The Power of PHP’s array_chunk() Function
PHP, a versatile and widely-used scripting language, offers an array of functions to simplify complex tasks. One such function that often goes unnoticed but is immensely powerful is array_chunk(). This unsung hero of PHP can make your array manipulation tasks a breeze, improving the efficiency and readability of your code. In this blog, we will delve into the depths of array_chunk() and explore its capabilities, use cases, and real-world examples to help you harness its full potential.
Table of Contents
1. Understanding array_chunk()
Before we dive into practical examples, let’s first understand what the array_chunk() function does. At its core, array_chunk() divides an array into smaller arrays of a specified size. This division is particularly useful when dealing with large datasets, pagination, or when you need to split data into manageable chunks for processing.
1.1. The Syntax
The syntax of array_chunk() is quite straightforward:
php array_chunk(array $input, int $size, bool $preserve_keys = false): array
- $input: The input array you want to divide.
- $size: The size of each chunk.
- $preserve_keys (optional): A flag to indicate whether to preserve the keys in the chunked arrays. Default is false.
Now, let’s explore some practical scenarios where array_chunk() can be a game-changer.
2. Pagination Made Easy
One of the most common use cases for array_chunk() is implementing pagination for large datasets. Imagine you have an array of hundreds or even thousands of items, and you want to display them in smaller, more manageable chunks across multiple pages. array_chunk() can make this task a walk in the park.
Let’s take a look at a simplified example:
php $items = range(1, 100); // Create an array of 100 items $perPage = 10; // Number of items per page $pages = array_chunk($items, $perPage); // Now, let's say we want to display page 3 $pageNumber = 3; if (isset($pages[$pageNumber - 1])) { $currentPageItems = $pages[$pageNumber - 1]; foreach ($currentPageItems as $item) { echo $item . "\n"; } }
In this example, we create an array of 100 items and use array_chunk() to split them into chunks of 10 items each. To display a specific page (in this case, page 3), we access the corresponding chunk from the $pages array. This approach makes pagination code concise and easy to manage.
3. Processing Data in Batches
Suppose you have a task that involves processing a large dataset, and you want to do it in smaller, more manageable batches to avoid memory issues. array_chunk() can come to the rescue again.
Here’s a hypothetical scenario where you want to process user data in batches of 50:
php $users = getUsersFromDatabase(); // Fetch all user data $batchSize = 50; // Number of users to process in each batch $userBatches = array_chunk($users, $batchSize); foreach ($userBatches as $batch) { processUserData($batch); }
In this example, we retrieve user data from a database and split it into batches of 50 users each using array_chunk(). We can then process each batch separately, ensuring that the script doesn’t run into memory problems while dealing with a large dataset.
4. Creating Multidimensional Arrays
array_chunk() isn’t limited to breaking an array into chunks; it can also transform a single-dimensional array into a multidimensional one. This can be handy when dealing with tabular data or preparing data for display.
Consider the following example:
php $data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; $columns = 2; $table = array_chunk($data, $columns); print_r($table);
In this case, we have a one-dimensional array containing numbers from 1 to 10, and we use array_chunk() to split it into an array with 2 columns. The resulting $table variable will hold a multidimensional array like this:
php Array ( [0] => Array ( [0] => 1 [1] => 2 ) [1] => Array ( [0] => 3 [1] => 4 ) [2] => Array ( [0] => 5 [1] => 6 ) [3] => Array ( [0] => 7 [1] => 8 ) [4] => Array ( [0] => 9 [1] => 10 ) )
This transformation is particularly useful when you need to structure data for tabular displays or export it to a format like JSON or CSV.
5. Preserving Keys
By default, array_chunk() resets the keys in the resulting chunked arrays. However, you can preserve the keys by setting the optional $preserve_keys parameter to true. This can be helpful when you want to maintain the association between keys and values.
Let’s illustrate this with an example:
php $data = ['a' => 1, 'b' => 2, 'c' => 3, 'd' => 4, 'e' => 5]; $size = 2; $chunks = array_chunk($data, $size, true); print_r($chunks);
With $preserve_keys set to true, the output will be:
php Array ( [0] => Array ( [a] => 1 [b] => 2 ) [1] => Array ( [c] => 3 [d] => 4 ) [2] => Array ( [e] => 5 ) )
Preserving keys ensures that you can retain the original data structure, which may be crucial for certain operations.
6. Real-World Applications
Now that we’ve explored various scenarios where array_chunk() can be useful, let’s take a look at some real-world applications where this function shines.
6.1. Image Gallery Thumbnails
Imagine you’re building an image gallery, and you want to display thumbnail images in rows with a specified number of columns. You can use array_chunk() to organize your image data for rendering.
Here’s a simplified example:
php $images = getImagePathsFromDirectory(); // Get image paths from a directory $columns = 4; // Number of columns for the gallery $imageRows = array_chunk($images, $columns); foreach ($imageRows as $row) { echo '<div class="row">'; foreach ($row as $imagePath) { echo '<div class="column"><img src="' . $imagePath . '"></div>'; } echo '</div>'; }
In this case, array_chunk() helps you organize image paths into rows with the desired number of columns, making it easy to generate an attractive and responsive image gallery.
6.2. Bulk Email Processing
Suppose you’re working on a system that sends bulk emails to a large list of subscribers. To ensure smooth processing and avoid overloading your email server, you can use array_chunk() to send emails in smaller batches.
Here’s a simplified example:
php $subscribers = getSubscriberEmails(); // Get a list of subscriber emails $batchSize = 100; // Number of emails to send in each batch $emailBatches = array_chunk($subscribers, $batchSize); foreach ($emailBatches as $batch) { sendBulkEmail($batch); }
By chunking the subscriber list, you can efficiently manage the email sending process, reducing the risk of server overload and ensuring that all subscribers receive their emails.
Conclusion
PHP’s array_chunk() function is a versatile and valuable tool that can simplify various array manipulation tasks. Whether you’re implementing pagination, processing data in batches, restructuring data for display, or tackling other array-related challenges, array_chunk() can help you achieve your goals with elegance and efficiency.
By understanding how to use array_chunk() effectively and exploring its real-world applications, you can elevate your PHP coding skills and produce cleaner, more maintainable code. So, the next time you find yourself working with arrays in PHP, don’t forget to harness the power of array_chunk() to make your life as a developer easier and more productive. Happy coding!
Table of Contents
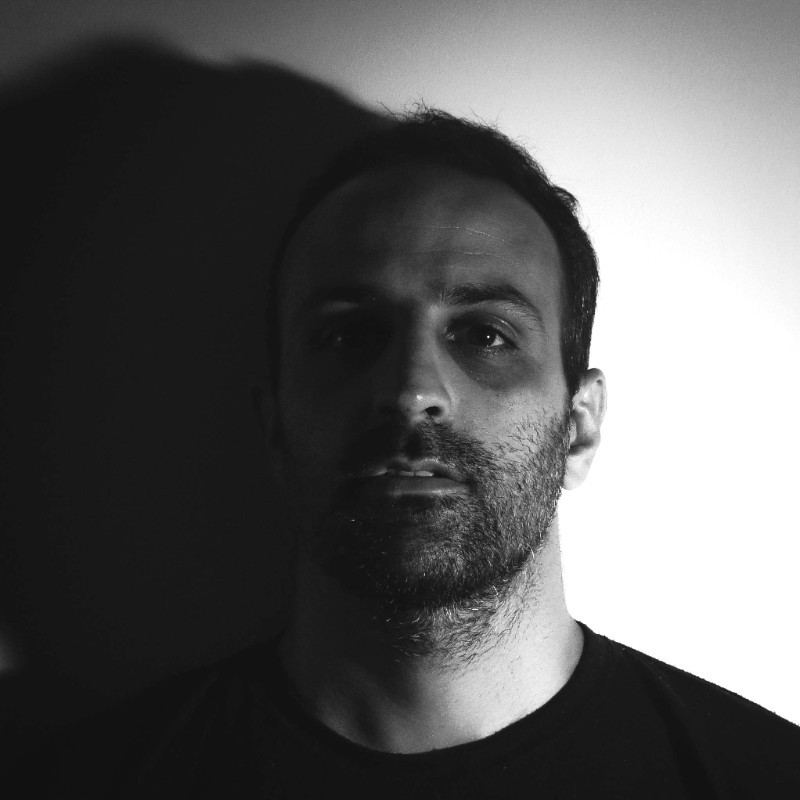
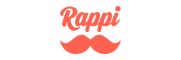