PHP’s array_map() Function: The Full Guide
In the world of PHP, arrays are fundamental data structures that allow developers to store and manage collections of data efficiently. Often, though, developers need to apply a transformation or perform an operation on every element of an array. This is where the array_map() function comes into play. In this guide, we will delve deep into the world of array_map(), exploring its syntax, use cases, and real-world examples to help you harness its capabilities effectively.
1. Understanding array_map()
array_map() is a versatile and powerful PHP function that allows you to apply a specified callback function to every element in one or more arrays, returning a new array with the transformed values. Its syntax is as follows:
php array_map(callback_function, array1, array2, ...)
- callback_function: This is the function that will be applied to each element of the input arrays. It can be a built-in function, a user-defined function, or an anonymous function.
- array1, array2, …: The input arrays that you want to apply the callback function to.
2. Applying Transformations
One of the primary use cases of array_map() is to transform the elements of an array. Let’s consider a scenario where you have an array of numbers and you want to square each number:
php $numbers = [2, 4, 6, 8, 10]; $squaredNumbers = array_map(function($num) { return $num * $num; }, $numbers); print_r($squaredNumbers);
In this example, the anonymous function squares each number in the input array, resulting in a new array containing the squared values. The output will be: [4, 16, 36, 64, 100].
3. Handling Multiple Arrays
array_map() can also work with multiple arrays simultaneously. Consider a scenario where you have two arrays representing product names and their corresponding prices. You want to combine these two arrays into a new associative array where the product name is the key and the price is the value:
php $products = ['Apple', 'Banana', 'Cherry']; $prices = [0.5, 0.3, 0.8]; $productsWithPrices = array_map(null, $products, $prices); print_r($productsWithPrices);
In this case, the null value is used as the callback function, which effectively pairs elements from the input arrays. The resulting array will be:
csharp Array ( [0] => Array ( [0] => Apple [1] => 0.5 ) [1] => Array ( [0] => Banana [1] => 0.3 ) [2] => Array ( [0] => Cherry [1] => 0.8 ) )
4. Handling More Complex Transformations
array_map() is not limited to simple transformations; it can handle more complex operations as well. Let’s say you have an array of strings representing names in the format “First Last,” and you want to convert them to the format “Last, First”:
php $names = ['John Doe', 'Jane Smith', 'Michael Johnson']; $transformedNames = array_map(function($name) { $parts = explode(' ', $name); return $parts[1] . ', ' . $parts[0]; }, $names); print_r($transformedNames);
In this example, the callback function splits each name into its constituent parts, rearranges them, and returns the transformed name. The output will be:
csharp Array ( [0] => Doe, John [1] => Smith, Jane [2] => Johnson, Michael ) Filtering Arrays
array_map() can also be used to filter arrays by applying a callback function that returns either true or false. If the callback function returns true for an element, that element is included in the resulting array; otherwise, it’s excluded.
Let’s say you have an array of numbers and you want to filter out the even numbers:
php $numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; $oddNumbers = array_map(function($num) { return $num % 2 != 0; }, $numbers); print_r($oddNumbers);
In this case, the callback function checks if each number is odd. The resulting array will only contain the odd numbers: [1, 3, 5, 7, 9].
5. Combining with Other Functions
array_map() can be used in combination with other array functions to perform more complex operations. For example, you can use array_map() to preprocess an array and then apply a reduction operation using array_reduce().
Consider a scenario where you have an array of temperatures in Celsius and you want to convert them to Fahrenheit and find the average temperature:
php $celsiusTemperatures = [20, 25, 30, 15, 22]; $fahrenheitTemperatures = array_map(function($celsius) { return ($celsius * 9/5) + 32; }, $celsiusTemperatures); $totalFahrenheit = array_reduce($fahrenheitTemperatures, function($carry, $temp) { return $carry + $temp; }); $averageFahrenheit = $totalFahrenheit / count($fahrenheitTemperatures); echo "Average Temperature in Fahrenheit: " . $averageFahrenheit . "°F";
Here, array_map() is used to convert Celsius temperatures to Fahrenheit, and array_reduce() calculates the sum of Fahrenheit temperatures. The average temperature is then computed and displayed.
Conclusion
The array_map() function in PHP opens up a world of possibilities when it comes to transforming and manipulating arrays. Its ability to apply a callback function to each element of one or more arrays simplifies many common tasks and allows developers to write concise and expressive code. Whether you’re performing simple transformations or complex operations, array_map() is a valuable tool to have in your PHP arsenal. By mastering this function, you can make your array manipulation tasks more efficient and elegant. Happy coding!
Table of Contents
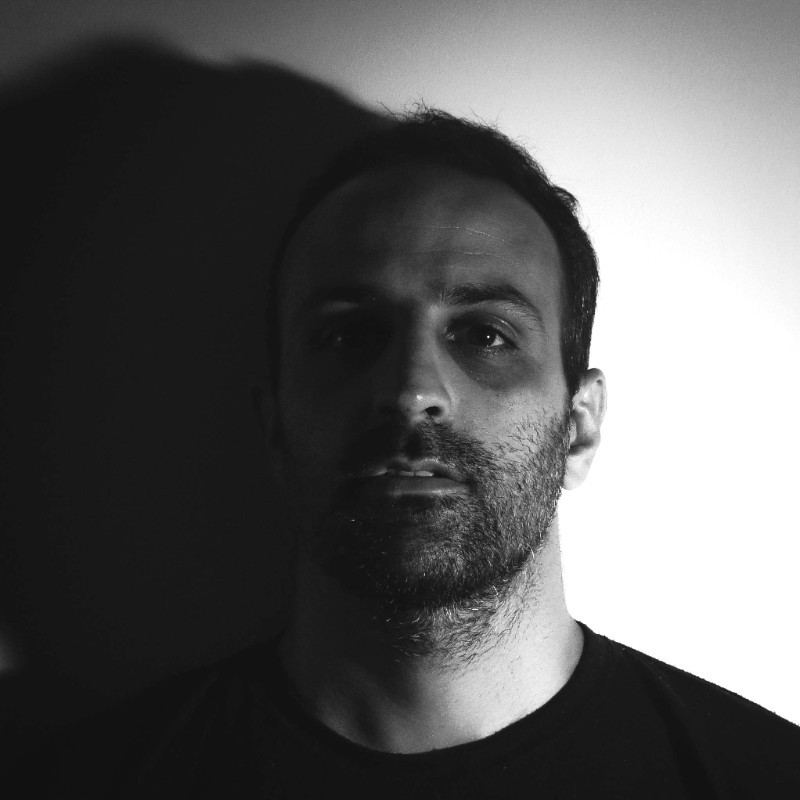
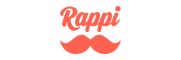