The Magic of PHP’s array_key_exists() Function
PHP, the popular server-side scripting language, is known for its array manipulation capabilities. Among its array-related functions, array_key_exists() stands out as a versatile tool that can work wonders in various scenarios. This magical function allows you to check whether a specific key exists within an array, making it a valuable addition to any PHP developer’s toolkit. In this blog, we’ll delve into the mystical realm of array_key_exists(), exploring its features, use cases, and real-world examples.
Table of Contents
1. Unveiling the Basics
Before we unravel the full potential of array_key_exists(), let’s start with the fundamentals.
1.1. What is array_key_exists()?
array_key_exists() is a built-in PHP function that checks if a given key exists within an array. It returns true if the key is found and false otherwise. This function takes two parameters:
- Key to Check: The first parameter is the key you want to verify within the array.
- Array to Search: The second parameter is the array you want to search for the key in.
Now that we’ve introduced the function, let’s see it in action.
1.2. A Simple Example
Consider the following array:
php $userInfo = [ 'username' => 'john_doe', 'email' => 'john@example.com', 'age' => 30, ]; $keyToCheck = 'email'; if (array_key_exists($keyToCheck, $userInfo)) { echo "The key '$keyToCheck' exists in the array."; } else { echo "The key '$keyToCheck' does not exist in the array."; }
In this example, we define an array $userInfo containing information about a user and then use array_key_exists() to check if the key ’email’ exists within the array. When you run this code, it will output: “The key ’email’ exists in the array.”
1.3. Return Values
As mentioned earlier, array_key_exists() returns true if the key is found and false if it is not. This return value is incredibly useful for making conditional decisions in your code, as demonstrated in the previous example.
2. The Magic Unleashed: Use Cases
Now that we’ve covered the basics, let’s explore the various scenarios in which array_key_exists() can work its magic.
2.1. Validating User Input
When dealing with user input, such as form submissions, it’s crucial to ensure that the expected keys exist in the input data. array_key_exists() can help you validate user-provided data efficiently.
php // Assume $formData is an array of user-submitted data $expectedKeys = ['username', 'email', 'password']; foreach ($expectedKeys as $key) { if (!array_key_exists($key, $formData)) { die("Required key '$key' is missing in the submitted data."); } } // Continue processing the validated data
In this example, we define an array of expected keys ($expectedKeys) and loop through them, using array_key_exists() to check if each key exists in the user-submitted data ($formData). If any required key is missing, the script terminates with an error message. This ensures that only valid data is processed further.
2.2. Configurations and Settings
Many PHP applications rely on configuration arrays to manage settings. With array_key_exists(), you can easily fetch and validate configuration values based on user-defined keys.
php // Configuration settings $config = [ 'site_title' => 'My Website', 'admin_email' => 'admin@example.com', 'debug_mode' => false, ]; // Function to get a configuration value function getConfigValue($key, $config) { if (array_key_exists($key, $config)) { return $config[$key]; } else { throw new Exception("Configuration key '$key' does not exist."); } } // Example usage $siteTitle = getConfigValue('site_title', $config);
In this example, we have a configuration array $config, and the getConfigValue() function uses array_key_exists() to safely retrieve configuration values. If the specified key does not exist, it throws an exception, preventing potential errors in your application.
2.3. Handling API Responses
When working with APIs, you often receive data in JSON format and need to access specific fields. array_key_exists() can help you verify the presence of expected keys in the API response.
php // Assume $apiResponse contains JSON data $apiData = json_decode($apiResponse, true); if (array_key_exists('status', $apiData) && $apiData['status'] === 'success') { $responseData = $apiData['data']; // Process the response data } else { // Handle API error }
In this example, we decode a JSON API response into an associative array ($apiData) and then use array_key_exists() to check if the ‘status’ key exists and has the value ‘success’. This allows us to safely proceed with processing the response data or handle errors gracefully.
2.4. Dynamic HTML Templating
Dynamic web applications often involve rendering HTML templates with data. array_key_exists() can help ensure that the necessary template variables exist before rendering.
php // Template data $templateData = [ 'title' => 'My Blog Post', 'content' => 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.', // ... ]; // Render the template function renderTemplate($templateData) { if (array_key_exists('title', $templateData) && array_key_exists('content', $templateData)) { echo "<h1>{$templateData['title']}</h1>"; echo "<p>{$templateData['content']}</p>"; // Render additional template elements } else { throw new Exception("Required template variables are missing."); } } // Render the template renderTemplate($templateData);
In this example, the renderTemplate() function checks if the required template variables (‘title’ and ‘content’) exist in the $templateData array before rendering the HTML. This helps prevent rendering errors due to missing data.
3. Advanced Techniques
While array_key_exists() is powerful on its own, combining it with other PHP functions and techniques can unlock even more possibilities.
3.1. Using isset() for Null Values
Sometimes, you may want to check if a key exists and if it has a non-null value. In such cases, you can use isset() in combination with array_key_exists().
php $myArray = [ 'key1' => null, 'key2' => 'value', ]; if (array_key_exists('key1', $myArray) && isset($myArray['key1'])) { // Key 'key1' exists and has a non-null value } else { // Key 'key1' does not exist or has a null value }
This approach ensures that the key exists and has a value other than null.
3.2. Simplifying with the Null Coalescing Operator
In PHP 7 and later versions, you can simplify checks for array keys using the null coalescing operator (??). It checks if a key exists and assigns a default value if it doesn’t.
php $myArray = [ 'key1' => 'value1', ]; $value = $myArray['key2'] ?? 'default'; // $value will be 'default' because 'key2' does not exist in $myArray
Here, if ‘key2’ does not exist in $myArray, the null coalescing operator assigns ‘default’ as the value of $value. This can make your code more concise and readable.
Replacing array_key_exists() with array_key_exists() with array_key_exists() in PHP 8.0 and later, you can use the null-safe operator (?->) to check nested keys in multidimensional arrays. This allows for concise and safe navigation through nested data structures.
php $data = [ 'user' => [ 'name' => 'John', 'email' => 'john@example.com', ], ]; if (isset($data['user']['email'])) { // Email exists and is not null $userEmail = $data['user']['email']; } else { // Email does not exist or is null }
In PHP 8.0 and later, you can achieve the same result more concisely:
php $userEmail = $data['user']?->email ?? null;
This code checks if $data[‘user’] exists and if it does, it checks if $data[‘user’][’email’] exists and assigns the value to $userEmail. If either the user or email key is missing or null, it assigns null to $userEmail.
4. Practical Examples
To solidify your understanding of array_key_exists(), let’s explore a few practical examples showcasing its magic in real-world scenarios.
Example 1: User Authentication
Imagine you’re building a user authentication system, and you need to check if a user with a specific username exists in your database. Here’s how array_key_exists() can help:
php // Simulated user data $userDatabase = [ 'john_doe' => [ 'password' => 'hashed_password', 'email' => 'john@example.com', ], // Other user records ]; $usernameToCheck = 'john_doe'; if (array_key_exists($usernameToCheck, $userDatabase)) { // User exists; check password and proceed with authentication $userData = $userDatabase[$usernameToCheck]; // ... } else { // User does not exist; display an error message echo "User '$usernameToCheck' does not exist."; }
In this example, we use array_key_exists() to determine if the provided username ($usernameToCheck) exists in the user database. If it does, we can then proceed with password validation and authentication.
Example 2: Product Inventory
Suppose you’re managing an e-commerce website with a vast product inventory. To ensure product details are available before displaying them, you can use array_key_exists():
php // Product data $productData = [ 'product_id' => 123, 'name' => 'Smartphone', 'price' => 499.99, // ... ]; $requiredFields = ['product_id', 'name', 'price', 'description']; foreach ($requiredFields as $field) { if (!array_key_exists($field, $productData)) { // Required field is missing; handle the error die("Product information is incomplete. Missing '$field'."); } } // Display product details echo "Product Name: {$productData['name']}"; echo "Price: {$productData['price']}"; // …
In this scenario, we iterate through an array of required fields ($requiredFields) and use array_key_exists() to ensure that each field exists in the product data before displaying it. This ensures that incomplete or incorrect product information is not displayed to users.
Example 3: Internationalization (i18n) Support
For applications that support multiple languages, you may have translation arrays containing language-specific strings. array_key_exists() can help ensure that you’re using valid keys for translations:
php // English translation $translationsEN = [ 'welcome' => 'Welcome to our website!', 'contact' => 'Contact us', // ... ]; // French translation $translationsFR = [ 'welcome' => 'Bienvenue sur notre site web !', 'contact' => 'Contactez-nous', // ... ]; $language = 'fr'; // User's selected language // Function to get a translated string function translate($key, $language) { global $translationsEN, $translationsFR; $translations = []; switch ($language) { case 'en': $translations = $translationsEN; break; case 'fr': $translations = $translationsFR; break; // Add more languages as needed } if (array_key_exists($key, $translations)) { return $translations[$key]; } else { // Key not found; return a default message or handle the error return "Translation not available for key '$key'"; } } // Example usage $translatedText = translate('welcome', $language);
In this example, we have translation arrays for English and French ($translationsEN and $translationsFR). The translate() function uses array_key_exists() to check if the requested translation key exists in the selected language’s translation array. If the key is not found, it returns a default message or handles the error accordingly.
Conclusion
PHP’s array_key_exists() function is indeed a magical tool for checking the existence of keys within arrays. Its simplicity and versatility make it a valuable asset in various PHP development scenarios, from validating user input to handling complex data structures. By incorporating array_key_exists() into your coding arsenal and exploring advanced techniques, you can enhance the reliability and robustness of your PHP applications. Embrace the magic of array_key_exists() and watch your PHP code come to life with confidence and precision.
Table of Contents
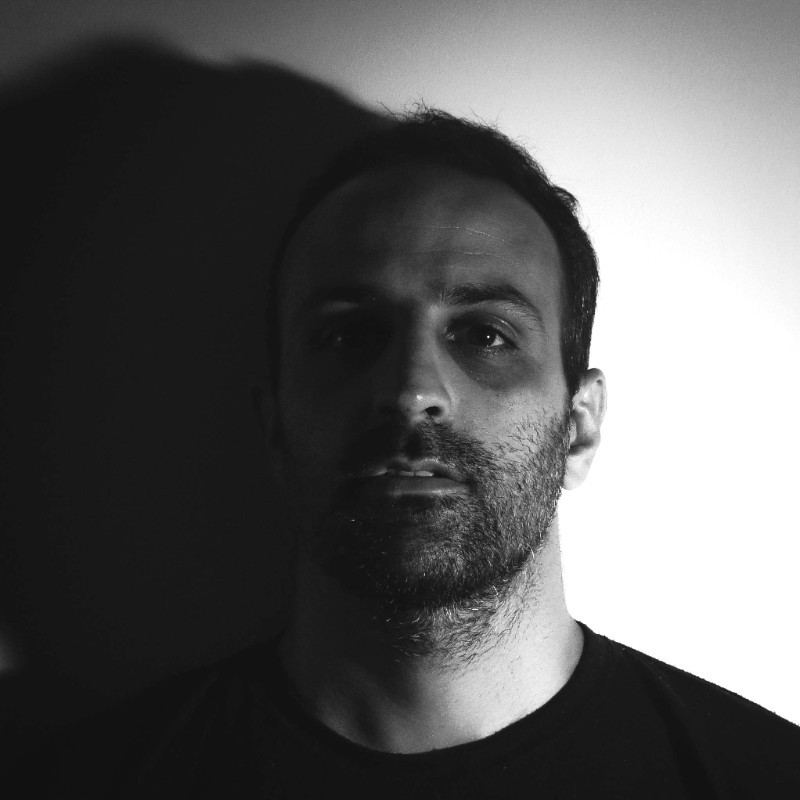
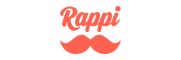