PHP’s array_multisort(): The Ultimate Sorting Tool
When it comes to sorting arrays in PHP, the array_multisort() function stands out as a powerful and versatile tool. It allows you to sort multidimensional arrays based on one or more columns, making it a valuable asset for developers dealing with complex data structures. In this blog, we will dive deep into the world of array_multisort(), exploring its syntax, use cases, and performance, and providing you with code samples to demonstrate its functionality.
Table of Contents
1. Understanding the Basics
Before we get into the details, let’s start with the basics of array_multisort(). At its core, this function is designed to sort one or more arrays or multi-dimensional arrays based on the values in one or more columns. It can handle both indexed and associative arrays, making it incredibly flexible for various use cases.
1.1. Syntax
The syntax of array_multisort() is relatively straightforward:
php array_multisort(array1, sorting_order1, sorting_type1, array2, sorting_order2, sorting_type2, ...);
- array1, array2, etc.: The arrays you want to sort.
- sorting_order1, sorting_order2, etc.: The sorting order for each array, where SORT_ASC represents ascending order, and SORT_DESC represents descending order.
- sorting_type1, sorting_type2, etc.: The sorting type for each array, which can be SORT_REGULAR, SORT_NUMERIC, SORT_STRING, SORT_LOCALE_STRING, or SORT_NATURAL.
1.2. Sorting Multiple Arrays
One of the standout features of array_multisort() is its ability to sort multiple arrays simultaneously while maintaining their relative associations. This means that if you have two or more arrays with corresponding elements, you can sort them based on a shared key without losing the relationships between elements.
2. Practical Use Cases
Now that we have a grasp of the basics, let’s explore some practical use cases for array_multisort().
2.1. Sorting a Simple Array
To start, let’s consider a straightforward example of sorting a simple indexed array:
php $fruits = array("apple", "banana", "cherry", "date"); array_multisort($fruits); print_r($fruits);
In this case, we’re sorting the $fruits array in ascending order by its values. The resulting output will be:
csharp Array ( [0] => apple [1] => banana [2] => cherry [3] => date )
2.2. Sorting Associative Arrays
array_multisort() shines even more when dealing with associative arrays. Consider a scenario where you have an array of products with prices and quantities, and you want to sort them by price:
php $products = array( array("name" => "Product A", "price" => 20.50, "quantity" => 10), array("name" => "Product B", "price" => 15.75, "quantity" => 5), array("name" => "Product C", "price" => 10.00, "quantity" => 8) ); // Sorting by price in descending order array_multisort(array_column($products, 'price'), SORT_DESC, $products); print_r($products);
Here, we’re using array_column() to extract the “price” column for sorting. The resulting output will be:
css Array ( [0] => Array ( [name] => Product A [price] => 20.5 [quantity] => 10 ) [1] => Array ( [name] => Product B [price] => 15.75 [quantity] => 5 ) [2] => Array ( [name] => Product C [price] => 10 [quantity] => 8 ) )
2.3. Sorting Multiple Arrays with Shared Keys
One of the most compelling use cases of array_multisort() is sorting multiple arrays by a shared key while maintaining their associations. Let’s say you have two arrays, one containing student names and another containing their corresponding scores, and you want to sort them by scores in descending order:
php $names = array("Alice", "Bob", "Charlie", "David"); $scores = array(90, 75, 88, 95); // Sorting by scores in descending order array_multisort($scores, SORT_DESC, $names); print_r($names); print_r($scores);
The resulting output will be:
csharp Array ( [0] => David [1] => Alice [2] => Charlie [3] => Bob ) Array ( [0] => 95 [1] => 90 [2] => 88 [3] => 75 )
As you can see, the array_multisort() function has sorted both arrays based on the scores while preserving the correspondence between names and scores.
3. Sorting with Multiple Criteria
In many real-world scenarios, you may need to sort arrays based on multiple criteria. For instance, you might want to sort a list of products first by category and then by price. This is where array_multisort() truly shines, as it allows you to specify multiple columns for sorting.
php $products = array( array("name" => "Product A", "category" => "Electronics", "price" => 200), array("name" => "Product B", "category" => "Clothing", "price" => 75), array("name" => "Product C", "category" => "Electronics", "price" => 150), array("name" => "Product D", "category" => "Clothing", "price" => 100) ); // Sorting by category in ascending order, then by price in ascending order array_multisort(array_column($products, 'category'), SORT_ASC, array_column($products, 'price'), SORT_ASC, $products); print_r($products);
The resulting output will be:
css Array ( [0] => Array ( [name] => Product C [category] => Electronics [price] => 150 ) [1] => Array ( [name] => Product A [category] => Electronics [price] => 200 ) [2] => Array ( [name] => Product D [category] => Clothing [price] => 100 ) [3] => Array ( [name] => Product B [category] => Clothing [price] => 75 ) )
In this example, we first sort the products by category in ascending order and then by price in ascending order, resulting in a sorted array based on multiple criteria.
4. Performance Considerations
While array_multisort() is a powerful tool, it’s essential to be mindful of its performance, especially when dealing with large arrays. Sorting large arrays can be resource-intensive, so here are some tips to optimize your code:
4.1. Indexing Key Columns
When sorting by a specific column, consider indexing that column using array_column() before calling array_multisort(). This can significantly improve performance by reducing the amount of data that needs to be processed during sorting.
4.2. Limit Sorting to What’s Necessary
Avoid sorting unnecessary columns. Only sort the columns that are relevant to your current task. Sorting all columns, especially in large multidimensional arrays, can be computationally expensive.
4.3. Use the Right Sorting Type
Choose the appropriate sorting type (SORT_REGULAR, SORT_NUMERIC, SORT_STRING, etc.) for your data. Using the correct type can lead to more efficient sorting.
4.4. Consider Alternative Sorting Methods
For extremely large datasets, it may be beneficial to explore alternative sorting methods like database sorting or using specialized sorting algorithms if applicable.
Conclusion
In this exploration of PHP’s array_multisort() function, we’ve seen how it can be the ultimate sorting tool for a wide range of scenarios. Whether you need to sort simple arrays or complex multidimensional arrays, array_multisort() provides the flexibility and power you need.
By understanding its syntax, practical applications, and performance considerations, you can leverage this function to efficiently organize your data. So, the next time you find yourself in need of sorting arrays in PHP, remember that array_multisort() is your go-to solution for tackling even the most challenging sorting tasks.
Table of Contents
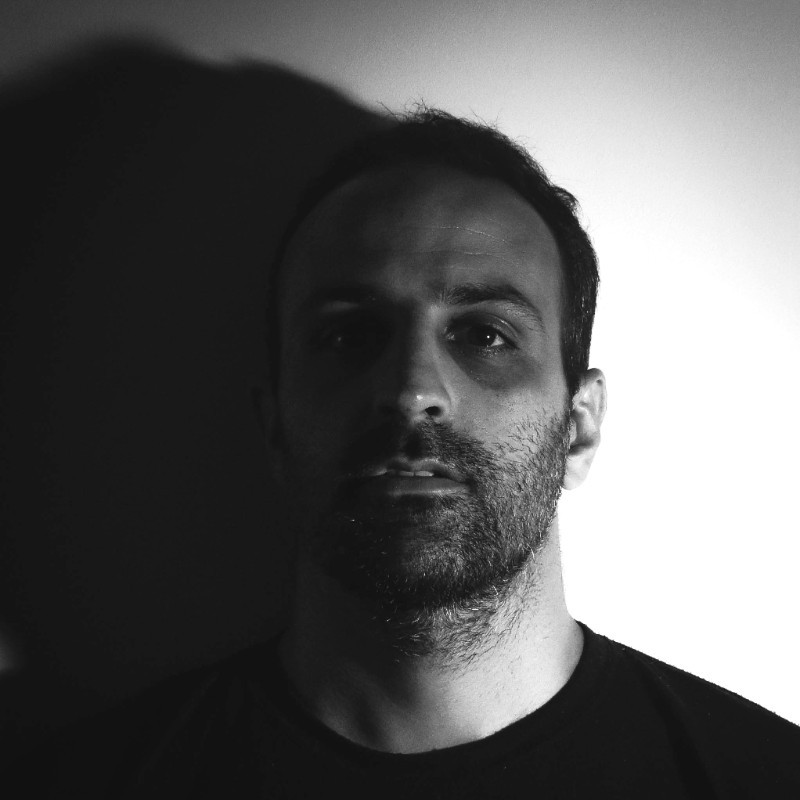
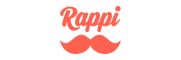