A Guide to PHP’s chr() Function: Working with ASCII
ASCII (American Standard Code for Information Interchange) is a character encoding standard that assigns a unique numerical value to each character on a keyboard, including letters, numbers, and special symbols. In PHP, the chr() function plays a crucial role in dealing with ASCII codes. Whether you’re building a text-based game, manipulating strings, or working on low-level data processing, understanding how to use chr() effectively is essential.
Table of Contents
1. Understanding ASCII Codes
Before diving into the details of the chr() function, let’s grasp the basics of ASCII codes. ASCII assigns a numerical value (ranging from 0 to 127) to each character, making it easier to represent and manipulate text in digital systems. These codes are used to store and transmit information in a machine-readable format.
1.1. ASCII Code Structure
- Decimal Representation: Each character in ASCII has a decimal representation, ranging from 0 to 127. For example, the decimal code for the letter ‘A’ is 65, while ‘a’ is represented as 97.
- Hexadecimal Representation: ASCII codes can also be expressed in hexadecimal format, which is often used in programming. The letter ‘A’ in hexadecimal is represented as 0x41, and ‘a’ as 0x61.
- Binary Representation: At its core, ASCII is a binary encoding system. Each character has a unique 7-bit binary code. For instance, ‘A’ is represented as 01000001 in binary.
1.2. Common ASCII Codes
Here are some examples of commonly used ASCII codes:
- Newline: ASCII code 10 represents the newline character, which is used to start a new line in text.
- Space: ASCII code 32 represents the space character, which is used to create spaces between words and characters.
- Digits: ASCII codes 48 to 57 represent the digits 0 to 9.
- Uppercase Letters: ASCII codes 65 to 90 represent uppercase letters from ‘A’ to ‘Z’.
- Lowercase Letters: ASCII codes 97 to 122 represent lowercase letters from ‘a’ to ‘z’.
- Special Characters: ASCII includes various special characters like punctuation marks, symbols, and control characters, each with its unique code.
Now that we have a basic understanding of ASCII, let’s explore how the chr() function in PHP can help us work with these codes effectively.
3. The PHP chr() Function
The chr() function in PHP is used to convert an ASCII code (an integer between 0 and 127) into its corresponding character. This function takes an integer as its argument and returns a single-character string.
3.1. Syntax of chr()
php chr(int $asciiCode): string
$asciiCode: The integer ASCII code to be converted.
3.2. Example Usage
Let’s start with some simple examples to demonstrate how the chr() function works:
Example 1: Convert ASCII code to character
php $asciiCode = 65; // ASCII code for 'A' $character = chr($asciiCode); echo $character; // Output: A
In this example, we use the chr() function to convert the ASCII code 65 to its corresponding character, which is ‘A’. The result is then echoed to the screen.
Example 2: Convert multiple ASCII codes to characters
php $asciiCodes = [72, 101, 108, 108, 111]; // ASCII codes for 'Hello' $characters = array_map('chr', $asciiCodes); echo implode('', $characters); // Output: Hello
Here, we convert an array of ASCII codes into characters and then concatenate them to form the word ‘Hello’.
4. Practical Applications of chr()
The chr() function can be incredibly useful in various scenarios, such as:
4.1. Text Manipulation
You can use chr() to generate characters dynamically and manipulate text. For example, you could create a function that generates a random string of characters for password generation:
php function generateRandomPassword($length) { $password = ''; for ($i = 0; $i < $length; $i++) { $randomAscii = rand(97, 122); // Generate a random lowercase letter $password .= chr($randomAscii); } return $password; } $password = generateRandomPassword(8); echo $password; // Output: Example: "qazwsxed"
In this example, we generate a random lowercase letter by generating a random ASCII code between 97 (‘a’) and 122 (‘z’) and then converting it to a character using chr().
4.2. File Handling
Working with binary files often involves dealing with ASCII codes to read or write specific characters. The chr() function simplifies this process:
php $file = fopen('binary_file.bin', 'wb'); $asciiCode = 65; // ASCII code for 'A' $character = chr($asciiCode); fwrite($file, $character); fclose($file);
Here, we open a binary file in write mode, convert the ASCII code for ‘A’ to a character, and write it to the file.
4.3. Encoding Conversions
In some cases, you might need to perform encoding conversions, especially when working with internationalization and character sets. The chr() function can be a part of such conversions when used in conjunction with other PHP functions.
5. Handling Extended ASCII Codes
While the standard ASCII table covers codes from 0 to 127, there are also extended ASCII codes that go beyond this range. These codes are used to represent characters from various languages and special symbols. To work with extended ASCII codes, you need to be aware of a few considerations.
5.1. Extended ASCII Range
Extended ASCII codes range from 128 to 255, giving you access to additional characters not available in the standard ASCII set. For example, these codes can represent characters with diacritics used in languages like Spanish, French, and German.
5.1.1. Converting Extended ASCII Codes
To convert extended ASCII codes to characters using the chr() function, simply pass the desired code as an argument. For example:
php $extendedAsciiCode = 150; // Extended ASCII code for a special character $character = chr($extendedAsciiCode); echo $character; // Output: a character represented by code 150
However, it’s essential to consider the character encoding used in your PHP environment. PHP internally uses UTF-8 as its default encoding, which supports extended ASCII characters as well as characters from various Unicode blocks. When working with extended ASCII or other non-ASCII characters, ensure that your script’s encoding is set appropriately to handle them correctly.
5.2. UTF-8 Encoding
UTF-8 is a variable-length encoding that can represent characters from various scripts and languages, making it a suitable choice for handling extended ASCII characters and beyond. When working with UTF-8-encoded strings, you can still use the chr() function to convert ASCII codes, but it’s essential to handle multibyte characters properly.
For example, if you want to convert the extended ASCII code 150 (or any other code) within a UTF-8 context, you can do so like this:
php $extendedAsciiCode = 150; // Extended ASCII code for a special character $character = chr($extendedAsciiCode); $utf8Character = mb_convert_encoding($character, 'UTF-8', 'ISO-8859-1'); echo $utf8Character;
In this example, we first convert the extended ASCII character to a UTF-8 character using the mb_convert_encoding() function. The second argument specifies the target encoding (‘UTF-8’), and the third argument indicates the source encoding (‘ISO-8859-1’, which is a common encoding for extended ASCII characters).
6. Handling Non-Printable ASCII Codes
ASCII includes several control characters that are non-printable, meaning they don’t have a visible representation when displayed as text. Instead, these control characters have specific functions, such as controlling cursor movement or indicating the end of a line. Examples of non-printable ASCII codes include the newline character (ASCII 10) and the tab character (ASCII 9).
When working with non-printable ASCII codes, it’s essential to understand their purpose and how to use them effectively.
6.1. Common Non-Printable ASCII Codes
Here are some common non-printable ASCII codes and their functions:
- ASCII 10 (Newline): Used to start a new line in text or move the cursor to the next line. It’s commonly used to separate lines of text in documents.
- ASCII 9 (Tab): Represents the tab character, which is used to create horizontal indentation or spacing between text.
- ASCII 13 (Carriage Return): Used in combination with the newline character (ASCII 10) to represent the end of a line. In some systems, a carriage return is used before a newline character to move the cursor to the beginning of the current line.
- ASCII 8 (Backspace): Represents the backspace control character, which moves the cursor one position backward without erasing any characters. This character is often used in text editors and terminals.
- ASCII 7 (Bell): Produces an audible or visible alert, typically used to get the user’s attention.
6.2. Working with Non-Printable ASCII Codes
To work with non-printable ASCII codes, you can use the chr() function just like you would with printable characters. Here are a few examples:
Example 1: Inserting a Newline Character
php $text = "Line 1" . chr(10) . "Line 2"; echo $text;
In this example, we use chr(10) to insert a newline character between “Line 1” and “Line 2,” resulting in two separate lines of text when displayed.
Example 2: Creating Tabs
php $text = "Item 1" . chr(9) . "Price: $10" . chr(10) . "Item 2" . chr(9) . "Price: $15"; echo $text;
Here, we use chr(9) to create tabs between item names and prices, aligning them neatly.
Example 3: Producing a Bell Sound
php echo "Processing complete." . chr(7);
In this example, we append chr(7) to the text to produce a bell sound or visual alert after the message.
6.3. Escaping Control Characters
Sometimes, you might need to display control characters as text rather than having them perform their control functions. To do this, you can escape the control characters by using their corresponding escape sequences. Here are some common escape sequences for non-printable ASCII control characters:
- \n: Represents the newline character (ASCII 10).
- \t: Represents the tab character (ASCII 9).
- \r: Represents the carriage return character (ASCII 13).
- \b: Represents the backspace character (ASCII 8).
For example, if you want to display the newline character as text, you can use the escape sequence \n:
php $text = "Line 1\nLine 2"; echo $text;
The output will display the text with a visible newline character between “Line 1” and “Line 2.”
7. Tips for Efficient ASCII Manipulation
Working with ASCII codes effectively requires some best practices and tips to ensure your code is efficient and reliable.
7.1. Error Handling
Always validate and handle user input when working with ASCII codes, especially when converting user-provided input to characters. Ensure that the input falls within the valid ASCII code range (0 to 127) and handle any potential errors or exceptions gracefully.
7.2. Character Encoding
Understand the character encoding used in your PHP environment, especially when working with extended ASCII characters or multibyte characters. Set the appropriate encoding when converting between ASCII codes and characters.
7.3. Performance Considerations
While the chr() function is straightforward and easy to use, it’s essential to consider performance when dealing with a large number of conversions. In such cases, avoid unnecessary conversions and use efficient data structures and algorithms.
7.4. Escape Sequences
When displaying control characters as text, use escape sequences for clarity. This helps other developers understand your code and prevents unexpected behavior.
7.5. Documentation
If your code deals extensively with ASCII codes and characters, consider documenting the purpose and usage of specific codes and characters. This can be especially helpful for maintaining and understanding complex systems.
Conclusion
Understanding and working with ASCII codes is a fundamental skill for PHP developers. The chr() function simplifies the process of converting ASCII codes to characters, making it easier to manipulate text, handle control characters, and work with extended ASCII characters when needed.
By mastering the use of the chr() function and following best practices for efficient ASCII manipulation, you can confidently work with text-based data, create dynamic content, and handle various character encoding scenarios in your PHP applications. Whether you’re building a simple text processing tool or a complex internationalization system, knowing how to work with ASCII is a valuable skill that can enhance your programming capabilities.
Table of Contents
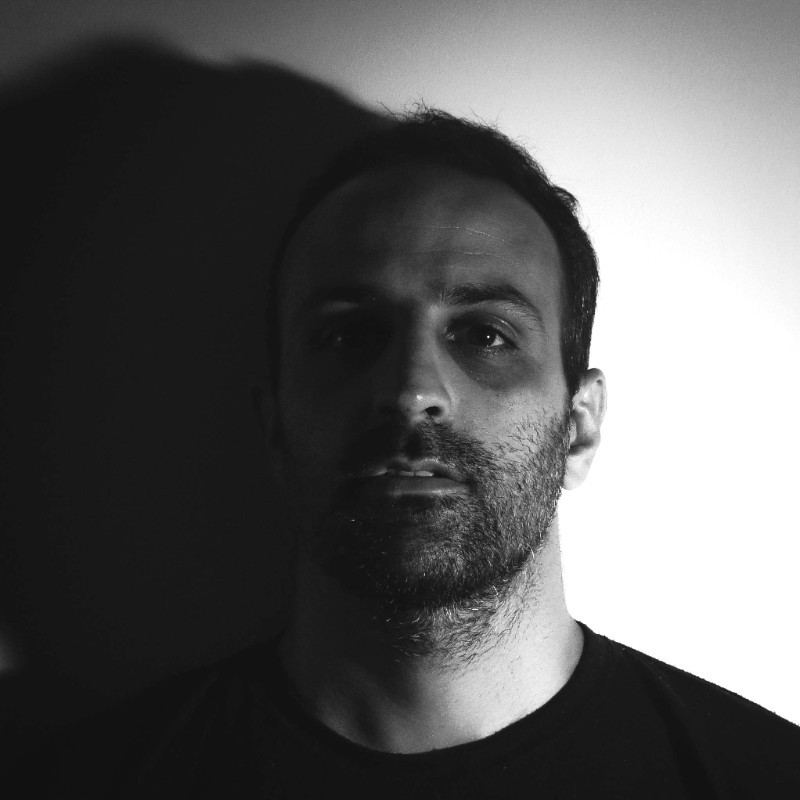
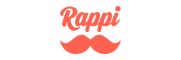