PHP Arrays: A Deep Dive
Arrays are among the most widely-used data structures in PHP, primarily due to their versatility and ease of use. They are fundamental tools that every PHP developer must master, which is why when you hire PHP developers, their proficiency in handling arrays is a critical consideration.
From storing simple lists to handling complex data structures, arrays offer endless possibilities and are a vital part of any PHP application. They are key components that make PHP a powerful and flexible language, the reason many businesses choose to hire PHP developers.
This article aims to provide a detailed understanding of PHP arrays, starting from the basics and then proceeding to more advanced topics, such as multidimensional arrays, array functions, and array manipulation. This knowledge is not only vital for those looking to hire PHP developers but also for those aiming to become competent PHP developers themselves.
If you’re looking to deepen your understanding and unlock the full potential of arrays in PHP, you’ve come to the right place. Mastering arrays will not only enable you to create more efficient code but also make you a more desirable candidate for businesses looking to hire PHP developers.
What is an Array?
In PHP, an array is a data structure that allows you to store multiple values in a single variable. This flexibility and adaptability make PHP arrays a crucial skill set for proficient PHP developers. Each value in the array can be accessed using a unique identifier known as a ‘key’. These keys can be either integer or string type, adding to the incredible flexibility and adaptability of PHP arrays to various scenarios.
Understanding these concepts is a crucial aspect for those who aspire to be hired as PHP developers. Companies looking to hire PHP developers often seek candidates with a strong grasp of such fundamental PHP constructs. So, whether you’re a budding PHP developer looking to enhance your skills or an employer aiming to hire PHP developers, understanding the intricacies of PHP arrays is a significant factor for success.
Types of Arrays
PHP provides three types of arrays: Indexed arrays, Associative arrays, and Multidimensional arrays.
Indexed Arrays
Indexed arrays have numeric keys, which are automatically assigned, starting from zero. Here is an example of an indexed array:
```php $fruits = array("Apple", "Banana", "Cherry"); echo $fruits[0]; // Outputs "Apple"
Associative Arrays
In associative arrays, the keys are custom strings, unlike the automatic numeric keys in indexed arrays. This means you can assign meaningful keys to values, making associative arrays perfect for storing things like database rows.
```php $user = array("name" => "John", "age" => 32, "city" => "London"); echo $user['name']; // Outputs "John"
Multidimensional Arrays
Multidimensional arrays are essentially arrays within arrays, useful for storing complex and structured data.
```php $users = array( "john" => array("age" => 32, "city" => "London"), "mary" => array("age" => 28, "city" => "Paris"), ); echo $users['mary']['city']; // Outputs "Paris"
Array Functions
PHP comes with a variety of built-in functions to perform operations on arrays. Let’s explore some of them.
array_push()
`array_push()` is used to add one or more elements to the end of an array.
```php $fruits = array("Apple", "Banana"); array_push($fruits, "Cherry", "Dragonfruit"); print_r($fruits); // Outputs Array ( [0] => Apple [1] => Banana [2] => Cherry [3] => Dragonfruit )
array_pop()
The `array_pop()` function removes the last element of an array and returns it.
```php $fruits = array("Apple", "Banana", "Cherry"); $lastFruit = array_pop($fruits); echo $lastFruit; // Outputs "Cherry"
array_shift() and array_unshift()
`array_shift()` removes the first element of an array and returns it, while `array_unshift()` adds one or more elements to the beginning of an array.
```php $fruits = array("Apple", "Banana", "Cherry"); $firstFruit = array_shift($fruits); echo $firstFruit; // Outputs "Apple" array_unshift($fruits, "Elderberry", "Fig"); print_r($fruits); // Outputs Array ( [0] => Elderberry [1] => Fig [2] => Banana [3] => Cherry )
sort(), rsort(), asort(), arsort(), k
sort(), krsort()
These six functions are used for sorting arrays in different orders. `sort()` and `rsort()` sort arrays in ascending and descending order, respectively. `asort()` and `arsort()` sort associative arrays in ascending and descending order, based on the values. `ksort()` and `krsort()` do the same but based on the keys.
```php $numbers = array(5, 3, 9, 1, 4); sort($numbers); print_r($numbers); // Outputs Array ( [0] => 1 [1] => 3 [2] => 4 [3] => 5 [4] => 9 )
Advanced Array Manipulation
In addition to the basics, PHP provides advanced techniques to manipulate arrays.
Filtering Arrays
You can filter the values in an array using `array_filter()`. This function traverses the array and keeps the values for which a user-provided callback function returns `true`.
```php $numbers = array(1, 2, 3, 4, 5, 6); $evenNumbers = array_filter($numbers, function($n) { return $n % 2 == 0; }); print_r($evenNumbers
Mapping Arrays
<br />`array_map()` applies a callback function to all values in the array.); // Outputs Array ( [1] => 2 [3] => 4 [5] => 6 )
```php $numbers = array(1, 2, 3, 4, 5); $squares = array_map(function($n) { return $n ** 2; }, $numbers); print_r($squares); // Outputs Array ( [0] => 1 [1] => 4 [2] => 9 [3] => 16 [4] => 25 )
Reducing Arrays
`array_reduce()` reduces an array to a single value by applying a callback function.
```php $numbers = array(1, 2, 3, 4, 5); $sum = array_reduce($numbers, function($carry, $n) { return $carry + $n; }, 0); echo $sum; // Outputs 15
Conclusion
PHP arrays are a robust, adaptable tool in your PHP toolkit. Their understanding is fundamental for those who aspire to be PHP developers or for businesses looking to hire PHP developers. By grasping the various types of arrays and learning to use PHP’s array functions, you can write cleaner, more efficient code, a highly desirable skill when you seek to hire PHP developers.
It’s also worth noting that PHP is continually evolving, with new array-related features and functions regularly added to the language. This constant evolution means that those looking to hire PHP developers should seek individuals with a commitment to lifelong learning. Similarly, those who wish to be hired as PHP developers must keep up-to-date with the latest developments as an essential part of mastering PHP arrays. Keep learning and coding!
Table of Contents
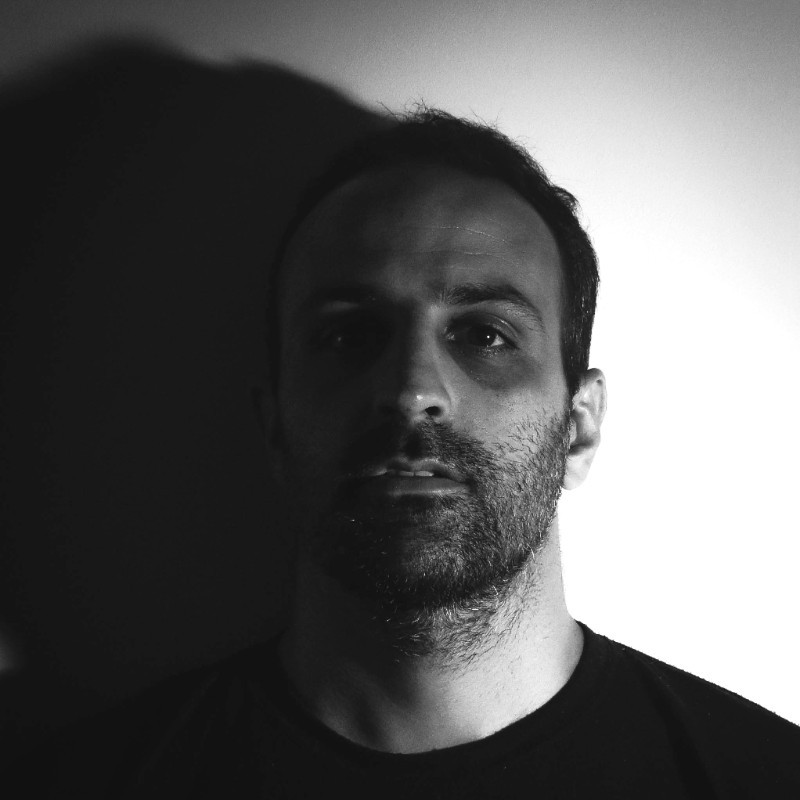
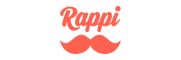