Unlocking PHP’s array_column() Function: A Practical Guide
PHP is a versatile and powerful programming language used in web development to create dynamic and interactive websites. One of its many features is the array_column() function, which simplifies the task of extracting data from multidimensional arrays. In this comprehensive guide, we’ll explore the ins and outs of array_column(), provide practical examples, and show you how to leverage this function to make your PHP code more efficient and readable.
Table of Contents
1. Understanding Multidimensional Arrays
Before diving into array_column(), it’s crucial to grasp the concept of multidimensional arrays. In PHP, an array can contain other arrays as its elements, forming a nested structure. This hierarchical arrangement of data is useful for representing complex data sets, such as database results or JSON data.
2. What is a Multidimensional Array?
A multidimensional array is an array that contains other arrays as its elements. Each sub-array can, in turn, contain additional arrays, creating a nested structure. This allows you to organize and represent data in a way that mirrors real-world relationships.
Let’s illustrate this with a simple example:
php $students = [ [ 'name' => 'Alice', 'age' => 21, 'grades' => [95, 87, 92], ], [ 'name' => 'Bob', 'age' => 19, 'grades' => [88, 76, 94], ], [ 'name' => 'Charlie', 'age' => 20, 'grades' => [91, 89, 93], ], ];
In this example, the $students array is multidimensional. Each element of the $students array is an associative array representing a student with their name, age, and an array of grades.
3. Introducing array_column()
The array_column() function in PHP is a versatile tool for extracting data from multidimensional arrays. It allows you to extract a specific column or field from each sub-array within a larger array. This can be incredibly useful when working with data that is organized in a tabular or grid-like structure.
3.1. Syntax of array_column()
The basic syntax of array_column() is as follows:
php array_column(array $array, mixed $column_key, mixed $index_key = null);
- $array: The input multidimensional array from which you want to extract data.
- $column_key: The column or field you want to extract from each sub-array.
- $index_key (optional): An optional field used as the index or keys for the resulting array.
Let’s break down these parameters with some practical examples.
4. Extracting a Single Column
Suppose you have the following multidimensional array representing a list of products:
php $products = [ ['id' => 1, 'name' => 'Widget A', 'price' => 19.99], ['id' => 2, 'name' => 'Widget B', 'price' => 24.99], ['id' => 3, 'name' => 'Widget C', 'price' => 29.99], ];
You can use array_column() to extract just the names of the products:
php $productNames = array_column($products, 'name');
The resulting $productNames array will contain the product names:
php ['Widget A', 'Widget B', 'Widget C']
5. Extracting a Column with Index Keys
You can also use array_column() to extract a specific column while preserving the original array’s keys. For instance, if you want to create an associative array where the product IDs are the keys and the product names are the values:
php $productNamesWithIds = array_column($products, 'name', 'id');
The resulting $productNamesWithIds array will look like this:
php [ 1 => 'Widget A', 2 => 'Widget B', 3 => 'Widget C', ]
6. Practical Use Cases
Now that you understand the basic usage of array_column(), let’s explore some practical use cases where this function can simplify your code and improve readability.
6.1. Database Query Results
When working with database queries, it’s common to receive results as multidimensional arrays. Consider a scenario where you retrieve a list of users from a database:
php // Simulated database query result $users = [ ['id' => 1, 'username' => 'alice', 'email' => 'alice@example.com'], ['id' => 2, 'username' => 'bob', 'email' => 'bob@example.com'], // ... ];
If you only need an array of usernames for some task, you can quickly extract them using array_column():
php $usernames = array_column($users, 'username');
6.2. Working with JSON Data
When dealing with JSON data, you often parse it into PHP arrays. Let’s say you have JSON data representing a list of books:
php $jsonData = '[ {"title": "The Great Gatsby", "author": "F. Scott Fitzgerald"}, {"title": "To Kill a Mockingbird", "author": "Harper Lee"}, // ... ]'; $books = json_decode($jsonData, true);
Now, if you want to extract all book titles into an array, array_column() can simplify the process:
php $titles = array_column($books, 'title');
7. Handling Nested Arrays
array_column() can handle nested arrays as well. Consider the following example:
php $students = [ [ 'name' => 'Alice', 'grades' => [ 'math' => 95, 'science' => 87, 'history' => 92, ], ], [ 'name' => 'Bob', 'grades' => [ 'math' => 88, 'science' => 76, 'history' => 94, ], ], ];
If you want to extract the math grades of each student, you can specify the nested array key as the $column_key:
php $mathGrades = array_column($students, 'grades', 'name');
The resulting $mathGrades array will look like this:
php [ 'Alice' => ['math' => 95, 'science' => 87, 'history' => 92], 'Bob' => ['math' => 88, 'science' => 76, 'history' => 94], ]
8. Handling Missing Keys
You may encounter situations where some sub-arrays do not contain the specified column or key. In such cases, array_column() simply skips those sub-arrays, resulting in a shorter output array.
For example, consider the following multidimensional array:
php $data = [ ['id' => 1, 'name' => 'Alice'], ['id' => 2, 'age' => 25], ['id' => 3, 'name' => 'Charlie'], ];
If you try to extract the ‘name’ column from this array:
php $names = array_column($data, 'name');
The resulting $names array will only contain the ‘name’ values where available:
php ['Alice', 'Charlie']
9. Handling Numeric Index Keys
By default, array_column() uses numeric index keys for the resulting array when you don’t specify the $index_key parameter. This can be useful in scenarios where you want to re-index your data numerically.
Consider the following array:
php $fruits = ['apple', 'banana', 'cherry'];
If you use array_column() without an $index_key, it will re-index the resulting array numerically:
php $selectedFruits = array_column($fruits, null);
The resulting $selectedFruits array will be:
php [0 => 'apple', 1 => 'banana', 2 => 'cherry']
10. Combining array_column() with Other Functions
array_column() is often used in combination with other array functions to perform more complex operations. For example, you can use it to calculate the average grade of students:
php $mathGrades = array_column($students, 'grades.math'); $averageMathGrade = array_sum($mathGrades) / count($mathGrades);
In this example, array_column() extracts the math grades, and array_sum() calculates their sum. Dividing by the count of grades gives you the average.
Conclusion
In this practical guide, we’ve explored the power of PHP’s array_column() function. You’ve learned how to extract data from multidimensional arrays effortlessly, making your PHP code more efficient and readable. Whether you’re working with database query results, JSON data, or any other multidimensional array, array_column() is a valuable tool in your PHP toolkit.
As you continue to develop PHP applications, remember that understanding and utilizing array functions like array_column() can significantly improve your code’s maintainability and simplicity. Experiment with different scenarios and explore the flexibility this function offers in handling various data structures. With array_column() in your arsenal, you’ll be better equipped to tackle complex data manipulation tasks in your PHP projects.
Table of Contents
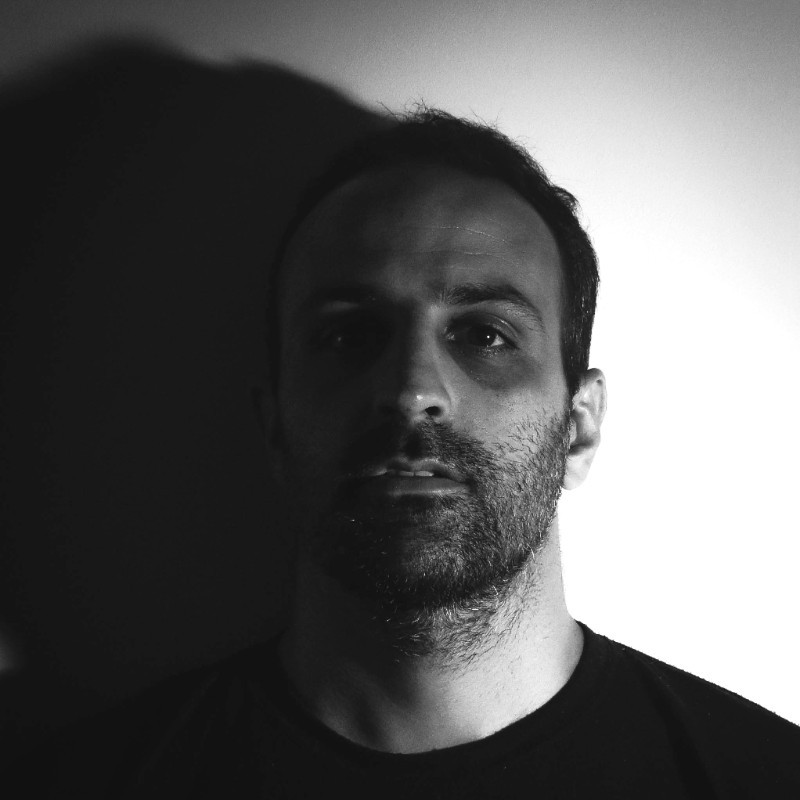
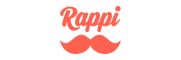