer is powered by the Eloquent ORM, which provides an intuitive and expressive way to interact with databases. Eloquent simplifies database operations, offering a fluent and object-oriented interface. Let’s see how Eloquent simplifies working with databases:
php // Define a Product model class Product extends Eloquent { // Define the table associated with the model protected $table = 'products'; } // Retrieve a single product by ID $product = Product::find(1); // Update a product's price $product->price = 29.99; $product->save();
In this example, we define a Product model that represents a database table. Using Eloquent, we can easily retrieve a specific product by its ID and update its price. Eloquent’s simplicity and powerful querying capabilities make it a go-to choice for working with databases.
Powerful Caching Mechanisms
Caching plays a crucial role in web application performance. Laravel offers seamless integration with various caching systems, including popular choices like Redis and Memcached. Let’s see how Laravel’s caching mechanisms work:
php // Retrieve data from the cache, or store it if not present $products = Cache::remember('all_products', 3600, function () { return Product::all(); });
In this code snippet, we use Laravel’s caching feature to store the result of a database query. The first time this code is executed, Laravel retrieves the products from the database and caches them for one hour. Subsequent calls within the hour fetch the data directly from the cache, significantly reducing database load and improving performance.
Authentication and Authorization
Building secure web applications often involves implementing authentication and authorization mechanisms. Laravel provides a robust authentication system right out of the box, making it effortless to secure your applications. Here’s an example of how Laravel simplifies authentication:
php // Register a new user $user = new User(); $user->name = 'John Doe'; $user->email = 'john@example.com'; $user->password = Hash::make('secret'); $user->save(); // Log in a user if (Auth::attempt(['email' => $email, 'password' => $password])) { // Authentication successful } else { // Authentication failed }
In this example, we demonstrate user registration and authentication using Laravel’s built-in features. Laravel handles the hashing of passwords, user session management, and authentication checks, saving developers valuable time and effort.
Robust Testing Framework
Laravel’s commitment to testing is evident through its robust testing framework. It provides various testing utilities, including PHPUnit integration, making it effortless to write and execute tests. Laravel’s testing framework facilitates unit tests, feature tests, and API tests, ensuring that your application remains reliable and bug-free.
Conclusion
Laravel and PHP form a perfect match for web development, providing developers with a powerful and efficient framework backed by a popular and versatile programming language. With Laravel’s expressive syntax, comprehensive features, and the vast PHP ecosystem, developers can build dynamic, scalable, and feature-rich web applications. Whether you’re a beginner or an experienced developer, Laravel and PHP empower you to create outstanding web applications with ease.
So, embrace the synergy of Laravel and PHP, and unlock endless possibilities in your web development journey.
Table of Contents
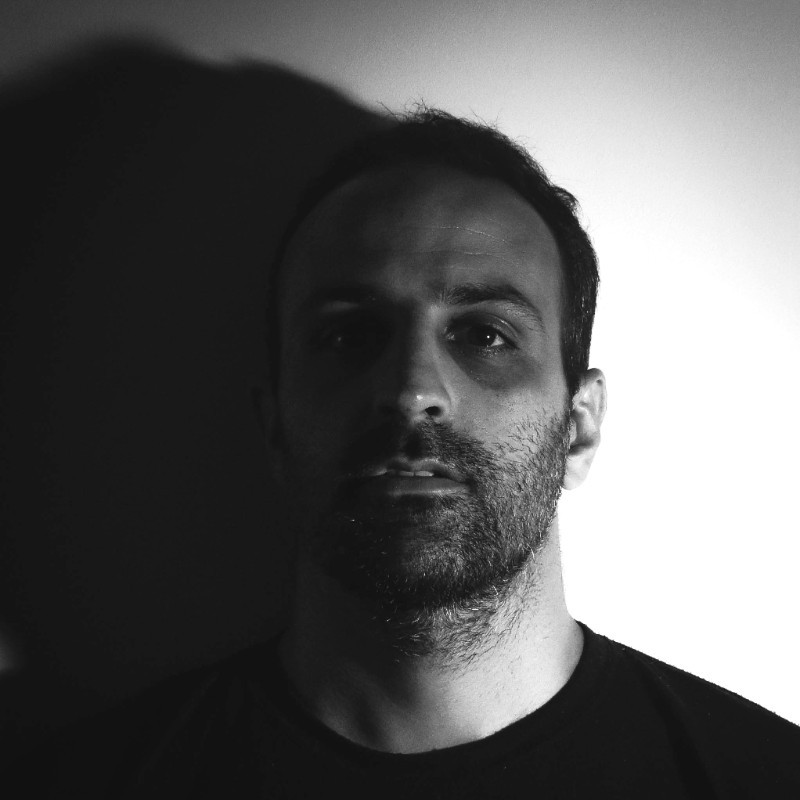
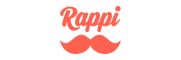