PHP: Building a Login System from Scratch
The ability for users to register and log into your website is an essential feature for many web applications. This tutorial will walk you through creating a simple, yet secure, login system using PHP and MySQL from scratch. If the process seems complicated or time-consuming, remember that there are professionals out there who specialize in this. You can hire PHP developers to handle this aspect of your website, ensuring a high level of expertise and efficiency.
This tutorial assumes you have a basic understanding of PHP, MySQL, and HTML. It is meant as a learning guide to understanding the nitty-gritty of creating a login system, which can be extremely beneficial if you’re planning to work closely with PHP developers or if you aspire to become one yourself. Also, remember that security is a serious concern in development, so this system should be used as a learning guide. In a production environment, it’s advised to hire PHP developers who are familiar with advanced security measures and frameworks to ensure robustness and security.
Let’s get started with our hands-on guide!
Database Setup
First, we need to set up a MySQL database. In a real-world scenario, this would be hosted on a server. However, for our purposes, a local installation (using a platform like XAMPP, WAMP, or MAMP) will suffice. Setting up and managing databases can sometimes be a complex task, especially for larger applications. This is where you might want to hire PHP developers. They come equipped with the necessary skills to efficiently handle databases, setup, management, and related tasks, simplifying the process and ensuring a seamless user experience.
Open your MySQL admin page (usually accessible through `localhost/phpmyadmin`) and create a new database. Let’s call it `login_system`. In this database, create a new table `users`. Our `users` table will have four columns:
- `id`: A unique ID for each user (Primary key, Auto increment).
- `username`: The username chosen by the user.
- `email`: The user’s email address.
- `password`: The user’s hashed password (NEVER store plain text passwords!).
Your SQL statement would look like this:
```sql CREATE TABLE `users` ( `id` int(11) NOT NULL AUTO_INCREMENT, `username` varchar(50) NOT NULL, `email` varchar(50) NOT NULL, `password` varchar(255) NOT NULL, PRIMARY KEY (`id`) );
Registration Form
To let users register, we need a form. Here is a basic form in HTML:
```html <form method="POST" action="register.php"> <input type="text" name="username" placeholder="Username" required> <input type="email" name="email" placeholder="Email" required> <input type="password" name="password" placeholder="Password" required> <input type="submit" value="Register"> </form>
When the form is submitted, the data is sent to `register.php` using a POST request.
Registration Processing (`register.php`)
In `register.php`, we process the data and store it in the database. Before storing, we hash the password using PHP’s `password_hash()` function. Here’s what the PHP code might look like:
```php <?php // Database connection require 'db_connect.php'; // Check if form is submitted if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $email = $_POST["email"]; $password = $_POST["password"]; // Hash the password $hashed_password = password_hash($password, PASSWORD_DEFAULT); // SQL query to insert the data into database $sql = "INSERT INTO users (username, email, password) VALUES (?, ?, ?)"; $stmt = $conn->prepare($sql); $stmt->bind_param("sss", $username, $email, $hashed_password); // Execute the statement if ($stmt->execute()) { echo "New record created successfully"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } $stmt->close(); $conn->close(); } ?>
This file also uses `db_connect.php`, which should include the necessary information to connect to your database:
```php <?php $servername = "localhost"; $username = "root"; $password = ""; $dbname = "login_system"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } ?>
Login Form
Now that users can register, we need a login form:
```html <form method="POST" action="login.php"> <input type="text" name="username" placeholder="Username" required> <input type="password" name="password" placeholder="Password" required> <input type="submit" value="Login"> </form>
Similar to the registration form, when this form is submitted, the data is sent to `login.php` via a POST request.
Login Processing (`login.php`)
In `login.php`, we need to check the provided username and password against the database. If a match is found, we log the user in; if not, we return an error. Here’s how that might look:
```php <?php // Database connection require 'db_connect.php'; // Check if form is submitted if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $password = $_POST["password"]; // Get user with the provided username $sql = "SELECT * FROM users WHERE username=?"; $stmt = $conn->prepare($sql); $stmt->bind_param("s", $username); $stmt->execute(); $result = $stmt->get_result(); $user = $result->fetch_assoc(); // Verify password if ($user && password_verify($password, $user['password'])) { // Successful login session_start(); $_SESSION["loggedin"] = true; $_SESSION["username"] = $username; echo "You are now logged in"; } else { // Authentication failed echo "Invalid username or password"; } $stmt->close(); $conn->close(); } ?>
Upon successful login, we start a session and store some information in the session variable. This will let us keep the user logged in as they navigate our site.
Logout
To log out, we just need to destroy the session:
```php <?php session_start(); session_destroy(); header("Location: login.php"); exit; ?>
This will log the user out and redirect them back to the login page.
Conclusion
And there you have it! A basic login system from scratch in PHP. Remember, this is a simplified version, and in a real-world application, you would want to handle additional things like form validation, email verification, password reset functionality, and more advanced security measures. In such scenarios, you might find it beneficial to hire PHP developers. These experts can efficiently handle the complexities of advanced features and security measures, leaving you with a robust and secure system.
You might also want to use prepared statements or an ORM to prevent SQL injection attacks, another area where the expertise of professional PHP developers can prove invaluable. They have the necessary knowledge and experience to protect your site against various potential threats, enhancing the security and reliability of your application. Happy coding, and remember, when in need, don’t hesitate to hire PHP developers to ensure a seamless, secure digital experience for your users!
Table of Contents
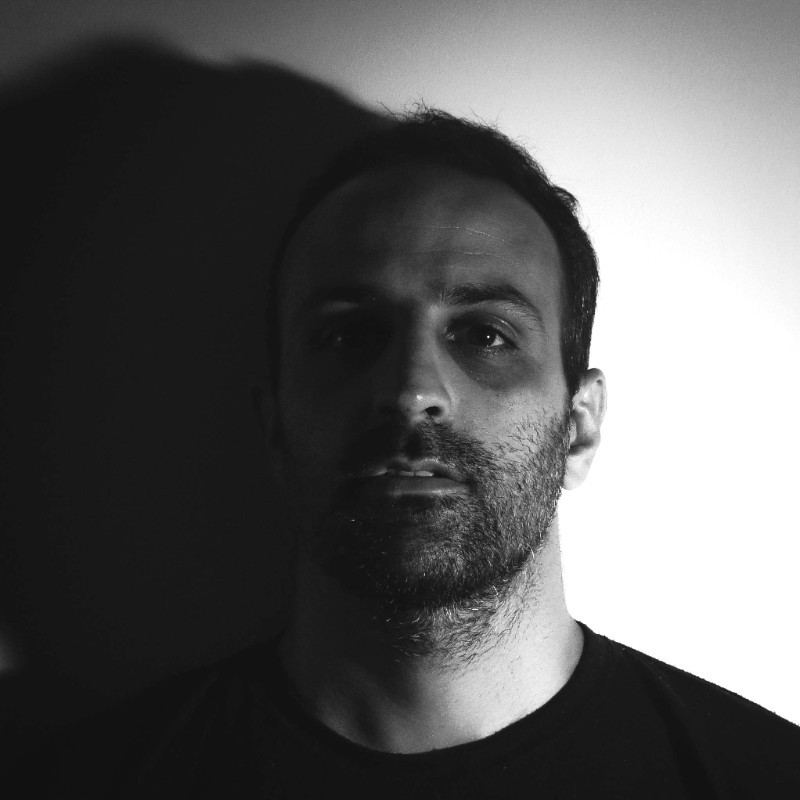
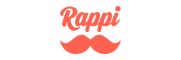