PHP’s array_flip() Function: A Comprehensive Look
Arrays are a fundamental data structure in PHP, serving as versatile containers for storing and manipulating data. One of the many built-in array functions that PHP offers is array_flip(). In this comprehensive guide, we’ll take an in-depth look at the array_flip() function, including its purpose, usage, benefits, and limitations. Along the way, we’ll explore practical examples and scenarios where this function can streamline your coding tasks.
Table of Contents
1. Understanding the Purpose of array_flip()
To grasp the significance of array_flip(), let’s begin by understanding its core purpose. This function is primarily used for switching the keys and values within an associative array. In simpler terms, it flips the array, making the keys become values and vice versa.
This reversal of key-value pairs can be incredibly useful in various programming situations. Whether you need to quickly search for values based on keys or transform data for specific output requirements, array_flip() can be your go-to solution.
1.1. Syntax and Basic Usage
Before diving into advanced use cases, let’s get familiar with the basic syntax and usage of array_flip().
php flipped_array = array_flip(original_array);
Here, original_array is the input array that you want to flip, and flipped_array is the resulting array with keys and values swapped.
2. Benefits of Using array_flip()
2.1. Efficient Key-Value Lookup
One of the primary benefits of array_flip() is its ability to enhance key-value lookups. Imagine you have an array that represents a mapping of user IDs to usernames:
php $usernames = [ 1 => 'alice', 2 => 'bob', 3 => 'charlie' ];
If you need to find the username associated with a specific user ID, you can use array_flip() to quickly switch the keys and values:
php $flipped_usernames = array_flip($usernames); $username = $flipped_usernames[2]; // Retrieves 'bob'
By flipping the array, you create a reverse mapping, making it efficient to retrieve values based on keys.
2.2. Removing Duplicate Values
Another valuable use case for array_flip() is eliminating duplicate values from an array. When you flip an array, duplicate values become keys, and PHP automatically removes duplicates. Here’s an example:
php $fruits = [ 'apple', 'banana', 'cherry', 'banana', 'date' ]; $unique_fruits = array_flip(array_flip($fruits)); print_r($unique_fruits);
In this code, we first flip the array to turn duplicate values into keys and then flip it back. The result is an array with unique values:
php Array ( [0] => apple [1] => banana [2] => cherry [4] => date )
This technique provides a concise way to deduplicate an array without requiring extensive loops or complex logic.
2.3. Preparing Data for SQL Queries
When working with databases, you may encounter scenarios where you need to construct SQL queries with dynamic conditions based on an array of values. array_flip() can be invaluable for this purpose. Consider the following example:
php $colors = [ 'red', 'green', 'blue' ]; $color_filter = "'" . implode("', '", array_flip($colors)) . "'"; $query = "SELECT * FROM products WHERE color IN ($color_filter)";
In this example, array_flip() is used to flip the colors array, creating a mapping of values to keys. This allows for easy construction of the SQL IN clause, ensuring that the query retrieves products with matching colors efficiently.
3. Limitations and Considerations
While array_flip() is a powerful tool, it’s essential to be aware of its limitations and potential pitfalls:
3.1. Unique Values Only
array_flip() works best when the original array contains unique values. If your array has duplicate values, flipping it will result in data loss, as duplicate values will be merged into a single key. To handle arrays with duplicate values, consider using alternative methods, such as looping through the array and creating a new associative array.
3.2. Numeric Values
When flipping an array with numeric values, be cautious about potential type coercion. PHP will cast numeric keys to integers when using array_flip(). This can lead to unexpected behavior if you have keys like ‘012’ and ’12’, as they will be treated as the same key after flipping.
3.3. Performance Considerations
While array_flip() is efficient for small to moderately sized arrays, it may not be the best choice for extremely large datasets. In such cases, the memory usage and execution time of the function can become a concern. Always consider the size of your data and the available system resources when using array_flip().
4. Practical Examples
Let’s explore some practical examples to see how array_flip() can be applied in real-world scenarios.
Example 1: Reversing a Dictionary
Suppose you have a dictionary of English words and their corresponding definitions:
php $dictionary = [ 'apple' => 'a round fruit with red or green skin and white flesh', 'banana' => 'a long, curved fruit with yellow skin', 'cherry' => 'a small, round fruit with a red or pink skin' ];
You can easily reverse this dictionary using array_flip():
php $reversed_dictionary = array_flip($dictionary); print_r($reversed_dictionary);
The output will be:
php Array ( [a round fruit with red or green skin and white flesh] => apple [a long, curved fruit with yellow skin] => banana [a small, round fruit with a red or pink skin] => cherry )
This reversed dictionary can be handy for looking up word definitions based on descriptions.
Example 2: Counting Occurrences
Let’s say you have an array of colors representing the preferences of website visitors:
php $preferences = ['blue', 'green', 'red', 'blue', 'green', 'yellow'];
You want to count the occurrences of each color efficiently. Here’s how you can do it using array_flip():
php $flipped_preferences = array_flip($preferences); $color_counts = array_count_values($flipped_preferences); print_r($color_counts);
The output will show the count of each color:
php Array ( [blue] => 2 [green] => 2 [red] => 1 [yellow] => 1 )
Example 3: Generating Dropdown Options
In web development, you often need to populate dropdown lists with options retrieved from a database or other data source. Consider an array of countries that you want to use as options in an HTML <select> element:
php $countries = [ 'US' => 'United States', 'CA' => 'Canada', 'AU' => 'Australia', 'UK' => 'United Kingdom' ];
To generate the HTML for the dropdown, you can use array_flip():
php $options = array_flip($countries); foreach ($options as $value => $label) { echo "<option value='$value'>$label</option>"; }
This code will create a list of options with the country codes as values and the full country names as labels.
Conclusion
PHP’s array_flip() function is a versatile tool that allows you to switch keys and values within an associative array efficiently. Whether you need to enhance key-value lookups, remove duplicates, or prepare data for SQL queries, array_flip() can simplify your coding tasks. However, it’s crucial to be aware of its limitations and use it judiciously, especially with large datasets and arrays containing numeric or duplicate values.
By mastering array_flip() and incorporating it into your PHP development toolbox, you can streamline your code and improve your programming efficiency. Experiment with different use cases to discover how this function can benefit your projects, making your code more concise and maintainable.
In the world of PHP programming, array_flip() is undoubtedly a valuable function to have at your disposal.
Table of Contents
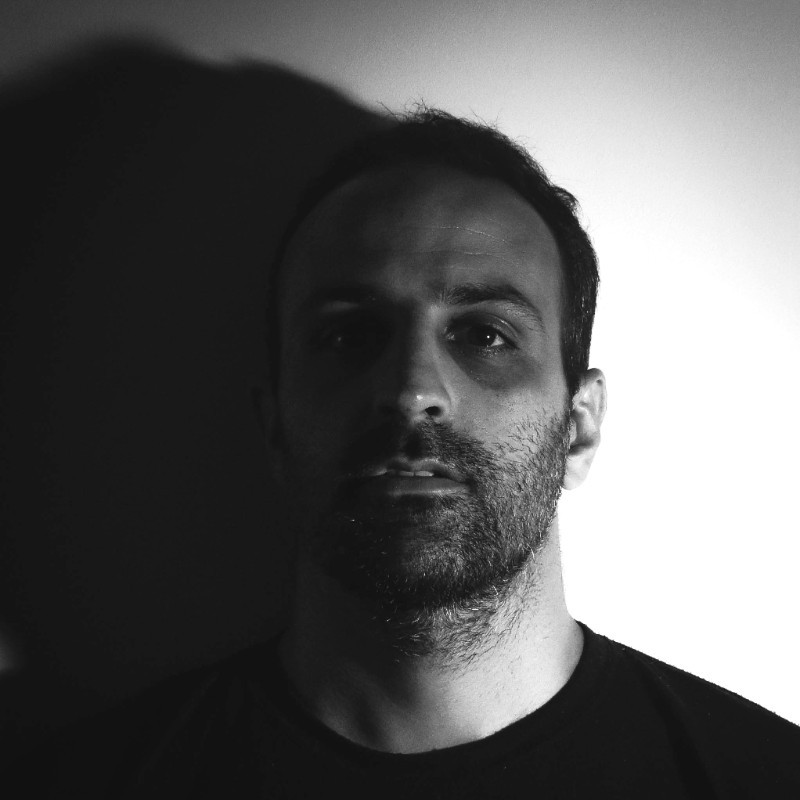
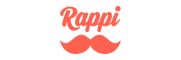